Select statement application method of Golang function
Golang, as an efficient and flexible programming language, has attracted the attention of more and more developers. One of the important features, the select statement, has won the favor of many developers. This article will introduce the application method of select statement in Golang function to help readers gain an in-depth understanding of the use and advantages of this statement.
- The definition and basic usage of the select statement
The select statement is one of the keywords to control the flow in Golang. It allows the program to wait for multiple communication operations. Any one of these operations is ready and the corresponding action is performed. If multiple communication operations can be performed, select randomly selects one of them for execution. The following is the basic structure of the select statement:
select { case communicationClause1: //执行语句 case communicationClause2: //执行语句 … default: //执行语句 }
Among them, communicationClause can be a channel operation, sending or receiving operation. When select is executed, it will traverse each case. If there is a case that can perform communication operations, then the code in that case will be executed. If multiple cases can be executed, any one of them will be randomly selected for execution. If no case can be executed, the code in the default statement block will be executed.
- Application scenarios of select statements
The select statement has many application scenarios in Golang. Some of the more common scenarios are listed below.
2.1. Waiting for multiple channel operations
Golang uses channels for concurrent programming. When we need to wait for multiple channel operations, we can use the select statement. The following example demonstrates how to wait for the output results of two goroutines and print them:
func main() { channel1 := make(chan string) channel2 := make(chan string) go func() { time.Sleep(1 * time.Second) channel1 <- "Hello" }() go func() { time.Sleep(2 * time.Second) channel2 <- "Golang" }() for i := 0; i < 2; { select { case message1 := <-channel1: fmt.Println(message1) i++ case message2 := <-channel2: fmt.Println(message2) i++ } } }
2.2. Timeout control
When doing network programming or other operations that require waiting, we often need Set a timeout to avoid waiting too long and causing the application to become unresponsive. The following is an example of using the select statement to implement timeout control:
func doSomething() error { timeout, errChan := time.After(time.Second * 5), make(chan error) go func() { errChan <- someTask() //someTask() 表示需要执行的任务 }() select { case err := <-errChan: return err //执行完成,返回结果 case <-timeout: return fmt.Errorf("timeout") //超时,返回错误信息 } }
2.3. Preventing deadlock
In channel operations, if there are not enough goroutines to receive data, deadlock will usually result. Through the select statement, you can avoid this situation and ensure the normal execution of the program. The following is an example of using a select statement to avoid deadlock:
func main() { channel := make(chan int) go func() { channel <- 10 }() select { case <-channel: fmt.Println("Receive data from channel") default: fmt.Println("No data received") } }
- Advantages of the select statement
The select statement is widely used in Golang, and its advantages are mainly reflected in The following aspects:
3.1. Can perform concurrent programming very easily, allowing developers to write concise and efficient concurrent programs.
3.2. The select statement can avoid excessive occupation of CPU resources. If you need to sleep for a period of time while waiting for certain operations, you can put the CPU into sleep state to avoid wasting system resources.
3.3. It is very suitable for use in multi-channel monitoring scenarios, and can achieve efficient event management and processing. When processing thousands of concurrent events, the performance is very superior.
- Summary
This article introduces the application method of select statement in Golang function and its advantages in concurrent programming. Through the above application scenarios and examples, I believe readers have a deeper understanding of the select statement. As an excellent programming language, Golang is excellent in concurrent programming, and the select statement has become one of the most commonly used tools in Golang. It is recommended that developers learn and use it more and develop efficient and stable concurrent applications based on actual needs.
The above is the detailed content of Select statement application method of Golang function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
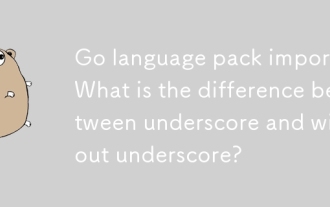
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
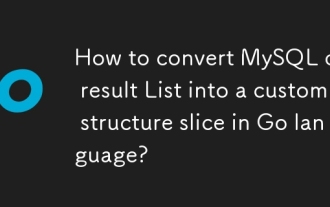
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
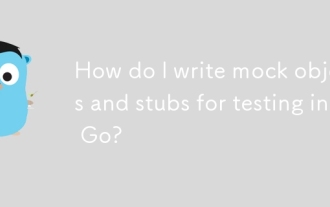
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
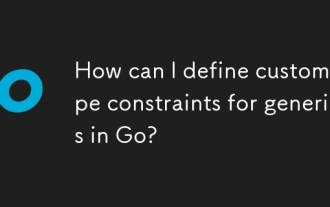
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
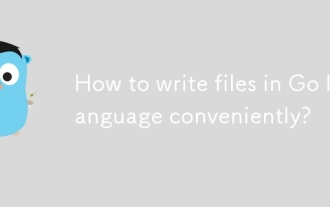
This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
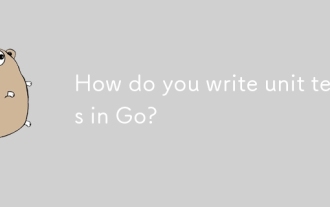
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
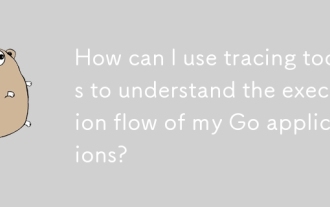
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
