How to write javascript plug-in
JavaScript is a commonly used scripting language that is widely used in web development. JavaScript plug-ins are components that add interactivity and complexity to web applications. In this article, we will learn how to write JavaScript plugins.
1. Basic knowledge
Before writing a JavaScript plug-in, we need to understand the following basic knowledge:
- DOM operation: The main function of the JavaScript plug-in is to operate on the DOM Operation, so we need to have a certain understanding of DOM operations.
- Events: JavaScript plug-ins usually need to interact with users and process user input, so we need to understand various event types and how to handle them.
- Browser compatibility: Different browsers have different levels of support for JavaScript. We need to read through various documents to understand how to deal with browser compatibility issues.
2. Plug-in structure
The main components of JavaScript plug-in include HTML, CSS and JavaScript code.
- HTML: Plug-ins usually need to display some content on the page, so we need to use HTML to define the structure of the plug-in.
- CSS: Plug-ins require certain styles to beautify their appearance, so we need to use CSS to define the style of the plug-in.
- JavaScript: The main function of the plug-in is to use JavaScript to operate DOM elements. We need to write JavaScript code to implement the functionality of the plug-in.
3. Implementation of plug-in
The following are the steps to implement a simple JavaScript plug-in.
- Define plug-in structure: Use HTML to define the structure of the plug-in.
<div class="plugin"> <h3>插件标题</h3> <p>插件内容</p> </div>
- Define plugin style: Use CSS to define the style of the plugin.
.plugin { border: 1px solid #ccc; padding: 10px; } .plugin h3 { font-size: 16px; font-weight: bold; } .plugin p { font-size: 14px; line-height: 1.5; }
- Implement plug-in functions: Use JavaScript to implement plug-in functions.
// 获取插件元素 var pluginEle = document.querySelector('.plugin'); // 处理插件事件 pluginEle.addEventListener('click', function() { alert('插件被点击了!'); });
4. Optimization of plug-ins
In order to make JavaScript plug-ins more reliable, easy to maintain and expand, we need to optimize them.
- Encapsulate code: Encapsulate JavaScript code into functions to make it easier to reuse and modify.
(function() { // ...插件代码... })();
- Use namespaces: Use namespaces to avoid conflicting with variable names in other plugins or JavaScript libraries.
var MyPlugin = { // ...插件代码... };
- Avoid global variables: Try to avoid using global variables when writing plug-ins.
- Support options: Add options to the plug-in, allowing users to customize the functionality and appearance of the plug-in.
- Support event mechanism: use custom events to implement plug-in interaction and message delivery.
6. Conclusion
JavaScript plug-in is a very useful web development component. When writing JavaScript plug-ins, you need to understand the basic knowledge, define the plug-in structure, implement plug-in functions, and optimize the plug-in. Writing high-quality plug-ins is an important skill for experienced developers.
The above is the detailed content of How to write javascript plug-in. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


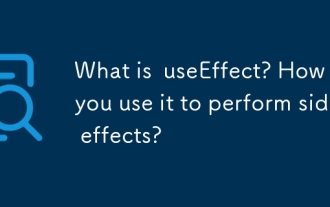
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
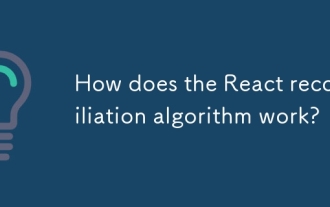
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
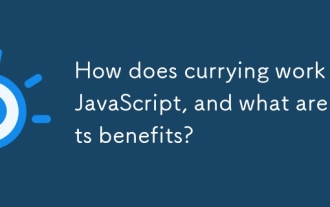
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
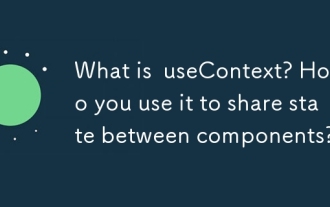
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
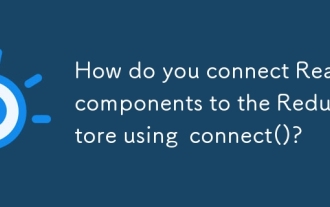
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.
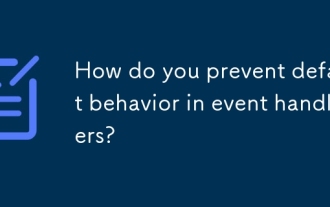
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
