How to implement real long connection in nodejs
With the rapid development of Internet technology, more and more applications need to implement real-time update or data transmission functions. In the traditional HTTP protocol, when the client requests data from the server, the server disconnects after completing the response. Continuous data transmission cannot be achieved, so it cannot meet real-time requirements. In order to solve this problem, two technologies, WebSocket and long connection, have emerged. This article mainly introduces how to use Node.js to implement real long connection technology.
1. What is long connection technology
In the traditional HTTP protocol, the client requests data from the server, and the server will disconnect after completing the response. Although this short-lived connection method saves server resources, it also brings some problems. For example, the client needs to frequently request data from the server, resulting in a waste of network bandwidth; at the same time, in applications with high real-time requirements, the client needs to keep sending requests, but the server also needs to keep processing the requests. , which will lead to a waste of server resources.
Long connection technology is a technology that maintains the connection status between the client and the server, and can realize real-time communication between the client and the server. In the long connection technology, after the client requests data from the server, the server does not return the data immediately. Instead, it maintains the connection state and waits for data updates on the server before returning. This method can reduce the number of frequent requests, reduce network bandwidth consumption, and also reduce the load on the server.
2. How to implement long-term connection in Node.js
- Use socket.io
socket.io is the most popular long-term connection in Node.js One of the solutions for connectivity. Socket.io is an open source real-time communication library that can establish a continuous connection between the client and the server to achieve two-way communication. It supports multiple protocols such as WebSocket, HTML5 Server-Sent Events (SSE) and Long Polling, so socket.io can provide the optimal real-time communication method under different browsers or operating environments.
First, you need to install the socket.io module in the Node.js environment:
npm install socket.io
In the server code, introduce the socket.io module and listen for client connections:
const app = require('http').createServer(handler) const io = require('socket.io')(app) app.listen(3000) io.on('connection', (socket) => { console.log('a user connected') })
In the client code, establish a connection through the socket.io library:
const socket = io.connect('http://localhost:3000')
In this example, when the client successfully establishes a connection, 'a user connected' will be output on the console.
After the connection is established, two-way communication can be achieved. For example, the server can send a message to the client:
io.emit('message', { content: 'Hello World!' })
and the client can receive the message:
socket.on('message', (data) => { console.log(data.content) })
- Use HTTP long connection
Use HTTP long connections are also a way to implement long connections. In the HTTP protocol, there is no need to disconnect between the client and the server, and the connection can be maintained continuously. Long connections can be achieved by periodically sending blank requests or using semi-closed technology.
In Node.js, you can use the keep-alive feature of the HTTP module to implement HTTP long connections. keep-alive is a mechanism to maintain the state of a TCP connection. In an HTTP long connection, the server will add the following code to the HTTP header before returning a response:
Connection: keep-alive Keep-Alive: timeout=5, max=1000
This means that the server requires the client to maintain the connection for 5 seconds, and can maintain a maximum of 1,000 connections.
The client can use the keepAlive
attribute of the http.Agent
class to open a long connection:
const http = require('http') const agent = new http.Agent({ keepAlive: true }) http.get({ hostname: 'localhost', port: 8080, path: '/', agent: agent }, (res) => { res.setEncoding('utf8') res.on('data', (chunk) => { console.log(chunk) }) })
In the above example, the client opens Long connection and sent an HTTP GET request. When the server has data updates, update information will be automatically sent.
3. Applicable Scenarios of Long Connections
Long connection technology can be used in many scenarios, such as online games, social chat, real-time page updates and other applications that require real-time performance. However, long connections are not omnipotent and have certain flaws and shortcomings.
First of all, long connections will occupy server resources because the connection needs to be maintained, thereby increasing the burden on the server. If the amount of concurrency is too large, server performance will decrease.
Secondly, long connections may cause blocking. When the server processing time is too long or the client network is unstable, the connection may be stuck or unresponsive for a long time.
Therefore, when using long connection technology, you need to carefully consider the application scenario, choose the appropriate technical solution, and perform appropriate optimization to ensure the stability and performance of the application.
4. Summary
Long connection technology is an important real-time communication technology that helps improve the real-time nature of applications. Node.js provides a variety of ways to implement long connections, among which socket.io and HTTP long connections are two commonly used technical solutions. When using long connection technology, you need to carefully consider the application scenario and perform appropriate optimization to ensure the stability and performance of the application.
The above is the detailed content of How to implement real long connection in nodejs. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
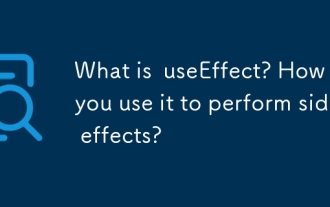
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
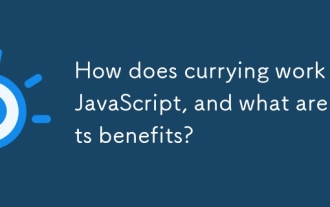
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
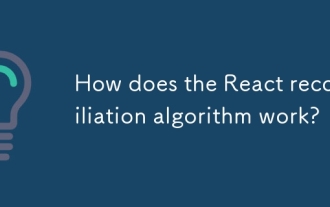
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
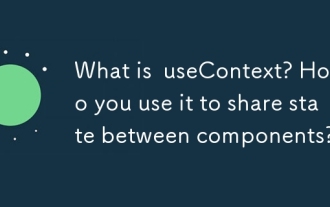
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
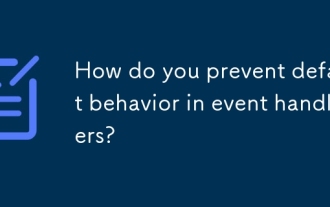
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
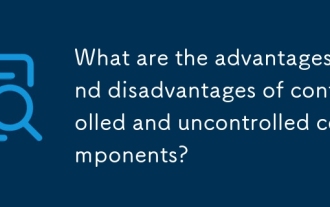
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
