JavaScript sets font style
In front-end development, it is often necessary to set the style of web fonts to meet the needs of aesthetics and reading experience. JavaScript is a commonly used front-end scripting language. By using its API, you can easily style web page fonts.
1. Set the font size
Using JavaScript to set the font size can be achieved using the style attribute and the CSS attribute font-size. The following is a sample code using the DOM API:
// 获取指定元素 var elements = document.getElementsByClassName('my-class'); // 设置字体大小 for(var i=0; i<elements.length; ++i) { elements[i].style.fontSize = '20px'; }
2. Set the font color
Similarly, you can use the CSS property color to set the font color. The sample code is as follows:
// 获取指定元素 var elements = document.getElementsByClassName('my-class'); // 设置字体颜色 for(var i=0; i<elements.length; ++i) { elements[i].style.color = 'red'; }
3. Set the font type
When using JavaScript to set the font type, you need to use the CSS property font-family. The sample code is as follows:
// 获取指定元素 var elements = document.getElementsByClassName('my-class'); // 设置字体类型 for(var i=0; i<elements.length; ++i) { elements[i].style.fontFamily = 'Arial, sans-serif'; }
4. Set the font thickness
Use the CSS property font-weight to set the font thickness. The sample code is as follows:
// 获取指定元素 var elements = document.getElementsByClassName('my-class'); // 设置字体粗细 for(var i=0; i<elements.length; ++i) { elements[i].style.fontWeight = 'bold'; }
5. Set the font style
In addition to the above properties, you can also use the CSS property font-style to set the font style, such as italics. The sample code is as follows:
// 获取指定元素 var elements = document.getElementsByClassName('my-class'); // 设置字体样式 for(var i=0; i<elements.length; ++i) { elements[i].style.fontStyle = 'italic'; }
Summary
Through the above code examples, we can see that it is very convenient to use JavaScript to set the web font style, which can be achieved by simply using the DOM API and CSS properties. When using it, you need to pay attention to browser compatibility and set appropriate font styles according to design requirements to improve the user's reading experience and aesthetics.
The above is the detailed content of JavaScript sets font style. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
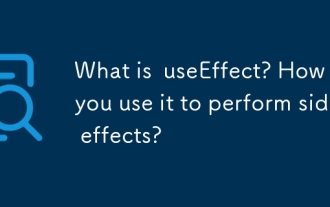
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
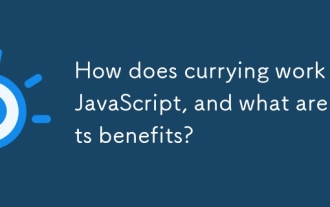
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
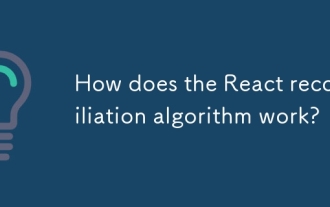
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
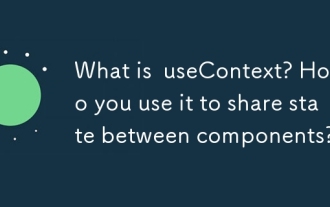
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
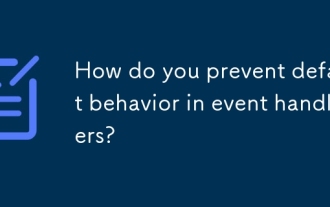
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
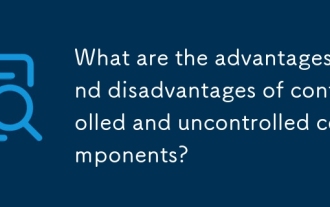
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
