How to use the numpy.ufunc function in Python
1. Description
What is the function of numpy.ufunc? Answer: It is a numpy function, because numpy targets array tensors, so almost every function is a ufunc.
2. Concept of numpy.ufunc function
2.1 Introduction to numpy.ufunc
A function that operates on the entire array element by element. Therefore, ufunc is a general term, and there are many of these functions.
函 (or UFUNC) is a function that operates NDARRAYS in an element way, supporting the array broadcast, type conversion, and other standard functions. Ufunc is a wrapper that "vectorizes" a function, taking a fixed number of specific inputs and producing a fixed number of specific outputs. See details of the underlying universal function (ufunc).
In NumPy, general functions are instances of the numpy.ufunc
class. Many built-in functions are implemented in compiled C code. Basic ufuncs operate on scalars, but there is also a generic type where the basic elements are subarrays (vectors, matrices, etc.) and the broadcasting is done in other dimensions. You can alsoufunc
use the frompyfuncopen in new window factory function to generate a custom instance.
2.2 numpy.ufunc.nin and numpy.ufunc.nout
This function expresses the number of input parameters corresponding to the ufun function, as shown below. The corresponding number of input parameters for ufunc.
np.add.nin 2 np.multiply.nin 2 np.power.nin 2 np.exp.nin 2
This function expresses the number of output parameters corresponding to the ufun function, such as the corresponding number of input parameters for the following ufunc.
np.add.nout 1 np.multiply.nout 1 np.power.nout 1 np.exp.nout 1
2.3 numpy.ufunc.nargs
numpy.ufunc corresponds to the number of parameters,
np.add.nargs 3 np.multiply.nargs 3 np.power.nargs 3 np.exp.nargs 2
For example, the np.add function has three parameters, two inputs and one output , as follows:
a = np.array([2,4,5,6]) b = np.array([2,2,3,3]) c = np.zeros((4,)) np.add( a,b,c ) print( c )
2.4 numpy.ufunc.ntypes
Indicates the input data type format of a ufunc: the number of numeric NumPy types that ufunc can operate - 18 in total.
np.add.ntypes 18 np.multiply.ntypes 18 np.power.ntypes 17 np.exp.ntypes 7 np.remainder.ntypes 14
2.5 numpy.ufunc.type
np.add.types ['??->?', 'bb->b', 'BB->B', 'hh->h', 'HH->H', 'ii->i', 'II->I', 'll->l', 'LL->L', 'qq->q', 'QQ->Q', 'ff->f', 'dd->d', 'gg->g', 'FF->F', 'DD->D', 'GG->G', 'OO->O'] np.multiply.types ['??->?', 'bb->b', 'BB->B', 'hh->h', 'HH->H', 'ii->i', 'II->I', 'll->l', 'LL->L', 'qq->q', 'QQ->Q', 'ff->f', 'dd->d', 'gg->g', 'FF->F', 'DD->D', 'GG->G', 'OO->O'] np.power.types ['bb->b', 'BB->B', 'hh->h', 'HH->H', 'ii->i', 'II->I', 'll->l', 'LL->L', 'qq->q', 'QQ->Q', 'ff->f', 'dd->d', 'gg->g', 'FF->F', 'DD->D', 'GG->G', 'OO->O'] np.exp.types ['f->f', 'd->d', 'g->g', 'F->F', 'D->D', 'G->G', 'O->O'] np.remainder.types ['bb->b', 'BB->B', 'hh->h', 'HH->H', 'ii->i', 'II->I', 'll->l', 'LL->L', 'qq->q', 'QQ->Q', 'ff->f', 'dd->d', 'gg->g', 'OO->O']
2.6 Dimension ndim and shape
There are two parameters indicating the dimension, array.ndim and array.shape, where ndim is Refers to the total number of dimensions of the tensor, and shape refers to the length of the vector in each dimension. For example, the following example:
x = np.array([1, 2, 3]) print(x.ndim) print(x.shape) y = np.zeros((2, 3, 4)) print(y.ndim) print(y.shape)
Result:
1
(3,)
3
(2, 3, 4)
3. Broadcasting characteristics of ufunc
Each pass function accepts an array input and generates an array output by executing the core function element by element on the input (the elements are usually scalars, but can be generalized vector or higher-order subarray of ufunc). Follow standard broadcasting rules to ensure that even if the inputs don't have exactly the same shape, the operation will still work efficiently. Broadcasting can be understood through four rules:
All input arrays are smaller than the largest input array, ndimopen in new window whose shape is preceded by 1.
The size of each dimension of the output shape is the maximum of all input sizes in that dimension.
If the size of the input in a particular dimension matches the size of the output in that dimension, or if its value is exactly 1, then the input can be used in the calculation.
When the shape dimension is 1, the first data entry in that dimension will be used for all calculations along that dimension. In other words, the stepping machine of ufuncopen in new window will not step along that dimension (the stride will be 0 for that dimension).
Broadcasting is used throughout NumPy to decide how to handle arrays of different shapes; for example, all arithmetic operations (
, -
, * Between
,...) ndarrays are broadcast before the array operation in new window.
If the above rules produce valid results, a set of arrays is said to be "broadcastable" to the same shape, that is, one of the following conditions is met:
Arrays are all Have exactly the same shape.
Arrays all have the same number of dimensions, and the length of each dimension is the common length or 1.
An array whose size is too small can have its shape prepended to a size of length 1 to satisfy attribute 2.
If a.shape
is (5,1), b.shape
is (1,6), c. shape
is (6,) and d.shape
is () such that d is a scalar, then a , b , c and d can all be broadcast to dimensions (5, 6); and:
a acts like a (5,6) array, where [:, 0] is broadcast to other columns,
b acts like (5,6) array, where b[0, :] is broadcast to other rows,
c被视为类似于一个(1,6)的矩阵,因此类似于一个(5,6)的矩阵,其中c的每一项元素被广播到每一行,最终,...
d 的作用类似于(5,6)数组,其中重复单个值。
四、函数格式
可以在通用函数 (ufunc) 的文档中找到有关 ufunc 的详细说明。
调用ufuncs格式:
op( *x[, out], where=True, **kwargs)
将 op 应用于参数 *x elementwise,广播参数。
广播规则是:
长度为 1 的维度可以添加到任一数组之前。
数组可以沿长度为 1 的维度重复。
参数:
*xarray_like
outndarray,None,或 ndarray 和 None 的元组,可选
用于放置结果的备用数组对象;如果提供,它必须具有输入广播的形状。数组元组(可能仅作为关键字参数)的长度必须等于输出的数量;对 ufunc 分配的未初始化输出使用 None。
wherearray_like,可选
此条件通过输入广播。当条件为 True 时,ufunc 的结果将被赋值给 out 数组。在其他地方,out 数组将保留其原始值。请注意,如果通过默认 out=None 创建未初始化的 out 数组,则其中条件为 False 的位置将保持未初始化状态。
五、示例详解
5.1 用输出参数
a = np.array([2,4,5,6]) b = np.array([2,2,3,3]) c = np.zeros((4,)) np.add( a,b,c ) print( c )
5.2 行数组和列数组
a = np.arange(3) b = np.arange(3)[:, np.newaxis] print(a) print(b)
输出:
[0 1 2]
[[0]
[1]
[2]]
5.3 广播规则示例
a = np.arange(3) b = np.arange(3)[:, np.newaxis] print(a) print(b) s = a + b print(s)
六、ufunc后的数列运算
6.1 数列运算
在执行ufunc运算后,常常伴随数列运算,它们如下
__call__(*args, **kwargs) | Call self as a function. |
accumulate(array[, axis, dtype, out]) | Accumulate the result of applying the operator to all elements. |
at(a, indices[, b]) | Performs unbuffered in place operation on operand 'a' for elements specified by 'indices'. |
outer(A, B, /, **kwargs) | Apply the ufunc op to all pairs (a, b) with a in A and b in B. |
reduce(array[, axis, dtype, out, keepdims, ...]) | Reduces array's dimension by one, by applying ufunc along one axis. |
reduceat(array, indices[, axis, dtype, out]) | Performs a (local) reduce with specified slices over a single axis. |
resolve_dtypes(dtypes, *[, signature, ...]) | Find the dtypes NumPy will use for the operation. |
6.2 累计模式
累计模式不可以单独使用,而是与add以及multiply搭配使用:
np.add.accumulate([2, 3, 5]) array([ 2, 5, 10]) np.multiply.accumulate([2, 3, 5]) array([ 2, 6, 30])
np.add.accumulate(I, 0) array([[1., 0.], [1., 1.]]) np.add.accumulate(I) # no axis specified = axis zero array([[1., 0.], [1., 1.]])
6.3 对数组中某个index的元素进行局部处理
1) 将项目 0 和 1 设置为负值:
a = np.array([1, 2, 3, 4]) np.negative.at(a, [0, 1]) print( a ) array([-1, -2, 3, 4])
2) 递增项目 0 和 1,递增项目 2 两次:
a = np.array([1, 2, 3, 4]) np.add.at(a, [0, 1, 2, 2], 1) print( a ) array([2, 3, 5, 4])
3) 将第一个数组中的项 0 和 1 添加到第二个数组,并将结果存储在第一个数组中:
a = np.array([1, 2, 3, 4]) b = np.array([1, 2]) np.add.at(a, [0, 1], b) print(a) array([2, 4, 3, 4])
6.4 outer外积
简单数组外积
np.multiply.outer([1, 2, 3], [4, 5, 6]) array([[ 4, 5, 6], [ 8, 10, 12], [12, 15, 18]])
张量的外积
A = np.array([[1, 2, 3], [4, 5, 6]]) A.shape (2, 3) B = np.array([[1, 2, 3, 4]]) B.shape (1, 4) C = np.multiply.outer(A, B) C.shape; C (2, 3, 1, 4) array([[[[ 1, 2, 3, 4]], [[ 2, 4, 6, 8]], [[ 3, 6, 9, 12]]], [[[ 4, 8, 12, 16]], [[ 5, 10, 15, 20]], [[ 6, 12, 18, 24]]]])
6.5 reduce方法
a = np.multiply.reduce([2,3,5]) print( a) 30
X = np.arange(8).reshape((2,2,2)) X array([[[0, 1], [2, 3]], [[4, 5], [6, 7]]]) np.add.reduce(X, 0) array([[ 4, 6], [ 8, 10]]) np.add.reduce(X) # confirm: default axis value is 0 array([[ 4, 6], [ 8, 10]]) np.add.reduce(X, 1) array([[ 2, 4], [10, 12]]) np.add.reduce(X, 2) array([[ 1, 5], [ 9, 13]])
您可以使用 initial 关键字参数以不同的值初始化缩减,以及在何处选择要包含的特定元素:
np.add.reduce([10], initial=5) 15 np.add.reduce(np.ones((2, 2, 2)), axis=(0, 2), initial=10) array([14., 14.]) a = np.array([10., np.nan, 10]) np.add.reduce(a, where=~np.isnan(a)) 20.0
np.minimum.reduce([], initial=np.inf) inf np.minimum.reduce([[1., 2.], [3., 4.]], initial=10., where=[True, False]) array([ 1., 10.]) np.minimum.reduce([]) Traceback (most recent call last):
The above is the detailed content of How to use the numpy.ufunc function in Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
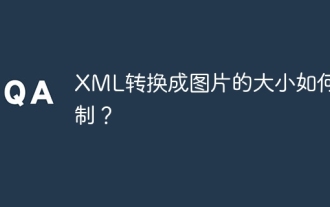
To generate images through XML, you need to use graph libraries (such as Pillow and JFreeChart) as bridges to generate images based on metadata (size, color) in XML. The key to controlling the size of the image is to adjust the values of the <width> and <height> tags in XML. However, in practical applications, the complexity of XML structure, the fineness of graph drawing, the speed of image generation and memory consumption, and the selection of image formats all have an impact on the generated image size. Therefore, it is necessary to have a deep understanding of XML structure, proficient in the graphics library, and consider factors such as optimization algorithms and image format selection.
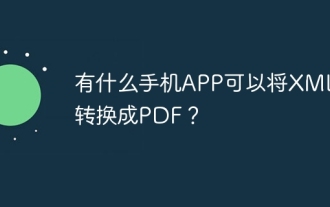
An application that converts XML directly to PDF cannot be found because they are two fundamentally different formats. XML is used to store data, while PDF is used to display documents. To complete the transformation, you can use programming languages and libraries such as Python and ReportLab to parse XML data and generate PDF documents.
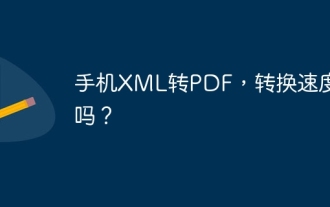
The speed of mobile XML to PDF depends on the following factors: the complexity of XML structure. Mobile hardware configuration conversion method (library, algorithm) code quality optimization methods (select efficient libraries, optimize algorithms, cache data, and utilize multi-threading). Overall, there is no absolute answer and it needs to be optimized according to the specific situation.
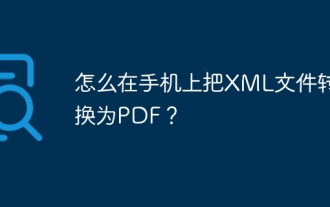
It is impossible to complete XML to PDF conversion directly on your phone with a single application. It is necessary to use cloud services, which can be achieved through two steps: 1. Convert XML to PDF in the cloud, 2. Access or download the converted PDF file on the mobile phone.
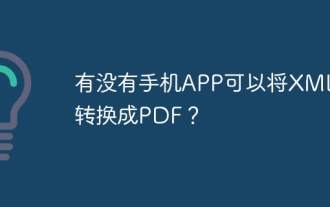
There is no APP that can convert all XML files into PDFs because the XML structure is flexible and diverse. The core of XML to PDF is to convert the data structure into a page layout, which requires parsing XML and generating PDF. Common methods include parsing XML using Python libraries such as ElementTree and generating PDFs using ReportLab library. For complex XML, it may be necessary to use XSLT transformation structures. When optimizing performance, consider using multithreaded or multiprocesses and select the appropriate library.
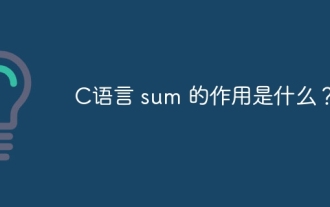
There is no built-in sum function in C language, so it needs to be written by yourself. Sum can be achieved by traversing the array and accumulating elements: Loop version: Sum is calculated using for loop and array length. Pointer version: Use pointers to point to array elements, and efficient summing is achieved through self-increment pointers. Dynamically allocate array version: Dynamically allocate arrays and manage memory yourself, ensuring that allocated memory is freed to prevent memory leaks.
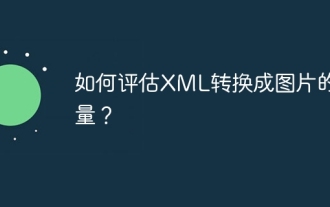
The quality evaluation of XML to pictures involves many indicators: Visual fidelity: The picture accurately reflects XML data, manual or algorithm evaluation; Data integrity: The picture contains all necessary information, automated test verification; File size: The picture is reasonable, affecting loading speed and details; Rendering speed: The image is generated quickly, depending on the algorithm and hardware; Error handling: The program elegantly handles XML format errors and data missing.
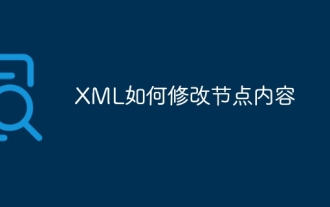
XML node content modification skills: 1. Use the ElementTree module to locate nodes (findall(), find()); 2. Modify text attributes; 3. Use XPath expressions to accurately locate them; 4. Consider encoding, namespace and exception handling; 5. Pay attention to performance optimization (avoid repeated traversals)
