How to reset the key value of a two-dimensional array in php
In PHP, resetting the key value of a two-dimensional array is a very common operation. It can make the array more convenient and faster when performing some operations that require a specific order. This article will introduce in detail the method and implementation steps of resetting the key value of a two-dimensional array.
What is a two-dimensional array?
In PHP, array is a very common data type. It can be used to store multiple related data and can be accessed in a specific order. A two-dimensional array refers to an array composed of multiple arrays, that is, each element in the array is a subarray. For example:
$students = array( array("name"=>"张三", "age"=>18, "gender"=>"男"), array("name"=>"李四", "age"=>20, "gender"=>"男"), array("name"=>"王五", "age"=>19, "gender"=>"女") );
In the above example, $students is a two-dimensional array containing three elements. Each element is a subarray containing three key-value pairs: name, age, and gender. We can access the elements in this two-dimensional array in the following ways:
echo $students[0]["name"]; // 输出:张三 echo $students[1]["gender"]; // 输出:男 echo $students[2]["age"]; // 输出:19
Reset the key value of the two-dimensional array
In some cases, we need to reset the key value of the two-dimensional array Reset, this operation can make the array more tidy and standardized, thus facilitating subsequent related operations. For example, when we need to sort an array according to a certain key value, if the order of the key values is not standardized, it will affect the sorting result.
The method to reset the key value of a two-dimensional array is very simple. You only need to use PHP's built-in function array_values(). This function returns a new array in which the keys of all subarrays are reset to numeric sequences, starting from 0.
For example, in the $students array above, assuming we only need the two key values name and gender, we can use the following code to reset the key values of the $students array:
$new_students = array(); foreach ($students as $student) { $new_students[] = array("name"=>$student["name"], "gender"=>$student["gender"]); } $new_students = array_values($new_students);
In the above code, we first use a foreach loop to traverse each element in the $students array, and then extract the name and gender key-value pairs to build a new array $new_students. Finally, we use the array_values() function to reset the key values of the $new_students array and obtain a new two-dimensional array containing two elements.
Summary
Resetting the key value of a two-dimensional array is a very common operation in PHP. It can make the array more tidy and standardized, thereby facilitating related operations. In PHP, the array_values() function can be used to easily reset the key values of a two-dimensional array. I hope this article can help readers better understand and apply this operation.
The above is the detailed content of How to reset the key value of a two-dimensional array in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
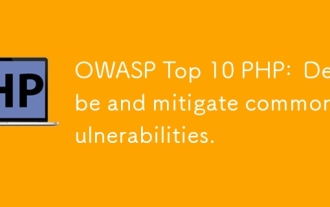
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
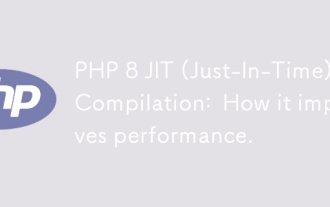
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
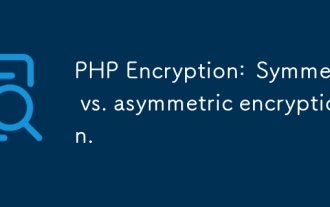
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.
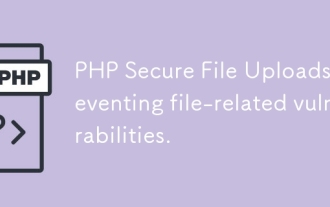
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
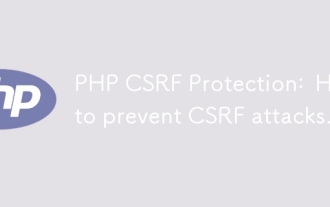
The article discusses strategies to prevent CSRF attacks in PHP, including using CSRF tokens, Same-Site cookies, and proper session management.

Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
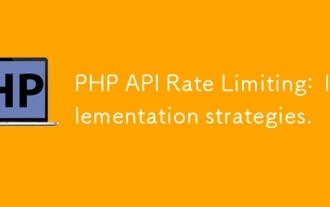
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
