


What is the method of encapsulating axios and using mock.js with vue3 and ts?
Preface
We should pay attention to distinguishing between Axios and Ajax:
Ajax is a general term for technology, and the technical content includes: HTML or XHTML , CSS, JavaScript, DOM, XML, XSLT, and the most important XMLHttpRequest, which is used to use asynchronous data transmission (HTTP request) between the browser and the server to make local requests to achieve local refresh. The use is based on XMLHttpRequest;
Axios is a promise-based HTTP library and a third-party library
Main technology stack: vue3, ts, axios, mock.js, elementPlus
1. Dependency installation and processing of axios
1. Dependency installation
Using asynchronous network requests is definitely inseparable from loading, message and other prompts. Today we will use it with elementPlus;
// 安装axios npm install axios --save // 安装 elementPlus npm install element-plus --save
2. Global axios package
In the utils directory under the src directory, create a new request.ts. Because TS is used, the data format needs to be defined in advance:
Define the format of request data return, which needs to be confirmed in advance
Define axios basic configuration information
Request interceptor: The place where all requests arrive first. We can customize the request header information here (such as token, multi-language, etc.)
Response interceptor: return the place where the data first arrives. , we can handle exception information here (for example: code 401 redirects to login, code 500 prompts error message)
import axios, { AxiosInstance, AxiosError, AxiosRequestConfig, AxiosResponse } from "axios"; import { ElMessage, ElLoading, ElMessageBox } from "element-plus"; // response interface { code, msg, success } // 不含 data interface Result { code: number, success: boolean, msg: string } // request interface,包含 data interface ResultData<T = any> extends Result { data?: T } enum RequestEnums { TIMEOUT = 10000, // 请求超时 request timeout FAIL = 500, // 服务器异常 server error LOGINTIMEOUT = 401, // 登录超时 login timeout SUCCESS = 200, // 请求成功 request successfully } // axios 基础配置 const config = { // 默认地址,可以使用 process Node内置的,项目根目录下新建 .env.development baseURL: process.env.VUE_APP_BASE_API as string, timeout: RequestEnums.TIMEOUT as number, // 请求超时时间 withCredentials: true, // 跨越的时候允许携带凭证 } class Request { service: AxiosInstance; constructor(config: AxiosRequestConfig) { // 实例化 serice this.service = axios.create(config); /** * 请求拦截器 * request -> { 请求拦截器 } -> server */ this.service.interceptors.request.use( (config: AxiosRequestConfig) => { const token = localStorage.getItem('token') ?? ''; return { ...config, headers: { 'customToken': "customBearer " + token } } }, (error: AxiosError) => { // 请求报错 Promise.reject(error) } ); /** * 响应拦截器 * response -> { 响应拦截器 } -> client */ this.service.interceptors.response.use( (response: AxiosResponse) => { const { data, config } = response; if (data.code === RequestEnums.LOGINTIMEOUT) { // 表示登录过期,需要重定向至登录页面 ElMessageBox.alert("Session expired", "System info", { confirmButtonText: 'Relogin', type: 'warning' }).then(() => { // 或者调用 logout 方法去处理 localStorage.setItem('token', ''); location.href = '/' }) } if (data.code && data.code !== RequestEnums.SUCCESS) { ElMessage.error(data); return Promise.reject(data); } return data }, (error: AxiosError) => { const { response } = error; if (response) { this.handleCode(response.status); } if (!window.navigator.onLine) { ElMessage.error("网络连接失败,请检查网络"); // 可以重定向至404页面 } } ) } public handleCode = (code: number): void => { switch (code) { case 401: ElMessage.error("登陆失败,请重新登录"); break; case 500: ElMessage.error("请求异常,请联系管理员"); break; default: ElMessage.error('请求失败'); break; } } // 通用方法封装 get<T>(url: string, params?: object): Promise<ResultData<T>> { return this.service.get(url, { params }); } post<T>(url: string, params?: object): Promise<ResultData<T>> { return this.service.post(url, params); } put<T>(url: string, params?: object): Promise<ResultData<T>> { return this.service.put(url, params); } delete<T>(url: string, params?: object): Promise<ResultData<T>> { return this.service.delete(url, { params }); } } export default new Request(config)
3. Actual use
Add api/index.ts in the src directory
Define the parameter type of the request
Define the specific parameter type of the response
Here we use the namespace in ts. In actual development, many of our APIs may have the same name with different meanings, so we use namespace for definition
import request from "@/utils/request"; namespace User { // login export interface LoginForm { userName: string, password: string } } export namespace System { export interface Info { path: string, routeName: string } export interface ResponseItem { code: number, items: Array<Sidebar>, success: boolean } export interface Sidebar { id: number, hashId: string | number, title: string, routeName: string, children: Array<SidebarItem>, } export interface SidebarItem { id: number, parentId: number, hashId: string | number, title: string, } } export const info = (params: System.Info) => { // response if (!params || !params.path) throw new Error('Params and params in path can not empty!') // 这里因为是全局的一个info,根据路由地址去请求侧边栏,所需不用把地址写死 return request.post<System.Sidebar>(params.path, { routeName: params.routeName }) }
Call in Vue file
<script lang="ts" setup name="Sidebar"> import { ref, reactive, onBeforeMount } from "vue" import { info } from "@/api" import { useRoute } from "vue-router" const route = useRoute(); let loading = ref<boolean>(false); let sidebar = ref<any>({}); const _fetch = async (): Promise<void> => { const routeName = route.name as string; const path = '/' + routeName.replace(routeName[0], routeName[0].toLocaleLowerCase()) + 'Info' try { loading.value = true; const res = await info({ path, routeName }); if (!res || !res.data) return; sidebar.value = res.data; } finally { loading.value = false } } onBeforeMount(() => { _fetch(); }) </script>
2. Dependency installation and processing of mock.js
1. Install dependencies
# 安装 npm install mockjs --save
When used in ts , we need to throw the module in the shims-vue.d.ts file now, otherwise there will be problems with introducing errors
/* eslint-disable */ declare module '*.vue' { import type { DefineComponent } from 'vue' const component: DefineComponent<{}, {}, any> export default component } declare module 'mockjs';
2. Create the files required for the new mock
index.ts (belongs to the mockjs global configuration file), mockjs/javaScript/index.ts (specific data files), these two need to be paid attention to, and others do not need to be paid attention to
1. Create a new mockjs /javaScript/index.ts (specific data file)
Because the data here is mainly the data in the sidebar, which are all fixed, so the rules of mockjs are not used to generate data.
import { GlobalSidebar, Sidebar } from "../../sidebar"; namespace InfoSidebar { export type InfoSidebarParams = { body: string, type: string, url: string } } const dataSource: Array<GlobalSidebar> = [ { mainTitle: 'JavaScript基础问题梳理', mainSidebar: [ { id: 0, hashId: 'This', title: 'this指向', routeName: 'JsBasic', children: [ { id: 1, parentId: 0, hashId: 'GlobalFunction', title: '全局函数' }, { id: 2, parentId: 0, hashId: 'ObjectMethod', title: '对象方法' }, { id: 3, parentId: 0, hashId: 'Constructor', title: '构造函数' }, { id: 4, parentId: 0, hashId: 'SetTimeout', title: '定时器、回调函数' }, { id: 5, parentId: 0, hashId: 'EventFunction', title: '事件函数' }, { id: 6, parentId: 0, hashId: 'ArrowFunction', title: '箭头函数' }, { id: 7, parentId: 0, hashId: 'CallApplyBind', title: 'call、apply、bind' }, ] }, { id: 2, hashId: 'DeepClone', title: '深拷贝和浅拷贝', routeName: 'JsBasic', children: [] } ] }, ]; export default { name: 'jsBasicInfo', jsBasicInfo(params: InfoSidebar.InfoSidebarParams) { const param = JSON.parse(params.body) if (!param) throw new Error("Params can not empty!"); const data = dataSource.find((t: GlobalSidebar) => { return t.mainSidebar.filter((x: Sidebar) => { return x.routeName === param.routeName }) }) return { data, success: true, code: 200 } } }
Sidebar.ts
/** * @param { number } id Unique value * @param { string } hashId href Unique value * @param { string } title show current title * @param { string } routeName page find data */ interface GlobalSidebar { mainTitle: string, mainSidebar: Array<Sidebar> } interface Sidebar { id: number, hashId: string | number, title: string, routeName: string, children: Array<SidebarItem>, } interface SidebarItem { id: number, parentId: number, hashId: string | number, title: string, } export { GlobalSidebar, Sidebar, SidebarItem }
2. Create a new mockjs/index.ts
import Mock from "mockjs"; import jsBasicInfo from "./tpl/javaScript/index"; const requestMethod = 'post'; const BASE_URL = process.env.VUE_APP_BASE_API; const mocks = [jsBasicInfo]; for (let i of mocks) { Mock.mock(BASE_URL + '/' + i.name, requestMethod, i.jsBasicInfo); } export default Mock
3. Introduce main.ts
import { createApp } from 'vue' import App from './App.vue' if(process.env.NODE_ENV == 'development'){ require('./mockjs/index') } const app = createApp(App); app.mount('#app');
3. Combined use
is actually the code that just called axios
<script lang="ts" setup name="Sidebar"> import { ref, reactive, onBeforeMount } from "vue" import { info } from "@/api" import { useRoute } from "vue-router" const route = useRoute(); let loading = ref<boolean>(false); let sidebar = ref<any>({}); const _fetch = async (): Promise<void> => { const routeName = route.name as string; const path = '/' + routeName.replace(routeName[0], routeName[0].toLocaleLowerCase()) + 'Info' try { loading.value = true; const res = await info({ path, routeName }); if (!res || !res.data) return; sidebar.value = res.data; } finally { loading.value = false } } onBeforeMount(() => { _fetch(); }) </script>
The above is the detailed content of What is the method of encapsulating axios and using mock.js with vue3 and ts?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
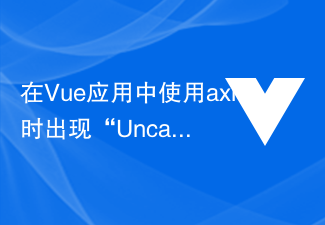
It is very common to use axios in Vue applications. axios is a Promise-based HTTP client that can be used in browsers and Node.js. During the development process, the error message "Uncaught(inpromise)Error: Requestfailedwithstatuscode500" sometimes appears. For developers, this error message may be difficult to understand and solve. This article will explore this
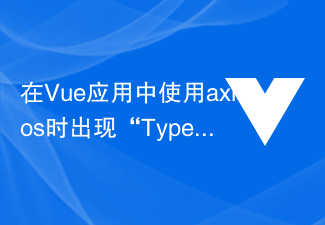
Recently, during the development of Vue applications, I encountered a common problem: "TypeError: Failedtofetch" error message. This problem occurs when using axios to make HTTP requests and the backend server does not respond to the request correctly. This error message usually indicates that the request cannot reach the server, possibly due to network reasons or the server not responding. What should we do after this error message appears? Here are some workarounds: Check your network connection due to
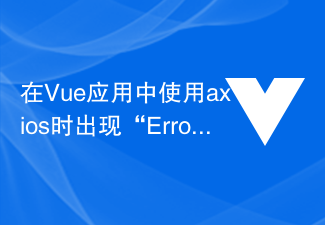
How to solve the problem of "Error: NetworkError" when using axios in Vue application? In the development of Vue applications, we often use axios to make API requests or obtain data, but sometimes we encounter "Error: NetworkError" in axios requests. What should we do in this case? First of all, you need to understand what "Error:NetworkError" means. It usually means that the network connection
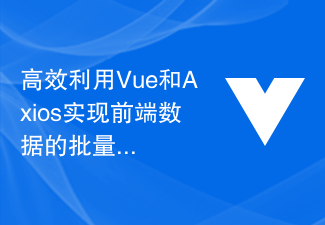
Efficiently utilize Vue and Axios to implement batch processing of front-end data. In front-end development, data processing is a common task. When we need to process a large amount of data, processing the data will become very cumbersome and inefficient if there is no effective method. Vue is an excellent front-end framework, and Axios is a popular network request library. They can work together to implement batch processing of front-end data. This article will introduce in detail how to efficiently use Vue and Axios for batch processing of data, and provide relevant code examples.
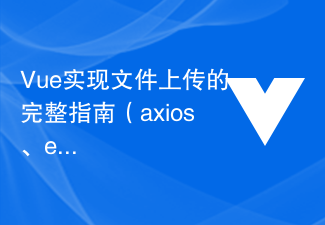
A complete guide to implementing file upload in Vue (axios, element-ui) In modern web applications, file upload has become a basic function. Whether uploading avatars, pictures, documents or videos, we need a reliable way to upload files from the user's computer to the server. This article will provide you with a detailed guide on how to use Vue, axios and element-ui to implement file upload. What is axiosaxios is a prom based
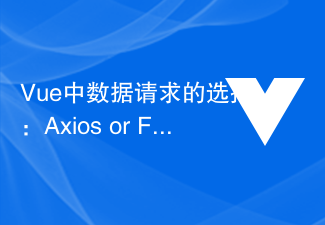
Choice of data request in Vue: AxiosorFetch? In Vue development, handling data requests is a very common task. Choosing which tool to use for data requests is a question that needs to be considered. In Vue, the two most common tools are Axios and Fetch. This article will compare the pros and cons of both tools and give some sample code to help you make your choice. Axios is a Promise-based HTTP client that works in browsers and Node.
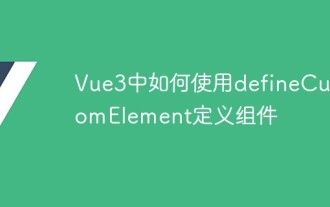
Using Vue to build custom elements WebComponents is a collective name for a set of web native APIs that allow developers to create reusable custom elements (customelements). The main benefit of custom elements is that they can be used with any framework, even without one. They are ideal when you are targeting end users who may be using a different front-end technology stack, or when you want to decouple the final application from the implementation details of the components it uses. Vue and WebComponents are complementary technologies, and Vue provides excellent support for using and creating custom elements. You can integrate custom elements into existing Vue applications, or use Vue to build
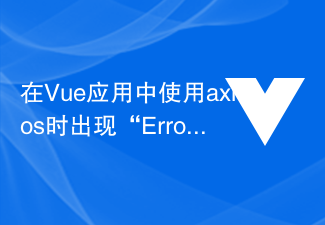
What should I do if "Error: timeoutofxxxmsexceeded" occurs when using axios in a Vue application? With the rapid development of the Internet, front-end technology is constantly updated and iterated. As an excellent front-end framework, Vue has been welcomed by everyone in recent years. In Vue applications, we often need to use axios to make network requests, but sometimes the error "Error: timeoutofxxxmsexceeded" occurs.
