golang slice reverse
Slice in Go language is very commonly used. It is a dynamic array that can be easily added, deleted and modified. But sometimes we need to reverse the slice elements, for example, reverse {1, 2, 3, 4, 5} into {5, 4, 3, 2, 1}. This article will introduce how to use the Go language to implement slicing. reverse.
- Use loops to achieve
The reversal of a slice is actually to arrange the elements of the slice in reverse order, which can be achieved using a loop. The specific method is to move the first element of the slice to the end, the second element to the second to last, and so on. It should be noted that if the length of the slice is an odd number, the middle elements do not need to be exchanged.
The following is the code implementation using a loop:
func reverse(s []int) { for i := 0; i < len(s)/2; i++ { j := len(s) - i - 1 s[i], s[j] = s[j], s[i] } }
The reverse function in the code receives a slice of type int as a parameter and reverses the slice. First use a for loop to traverse the first half of the elements of the slice and exchange them with the corresponding last elements. It should be noted that the index j is obtained by len(s)-i-1, because the index of the last element of the slice is len(s)-1, and the index of the last element corresponding to the i-th element is len(s) -i-1. When the slice length is an odd number, the middle element does not need to be exchanged.
Use this function to reverse an int type slice of any length, for example:
func main() { s1 := []int{1,2,3,4,5} reverse(s1) fmt.Println(s1) s2 := []int{2,4,6,8,10,12} reverse(s2) fmt.Println(s2) }
The output result is as follows:
[5 4 3 2 1] [12 10 8 6 4 2]
- Use the standard library function to implement
In addition to using loops, the standard library also provides a function to reverse slices-reverse. This function is defined in the sort package and is used as follows:
package main import ( "fmt" "sort" ) func main() { s1 := []int{1,2,3,4,5} sort.Slice(s1, func(i, j int) bool { return i > j }) fmt.Println(s1) s2 := []int{2,4,6,8,10,12} sort.Slice(s2, func(i, j int) bool { return i > j }) fmt.Println(s2) }
The sort.Slice function in the code receives two parameters. The first parameter is the slice to be reversed, and the second parameter is A function that defines the rules for inversion. In this function, if i is greater than j, it returns true, indicating that i and j need to be swapped forward.
It should be noted that the sort.Slice function can only operate on types that conform to the sort.Interface interface, so the element type of the slice needs to implement the Len, Less and Swap methods. For slices of type int, it has implemented these methods, so you can directly use the sort.Slice function to perform the reverse operation.
Use the sort.Slice function to reverse the slice. The code is as follows:
[5 4 3 2 1] [12 10 8 6 4 2]
Both methods can easily reverse the slice. Which method should be used? You can choose according to the actual situation. If you only need to reverse once, it is recommended to use the loop method. If you need to reverse multiple times, you can consider using the sort.Slice function, because it can reuse the sorting rules and improve efficiency.
The above is the detailed content of golang slice reverse. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


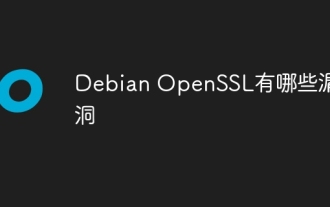
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
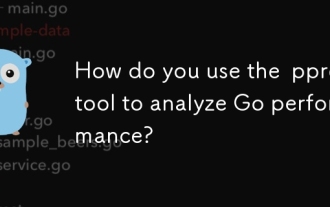
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
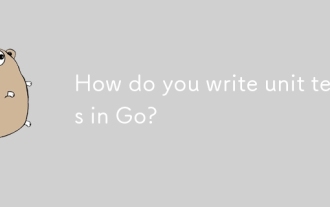
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
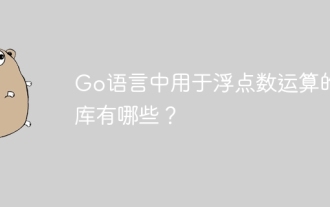
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
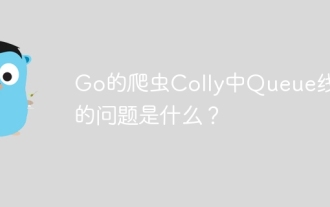
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
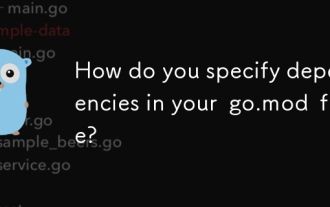
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
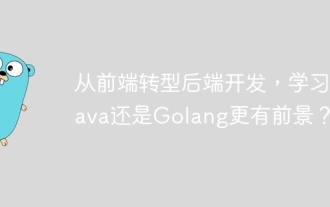
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
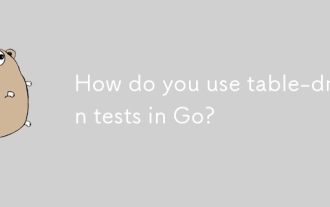
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
