How to use multi-core cpu online in nodejs
With the continuous updating of computer hardware technology, the number of processor cores is also gradually increasing. Many computers are now equipped with multi-core CPUs, which allow us to use computer resources more efficiently to speed up application processing. However, to take full advantage of multi-core CPUs, applications need to be optimized. This article will explain how to take full advantage of multi-core CPUs in Node.js applications.
Node.js is a server-side JavaScript language based on the event-driven non-blocking I/O model. It is highly regarded for its efficient I/O capabilities, lightweight footprint and rapid development efficiency. welcome. However, on multi-core CPUs, the performance of Node.js applications can become very low because Node.js can only use a single CPU core by default. If you want to take full advantage of multi-core CPUs, you need to use the cluster module of Node.js.
The cluster module is a core module of Node.js. It can distribute Node.js applications to multiple sub-processes. Each sub-process runs independently and can take advantage of multi-core CPUs. Using the cluster module can effectively improve the performance of your Node.js application while keeping it simple and easily extensible.
The following are some ways to use the cluster module.
- Create the main process
Before using the cluster module, you need to create a main process first. The main process is a process that does not handle any actual business logic. Its main task is to manage child processes. The master process can be created using the master attribute of the cluster module:
const cluster = require('cluster'); const numCPUs = require('os').cpus().length; if (cluster.isMaster) { console.log(`主进程 ${process.pid} 正在运行`); // Fork workers. for (let i = 0; i < numCPUs; i++) { cluster.fork(); } cluster.on('exit', (worker, code, signal) => { console.log(`工作进程 ${worker.process.pid} 已经退出`); }); } else { console.log(`工作进程 ${process.pid} 已经启动`); // TODO: 实际的业务逻辑处理 }
In this example code, the cluster.isMaster attribute is used to check whether the current process is the master process. If it is the main process, it will start multiple child processes and listen to their exit events. If it's a worker process, it handles the actual business logic.
- Hot code reloading
In the actual development process, code changes often occur. In order to avoid restarting all child processes, the cluster module provides a hot reload method to restart worker processes. You can use cluster.on('SIGUSR2', ...) to listen for signal events. When the signal is received, restart all child processes:
const cluster = require('cluster'); const numCPUs = require('os').cpus().length; function startWorker() { const worker = cluster.fork(); console.log(`工作进程 ${worker.id} 已经启动`); } if (cluster.isMaster) { console.log(`主进程 ${process.pid} 正在运行`); // Fork workers. for (let i = 0; i < numCPUs; i++) { startWorker(); } cluster.on('exit', (worker, code, signal) => { console.log(`工作进程 ${worker.process.pid} 已经退出,重启中...`); startWorker(); }); cluster.on('SIGUSR2', () => { console.log('收到信号,重启所有工作进程...'); const workers = Object.values(cluster.workers); const restartWorker = (workerIndex) => { const worker = workers[workerIndex]; if (!worker) return; worker.on('exit', () => { if (!worker.exitedAfterDisconnect) return; console.log(`工作进程 ${worker.process.pid} 重新启动`); startWorker(); restartWorker(workerIndex + 1); }); worker.disconnect(); } restartWorker(0); }); } else { console.log(`工作进程 ${process.pid} 已经启动`); // TODO: 实际的业务逻辑处理 }
In this sample code, when the SIGUSR2 signal is received , a SIGTERM signal is sent to all worker processes to gracefully shut down them and then restart them.
- Shared server port
In Node.js, multiple processes can share the same server port. This approach improves application performance by enabling each worker process to handle incoming connections. When using the cluster module, you can share server ports using the following code:
const cluster = require('cluster'); const http = require('http'); const numCPUs = require('os').cpus().length; if (cluster.isMaster) { console.log(`主进程 ${process.pid} 正在运行`); // Fork workers. for (let i = 0; i < numCPUs; i++) { cluster.fork(); } cluster.on('exit', (worker, code, signal) => { console.log(`工作进程 ${worker.process.pid} 已经退出`); }); } else { console.log(`工作进程 ${process.pid} 已经启动`); const server = http.createServer((req, res) => { res.writeHead(200); res.end('你好世界 '); }); server.listen(3000, (err) => { if (err) throw err; console.log(`工作进程 ${process.pid} 正在监听3000端口`); }); }
In this example code, each worker process will listen on port 3000. When an incoming connection comes in, the server will route it to different worker processes, improving the performance of your application.
Summary
Using the cluster module can easily make Node.js applications fully utilize computer resources on multi-core CPUs. When using the cluster module, you need to pay attention to the following points:
- The main process is only responsible for managing child processes and does not handle business logic.
- Worker processes should run independently and handle the actual business logic.
- You can use code hot reloading to avoid restarting all worker processes.
- Multiple processes can share the same server port to improve application performance.
To make more effective use of multi-core CPUs, other optimizations are required, such as asynchronous I/O operations, memory management and code optimization. By combining different technologies to optimize your application, you can make it perform better on multi-core CPUs, thus providing a better experience for users.
The above is the detailed content of How to use multi-core cpu online in nodejs. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
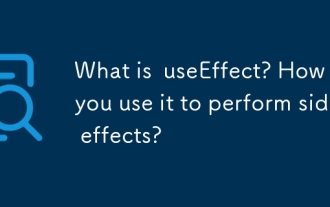
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
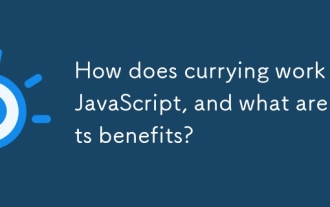
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
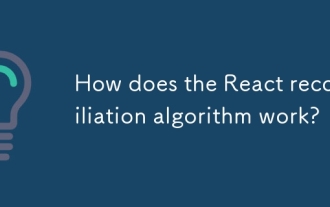
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
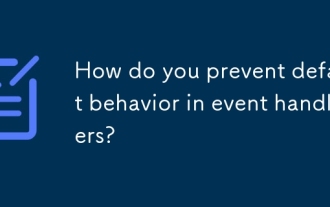
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
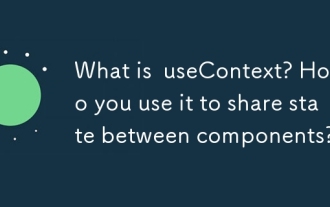
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
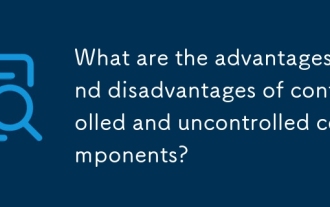
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
