webbrowser wtl javascript error handling
In web development, JavaScript is an essential part. With the continuous development and application of JavaScript, developers are faced with more and more JavaScript errors. These errors may lead to page crashes, functional failures, or even user information leakage. Therefore, it is very important to promptly identify and handle JavaScript errors. This article will introduce how to use the WebBrowser control, WTL, and JavaScript for JavaScript error handling.
- Introduction to WebBrowser control
The WebBrowser control is an ActiveX control in the Microsoft Windows operating system and can be used to embed web browsers. The WebBrowser control supports all web browsing functions supported by IE browser, such as HTML, CSS, JavaScript, etc.
The WebBrowser control can embed a web browser in a local Win32 application, provide a browser-style GUI interface, and allow developers to use programming languages such as C to call browser APIs to access Web content. This control allows developers to embed web pages in desktop applications such as Microsoft Word, providing a richer user interface and functionality.
- WTL Framework Introduction
WTL (Windows Template Library) is a C template library for Windows application development. It makes use of technologies such as template classes provided by ATL (Active Template Library) to make it easier for developers to write Windows applications. Because WTL uses template classes, it can reduce the burden of creating controls and processing resources. Therefore, WTL is one of the best-performing UI libraries on the Windows platform.
WTL provides a large number of macros and template classes for window control and message processing, which can reduce the amount of code for Win32 API calls. It provides a large number of helper classes and tools for creating, extending and interacting with interface controls, allowing developers to integrate the WebBrowser control in their programs in less time.
- Introduction to JavaScript Error Handling
Since JavaScript is a runtime interpreted language, errors may occur in the code. JavaScript errors are divided into syntax errors and logic errors.
Syntax errors are errors caused by the code not complying with JavaScript syntax specifications. For example, spelling errors, missing commas, mismatched brackets, etc.
Logical errors are errors caused by incorrect code meaning or incorrect execution context. For example, adding strings and numbers, using undeclared variables, etc.
For syntax errors, they can be corrected through the IDE or code editor. For logical errors, they need to be solved through code debugging or error handling. The JavaScript error handling methods are mainly divided into try-catch statements and window.onerror methods.
The try-catch statement is a commonly used error handling method. It is used to capture errors that occur in the try code block and handle them through the statements in the catch code block. For example:
try { // some code } catch(err) { console.log(err.message); }
window.onerror method is a JavaScript global error handling method that allows developers to handle JavaScript errors at runtime. For example:
window.onerror = function(message, url, lineNumber) { console.log("Error: " + message + " in " + url + " at line " + lineNumber); }
- WebBrowser control embedded in WTL application
To embed the WebBrowser control in WTL, you need to perform the following steps:
4.1 Create a WTL application Win32 Application
Use Visual Studio to create an empty Win32 application, then select the WTL Application Wizard and create a WTL application.
4.2 Add WebBrowser control in the dialog box
In the dialog box editor, add a space type of "CCustomControl", and then set its ID to "IDC_EXPLORER".
4.3 Create a class containing the WebBrowser control
class CWebBrowserView : public CWindowImpl<CWebBrowserView, CAxWindow> { public: BEGIN_MSG_MAP(CWebBrowserView) MESSAGE_HANDLER(WM_CREATE, OnCreate) END_MSG_MAP() private: LRESULT OnCreate(UINT uMsg, WPARAM wParam, LPARAM lParam, BOOL& bHandled) { HRESULT hr = CreateControl(CComBSTR("{8856F961-340A-11D0-A96B-00C04FD705A2}"), m_hWnd, NULL); return 0; } };
4.4 Add the WebBrowser control in the dialog box class
In the header file of the dialog box class, add member variables:
CWebBrowserView m_wndView;
In OnInitDialog, allocate space for the WebBrowser control:
CRect rcClient; GetClientRect(&rcClient); m_wndView.Create(m_hWnd, rcClient, NULL, WS_CHILD | WS_VISIBLE | WS_CLIPSIBLINGS | WS_CLIPCHILDREN);
- JavaScript error handling
Embedding JavaScript scripts in the WebBrowser control mainly includes the following Methods:
5.1 Directly parse JavaScript scripts
You can directly parse and execute JavaScript scripts through the methods provided by the WebBrowser control. For example:
CComPtr<IDispatch> spDocDisp; m_explorer.QueryService(IID_IDispatch, IID_IDispatch, reinterpret_cast<void**>(&spDocDisp)); CComVariant vtResult; CComBSTR bstrScript("if (confirm('Are you sure?')) { alert('OK'); }"); spDocDisp.Invoke1(L"eval", &CComVariant(bstrScript), &vtResult);
5.2 Embed JavaScript script in HTML page
You can embed JavaScript script in HTML page, for example:
<html> <body> <script type="text/javascript"> function checkForm() { var name = document.getElementById("name").value; if (name == '') { alert('Please enter your name.'); return false; } return true; } </script> <form onsubmit="return checkForm();"> <p>Name: <input type="text" id="name"></p> <p><input type="submit" value="Submit"></p> </form> </body> </html>
5.3 Load external JavaScript file
External JavaScript files can be loaded through script tags in HTML pages. For example:
<head> <script type="text/javascript" src="test.js"></script> </head>
- JavaScript Error Handling Example
Embed the WebBrowser control in a WTL application and embed a JavaScript script in an HTML page, for example:
<html> <body> <script type="text/javascript"> function test() { var name = document.getElementById("name").value; if (name == '') { throw new Error('Please enter your name.'); } } </script> <form onsubmit="test();"> <p>Name: <input type="text" id="name"></p> <p><input type="submit" value="Submit"></p> </form> </body> </html>
Add window message processing in WTL applications to obtain JavaScript error information. For example:
LRESULT OnError(UINT uMsg, WPARAM wParam, LPARAM lParam, BOOL& bHandled) { CComPtr<IServiceProvider> spSrvProvider; HRESULT hr = m_explorer.QueryInterface(IID_IServiceProvider, reinterpret_cast<void**>(&spSrvProvider)); CComPtr<IWebBrowser2> spBrowser; hr = spSrvProvider->QueryService(SID_SWebBrowserApp, IID_IWebBrowser2, reinterpret_cast<void**>(&spBrowser)); CComPtr<IDispatch> spScript; hr = spBrowser->get_Script(&spScript); CComBSTR bstrMessage; hr = spScript.GetPropertyByName(L"error.message", &CComVariant(), &bstrMessage); CComBSTR bstrUrl; hr = spScript.GetPropertyByName(L"error.url", &CComVariant(), &bstrUrl); CComVariant vtLine; hr = spScript.GetPropertyByName(L"error.lineNumber", &CComVariant(), &vtLine); ATLTRACE(_T("JavaScript error: %s in %s at line %d"), bstrMessage, bstrUrl, V_I4(&vtLine)); return 0; }
Then, register the error message handling to the DocumentComplete event of the WebBrowser control. For example:
void OnDocumentComplete(IDispatch *pDisp, VARIANT *url) { CComQIPtr<IHTMLDocument2> spDoc2(pDisp); CComQIPtr<IHTMLWindow2> spWin2; spDoc2->get_parentWindow(&spWin2); if (spWin2) { CComVariant vt; spWin2->execScript(CComBSTR("window.onerror=function() { return true; }"), CComBSTR("javascript"), &vt); spWin2->execScript(CComBSTR("window.onerror"), CComBSTR("javascript"), &vt); } }
In this way, when a JavaScript error is encountered in the WebBrowser control, the error information can be obtained and processed through the OnErro message processing function.
- Summary
This article introduces how to embed the WebBrowser control in a WTL application and introduces JavaScript error handling methods. JavaScript is an important part of web development, and timely handling of JavaScript errors is crucial to improving the robustness and security of applications. When developing using the WebBrowser control and WTL technology, developers can more easily implement JavaScript error detection and processing, providing users with more stable and secure web applications.
The above is the detailed content of webbrowser wtl javascript error handling. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
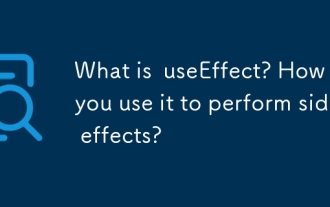
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
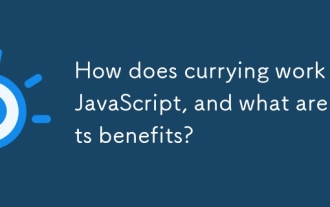
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
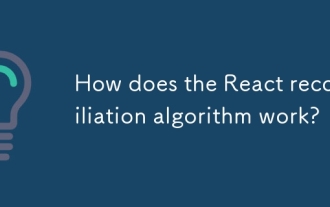
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
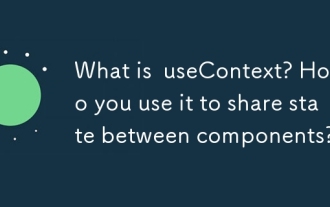
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
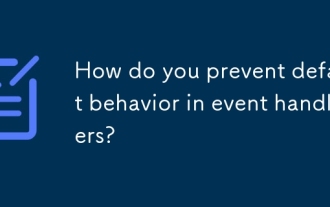
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
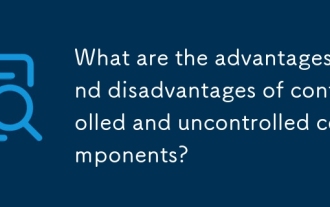
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
