


How to implement automated operation and maintenance in python
1. Install
pip install paramiko
2. Import module
import paramiko
3. Use
def initSshClinet (): ''' Initialization, SSH connection account and password to log in to the server: return: sshClinet ''' ip = ""#Server ip address sshClinet = paramiko.SSHClient() sshClinet.set_missing_host_key_policy(paramiko.AutoAddPolicy()) sshClinet.connect( ip, 22, userName, pw, timeout=360) return sshClinet
def exeCommond(commond): ''' Execute shell command''' stdin, stdout, stderr = sshClient.exec_command(command) outStr = stdout .readlines() print("\n".join(outStr))
def sftpUploadFile(localPath, remotePath): #Get the SFTP instance sftp = sshClinet.open_sftp() #Perform the upload action sftp.put(localPath , remotePath)
def sftpDownloadFile(localPath, remotePath): #Get the SFTP instance sftp = sshClinet.open_sftp() #Execute the download action sftp.get(localPath, remotePath)
Remember to close at the end Connection
sshClient.close()
You can also log in using a private key:
# Configure the private key file location private = paramiko.RSAKey.from_private_key_file('/Users/ ch/.ssh/id_rsa')#Instantiate SSHClientclient = paramiko.SSHClient()#Automatically add a policy to save the host name and key information of the server. If it is not added, hosts that are no longer recorded in the local know_hosts file will not be able to connect to the client. .set_missing_host_key_policy(paramiko.AutoAddPolicy())#Connect to the SSH server and authenticate with username and password client.connect(hostname='10.0.0.1',port=22,username='root',pkey=private)
Learn
paramiko contains two core components: SSHClient and SFTPClient.
SSHClient functions similar to the Linux ssh command. It is an encapsulation of the SSH session. This class encapsulates the transport, channel and SFTPClient establishment method (open_sftp). It is usually used to execute remote Order. The function of SFTPClient is similar to the sftp command of Linux. It is an encapsulation of the SFTP client and is used to implement remote file operations, such as file upload, download, and file permission modification.
Channel is a kind of Socket, a secure SSH transmission channel. Transport is an encrypted session. When used, an encrypted Tunnels (channel) will be created simultaneously. This Tunnels is called ChannelSession and is maintained between the client and the Server. The connected object, use connect()/start_client()/start_server() to start the session
Introduction to common methods of SSHClient
connect(): realize the connection of the remote server With authentication, only hostname is a required parameter for this method.
hostname Target host for connection port=SSH_PORT Specified port username=None Verified username password=None Verified user password pkey=None Private key method for authentication key_filename=None A file name or file list, Specify private key file timeout=None Optional tcp connection timeout allow_agent=True Whether to allow connection to ssh agent, the default is True Allow look_for_keys=True Whether to search for private key files in ~/.ssh, the default is True Allow compress=False Whether to turn on compression
set_missing_host_key_policy(): Set the response policy when the remote server is not recorded in the know_hosts file. Pass in the subclass of MissingHostKeyPolicy. Currently, three strategies are supported:
Set the strategy when the connected remote host does not have a local host key or HostKeys object. Currently, three strategies are supported:
AutoAddPolicy automatically adds Host name and host key to the local HostKeys object, does not depend on the configuration of load_system_host_key. That is, when establishing a new ssh connection, you do not need to enter yes or no for confirmation. WarningPolicy is used to record python warnings for an unknown host key. And accept, the function is similar to AutoAddPolicy, but it will prompt that new connections RejectPolicy automatically rejects unknown host names and keys, relying on the configuration of load_system_host_key. This is the default option
exec_command(): A method to execute Linux commands on a remote server.
open_sftp(): Create an sftp session based on the current ssh session. This method returns an SFTPClient object.
Introduction to common methods of SFTPClient
from_transport(cls,t): Create a connected SFTP client channel put(localpath, remotepath, callback=None, confirm= True): Upload local files to the server Parameter confirm: Whether to call the stat() method to check the file status and return the result of ls -l get(remotepath, localpath, callback=None): Download files from the server to the local mkdir(): In Create a directory on the server remove(): Delete the directory on the server rename(): Rename the directory on the server stat(): View the server file status listdir(): List the files in the server directory
The above is the detailed content of How to implement automated operation and maintenance in python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



HadiDB: A lightweight, high-level scalable Python database HadiDB (hadidb) is a lightweight database written in Python, with a high level of scalability. Install HadiDB using pip installation: pipinstallhadidb User Management Create user: createuser() method to create a new user. The authentication() method authenticates the user's identity. fromhadidb.operationimportuseruser_obj=user("admin","admin")user_obj.
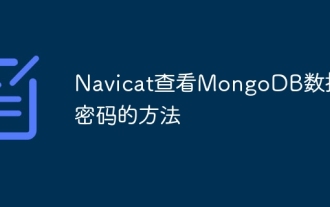
It is impossible to view MongoDB password directly through Navicat because it is stored as hash values. How to retrieve lost passwords: 1. Reset passwords; 2. Check configuration files (may contain hash values); 3. Check codes (may hardcode passwords).
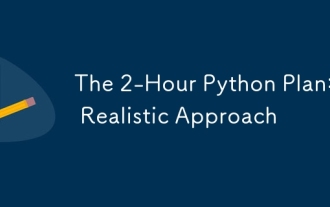
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
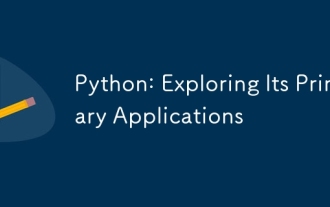
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
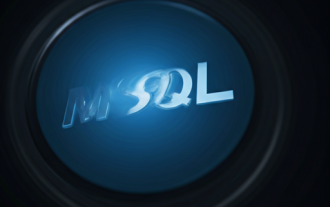
MySQL database performance optimization guide In resource-intensive applications, MySQL database plays a crucial role and is responsible for managing massive transactions. However, as the scale of application expands, database performance bottlenecks often become a constraint. This article will explore a series of effective MySQL performance optimization strategies to ensure that your application remains efficient and responsive under high loads. We will combine actual cases to explain in-depth key technologies such as indexing, query optimization, database design and caching. 1. Database architecture design and optimized database architecture is the cornerstone of MySQL performance optimization. Here are some core principles: Selecting the right data type and selecting the smallest data type that meets the needs can not only save storage space, but also improve data processing speed.
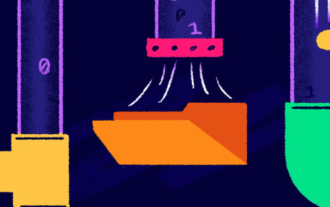
As a data professional, you need to process large amounts of data from various sources. This can pose challenges to data management and analysis. Fortunately, two AWS services can help: AWS Glue and Amazon Athena.
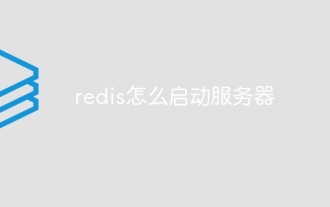
The steps to start a Redis server include: Install Redis according to the operating system. Start the Redis service via redis-server (Linux/macOS) or redis-server.exe (Windows). Use the redis-cli ping (Linux/macOS) or redis-cli.exe ping (Windows) command to check the service status. Use a Redis client, such as redis-cli, Python, or Node.js, to access the server.
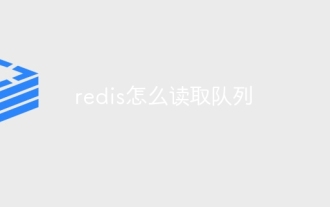
To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.
