How does the springboot project read files in the resources directory?
1: Use the ClassLoader.getResourceAsStream() method
You can use the class loader to obtain the input stream of the resource file. This method requires passing in a resource file path as a parameter, and then returns an InputStream object.
InputStream inputStream = getClass().getClassLoader().getResourceAsStream("file.txt");
Note that the resource file path returned by this method is relative to the root path of the class loader. Therefore, for files in the resources directory, you need to prefix the file name with "classpath:". For example: "classpath:file.txt".
2: Use the Class.getResourceAsStream() method
To read resource files, you can use the getResourceAsStream() method of the Class class. This method requires inputting the path to a resource file and returns an InputStream object.
InputStream inputStream = getClass().getResourceAsStream("/file.txt");
The resource file path returned by this method is relative to the path of the current class. Therefore, for files in the resources directory, you need to add "/" prefix before the file name. For example: "/file.txt".
3: Use ResourceLoader to load files
Use Spring's ResourceLoader interface to load resource files. The ResourceLoader interface has a getResource() method, which accepts a resource file path parameter and returns a Resource object.
Resource resource = resourceLoader.getResource("classpath:file.txt"); InputStream inputStream = resource.getInputStream();
It should be noted that the ResourceLoader object needs to be injected into the class and used in the method. For example:
@Autowired private ResourceLoader resourceLoader; public void readResourceFile() throws IOException { Resource resource = resourceLoader.getResource("classpath:file.txt"); InputStream inputStream = resource.getInputStream(); }
4: Use ResourceUtils to load files
Spring provides the ResourceUtils tool class, which can be used to load resource files. To obtain a file object, use the ResourceUtils.getFile() method.
File file = ResourceUtils.getFile("classpath:file.txt");
It should be noted that this method only applies to local file systems and JAR files. This method may not work when working with WAR files or other types of files.
5: Load files using ApplicationContext
To load resource files, you can use the getResource() method in ApplicationContext. A method that accepts a resource file path as a parameter and returns a Resource object.
Resource resource = applicationContext.getResource("classpath:file.txt"); InputStream inputStream = resource.getInputStream();
It should be noted that the ApplicationContext object needs to be injected into the class and used in the method. For example:
@Autowired private ApplicationContext applicationContext; public void readResourceFile() throws IOException { Resource resource = applicationContext.getResource("classpath:file.txt"); InputStream inputStream = resource.getInputStream(); }
6: Use ServletContext to load files
You can use the getResourceAsStream() method of ServletContext to read resource files. The parameter of this function is the resource file path and returns an InputStream object.
InputStream inputStream = servletContext.getResourceAsStream("/WEB-INF/classes/file.txt");
It should be noted that the ServletContext object needs to be injected into the class and used in the method. For example:
@Autowired private ServletContext servletContext; public void readResourceFile() throws IOException { InputStream inputStream = servletContext.getResourceAsStream("/WEB-INF/classes/file.txt"); }
7: Use File System to load files
You can use the File class to read resource files. The full file path is required.
File file = new File("src/main/resources/file.txt"); InputStream inputStream = new FileInputStream(file);
It should be noted that using this method requires providing the complete file path, so you need to know the absolute path where the file is located.
8: Use Paths and Files to load files
In Java NIO, you can read resource files with the help of the Paths and Files classes. This method requires the full file path.
Path path = Paths.get("src/main/resources/file.txt"); InputStream inputStream = Files.newInputStream(path);
It should be noted that using this method requires providing the complete file path, so you need to know the absolute path where the file is located.
9: Use ClassPathResource to load files
Use the ClassPathResource class provided by Spring to read resource files. This method requires the relative path of the resource file.
ClassPathResource resource = new ClassPathResource("file.txt"); InputStream inputStream = resource.getInputStream();
It should be noted that ClassPathResource will search for resource files on the class path, so there is no need to provide the complete file path.
The above is the detailed content of How does the springboot project read files in the resources directory?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


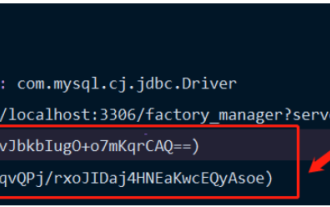
Introduction to Jasypt Jasypt is a java library that allows a developer to add basic encryption functionality to his/her project with minimal effort and does not require a deep understanding of how encryption works. High security for one-way and two-way encryption. , standards-based encryption technology. Encrypt passwords, text, numbers, binaries... Suitable for integration into Spring-based applications, open API, for use with any JCE provider... Add the following dependency: com.github.ulisesbocchiojasypt-spring-boot-starter2. 1.1Jasypt benefits protect our system security. Even if the code is leaked, the data source can be guaranteed.
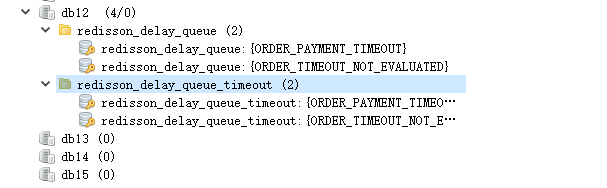
Usage scenario 1. The order was placed successfully but the payment was not made within 30 minutes. The payment timed out and the order was automatically canceled. 2. The order was signed and no evaluation was conducted for 7 days after signing. If the order times out and is not evaluated, the system defaults to a positive rating. 3. The order is placed successfully. If the merchant does not receive the order for 5 minutes, the order is cancelled. 4. The delivery times out, and push SMS reminder... For scenarios with long delays and low real-time performance, we can Use task scheduling to perform regular polling processing. For example: xxl-job Today we will pick
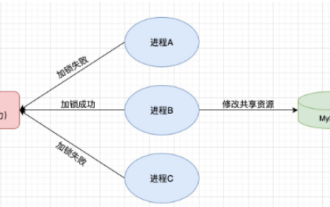
1. Redis implements distributed lock principle and why distributed locks are needed. Before talking about distributed locks, it is necessary to explain why distributed locks are needed. The opposite of distributed locks is stand-alone locks. When we write multi-threaded programs, we avoid data problems caused by operating a shared variable at the same time. We usually use a lock to mutually exclude the shared variables to ensure the correctness of the shared variables. Its scope of use is in the same process. If there are multiple processes that need to operate a shared resource at the same time, how can they be mutually exclusive? Today's business applications are usually microservice architecture, which also means that one application will deploy multiple processes. If multiple processes need to modify the same row of records in MySQL, in order to avoid dirty data caused by out-of-order operations, distribution needs to be introduced at this time. The style is locked. Want to achieve points
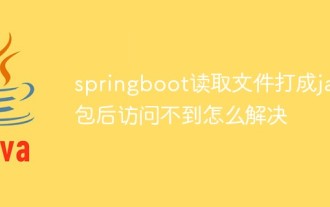
Springboot reads the file, but cannot access the latest development after packaging it into a jar package. There is a situation where springboot cannot read the file after packaging it into a jar package. The reason is that after packaging, the virtual path of the file is invalid and can only be accessed through the stream. Read. The file is under resources publicvoidtest(){Listnames=newArrayList();InputStreamReaderread=null;try{ClassPathResourceresource=newClassPathResource("name.txt");Input
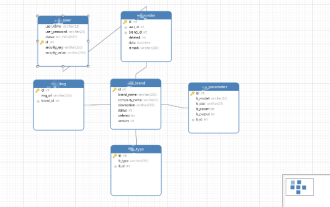
When Springboot+Mybatis-plus does not use SQL statements to perform multi-table adding operations, the problems I encountered are decomposed by simulating thinking in the test environment: Create a BrandDTO object with parameters to simulate passing parameters to the background. We all know that it is extremely difficult to perform multi-table operations in Mybatis-plus. If you do not use tools such as Mybatis-plus-join, you can only configure the corresponding Mapper.xml file and configure The smelly and long ResultMap, and then write the corresponding sql statement. Although this method seems cumbersome, it is highly flexible and allows us to
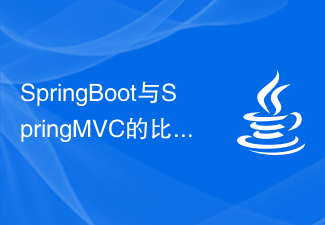
SpringBoot and SpringMVC are both commonly used frameworks in Java development, but there are some obvious differences between them. This article will explore the features and uses of these two frameworks and compare their differences. First, let's learn about SpringBoot. SpringBoot was developed by the Pivotal team to simplify the creation and deployment of applications based on the Spring framework. It provides a fast, lightweight way to build stand-alone, executable
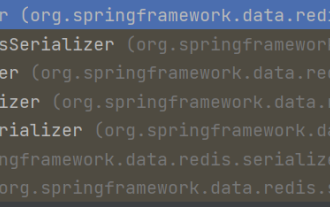
1. Customize RedisTemplate1.1, RedisAPI default serialization mechanism. The API-based Redis cache implementation uses the RedisTemplate template for data caching operations. Here, open the RedisTemplate class and view the source code information of the class. publicclassRedisTemplateextendsRedisAccessorimplementsRedisOperations, BeanClassLoaderAware{//Declare key, Various serialization methods of value, the initial value is empty @NullableprivateRedisSe
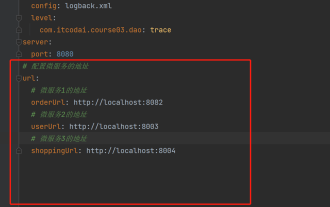
In projects, some configuration information is often needed. This information may have different configurations in the test environment and the production environment, and may need to be modified later based on actual business conditions. We cannot hard-code these configurations in the code. It is best to write them in the configuration file. For example, you can write this information in the application.yml file. So, how to get or use this address in the code? There are 2 methods. Method 1: We can get the value corresponding to the key in the configuration file (application.yml) through the ${key} annotated with @Value. This method is suitable for situations where there are relatively few microservices. Method 2: In actual projects, When business is complicated, logic
