How to store data in nodejs to xml
Node.js is a popular open source backend JavaScript runtime environment used by many developers to build high-performance and scalable web applications. In many cases, these applications need to store and retrieve data. While Node.js has built-in capabilities for file system operations, storing data in XML files may be more convenient in some cases. The following will introduce how to use Node.js to store data in XML.
1. What is XML?
XML is the abbreviation of Extensible Markup Language (Extensible Markup Language). It is a general markup language used to describe data formats. XML files consist of tags, attributes and text and can be nested to represent complex data structures. XML files are readable and extensible, which makes them a popular format for storing and exchanging data.
2. XML parser in Node.js
To store data in Node.js to XML, you first need a parser that can process XML. There are many Node.js modules that can parse XML, the most popular of which is xml2js. xml2js can convert XML files into JavaScript objects, and JavaScript objects can be written to files through the Node.js module fs. After installing and referencing xml2js, you can convert XML files into JavaScript objects and write JavaScript objects into XML files.
The following is a sample code using xml2js:
const fs = require('fs'); const xml2js = require('xml2js'); const builder = new xml2js.Builder(); const obj = { items: { item: [ { name: 'item 1', price: '10.00', }, { name: 'item 2', price: '20.00', }, ], }, }; const xml = builder.buildObject(obj); fs.writeFileSync('data.xml', xml);
In this example, we construct a JavaScript object that represents a product list containing two products. We use the Builder in xml2js to convert the JavaScript object into an XML string and write it to a file named "data.xml".
3. Reading data from XML
After storing data in XML, we may also need to read data from the XML file. Likewise, the xml2js module can be used to parse data from XML files and convert them into JavaScript objects. The following is a sample code using xml2js:
const fs = require('fs'); const xml2js = require('xml2js'); fs.readFile('data.xml', function(err, data) { const parser = new xml2js.Parser(); parser.parseString(data, function (err, result) { console.dir(result); }); });
In this example, we use the fs module to read a file named "data.xml", and then use Parser in xml2js to parse the file content. Parser converts the XML string into a JavaScript object and passes it to the callback function. In the callback function, we use console.dir to output the JavaScript object so that its contents can be viewed in the console.
4. Conclusion
Node.js can store and read XML files by using the xml2js module. xml2js can convert JavaScript objects to XML strings and XML strings to JavaScript objects. XML files are a convenient and readable data storage format for applications that need to store and retrieve data in Node.js.
The above is the detailed content of How to store data in nodejs to xml. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
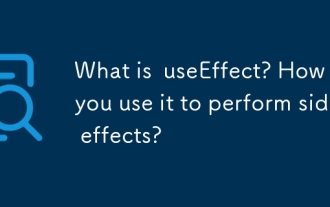
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
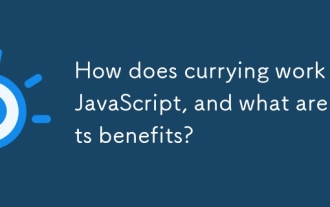
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
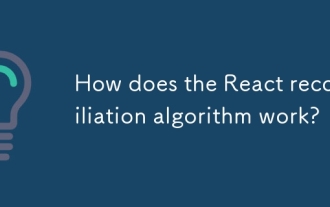
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
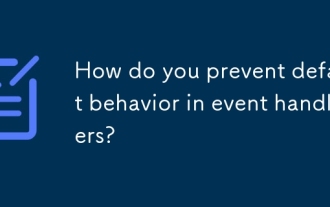
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
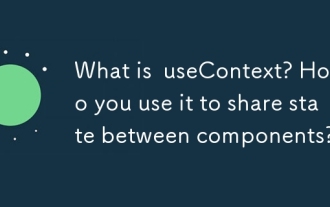
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
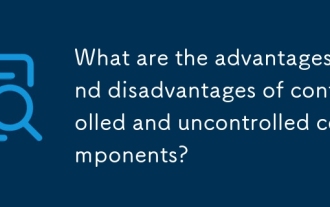
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
