How to use SQLite functions in PHP
With the increasing development of Internet technology, more and more websites and applications require data storage and management. In this regard, relational databases are very important tools that can help developers store and manage data in a fixed format. Currently, the mainstream relational databases include MySQL, Oracle, SQL Server, PostgreSQL, etc. However, for some lightweight applications, using a database such as MySQL is a bit too heavy, and in this case a lighter database is needed.
SQLite is a lightweight database that allows developers to easily embed data storage and management functions in applications. It is an open source relational database system, a fully documented database that can be operated without a network connection. In PHP, we can use SQLite functions to operate on the SQLite database. Below we will introduce in detail how to use SQLite functions in PHP.
- Open SQLite database
First, we need to open a SQLite database so that we can operate on the database. It is very simple to open a SQLite database using PHP. You only need to call the sqlite_open function in PHP that is specially used to open a SQLite database. The sqlite_open function has two parameters. The first parameter is the name of the SQLite database, and the second parameter is the permission setting parameter. The specific usage is as follows:
$db = sqlite_open("testDB.db", 0666, $error);
In the above example code, we opened a SQLite database named testDB.db and set read and write permissions (0666) for it. If the opening fails, $error will Save error message.
- Create SQLite table
Creating a table is an important operation of SQLite database. Although SQLite database is simpler than MySQL and other databases, we still need to First create a table to store data. For the operation of creating a table, we can use SQL statements to operate. In SQLite, one of the very basic SQL statements to create a table is:
CREATE TABLE tableName ( column1Type column1Name, column2Type column2Name, ... );
Among them, tableName represents the name of the table, column1Type and column2Type represent the type of the column, and column1Name and column2Name are the names of the columns. The following is a specific sample code:
$sql = " CREATE TABLE users ( user_id INTEGER PRIMARY KEY, user_name TEXT, user_email TEXT ); "; $result = sqlite_exec($db, $sql);
In the above sample code, we created a table named users. The table has three columns, namely user_id, user_name and user_email. Among them, user_id is set PRIMARY KEY.
- Query SQLite table data
The data saved in the SQLite table can be queried through SQL statements. The following is an example of querying table data:
$sql = "SELECT * FROM users"; $result = sqlite_query($db, $sql); while($row = sqlite_fetch_array($result)){ echo $row['user_name'] . "<br/>"; }
In the above example code, we used the SELECT statement to query the users table and output the queried data to the web page.
- Insert SQLite database data
Inserting data is also a very common operation in SQLite database. The following is an example of inserting data:
$sql = "INSERT INTO users (user_name, user_email) VALUES ('Tom', 'tom@example.com')"; $result = sqlite_exec($db, $sql);
In the above example code, we inserted a new piece of data into the users table, where user_name is Tom and user_email is tom@example.com.
- Modify SQLite database data
Modifying data is also one of the common operations. The following is a simple example:
$sql = "UPDATE users SET user_email = 'new@example.com' WHERE user_id = 1"; $result = sqlite_exec($db, $sql);
In the above example code, We use the UPDATE statement to modify the user_email value of the record with ID 1 in the users table.
- Delete SQLite database data
Deleting data is also a very common operation in SQLite database. The following is an example:
$sql = "DELETE FROM users WHERE user_name = 'Tom'"; $result = sqlite_exec($db, $sql);
In the above example code, we used the DELETE statement to delete the record whose user_name is Tom in the users table.
Summary:
Using SQLite functions in PHP to operate SQLite databases is very simple and easy to use. Using SQLite allows us to easily develop some lightweight applications without installing complex relational database software. SQLite is also widely used in mobile development due to its lightweight and efficient characteristics. I hope this article can be helpful to everyone when using SQLite database.
The above is the detailed content of How to use SQLite functions in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


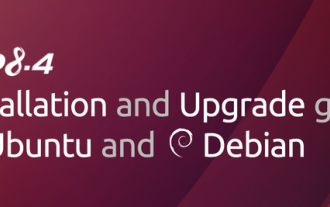
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
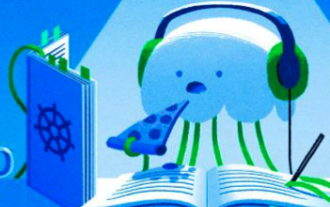
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
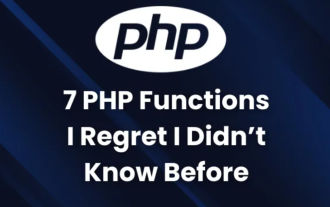
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
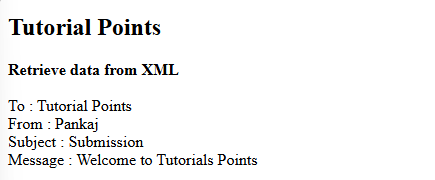
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
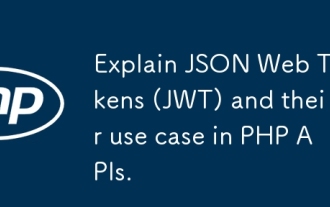
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
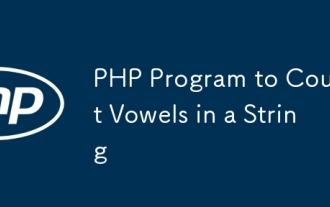
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
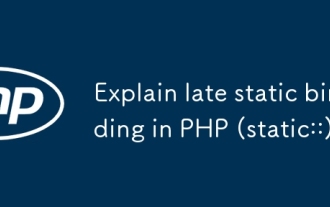
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
