Are two arrays nested loops in php?
In PHP, nested loops of two arrays can be implemented using the foreach
statement. Nested loops make it easier to perform operations on two arrays, such as searching, comparing, merging, etc.
The following is a simple example that shows how to use nested loops to find correspondences in two arrays:
$students = array( array('name' => 'Alice', 'id' => 1001), array('name' => 'Bob', 'id' => 1002), array('name' => 'Cathy', 'id' => 1003), ); $scores = array( array('id' => 1001, 'score' => 85), array('id' => 1002, 'score' => 90), array('id' => 1003, 'score' => 88), ); foreach ($students as $student) { foreach ($scores as $score) { if ($student['id'] == $score['id']) { echo $student['name'] . '的分数是:' . $score['score'] . '<br>'; break; } } }
In the above example, $students
In the array Some student information is saved, including name and student number. $scores
The array stores the student's score information, including student number and score. Next, use two nested foreach
to loop through the two arrays, find the correspondence between students and scores, and output the scores of each student.
In a nested loop, the inner loop is responsible for traversing the internal array, performing comparison operations in each traversal, processing and terminating the inner loop after finding items that meet the conditions. The outer loop is responsible for traversing the outer array and providing each element that needs to be compared.
It should be noted that there will be efficiency problems in nested loops. When the two arrays are large, the number of loops will be very large, causing a performance bottleneck. Therefore, in actual development, you should try to avoid too many nested loops. You can consider processing arrays to reduce the number of loops and improve program efficiency.
In general, nested loops in PHP can easily operate multiple arrays and implement various complex logic. However, you need to pay attention to the number of loops to avoid affecting program performance.
The above is the detailed content of Are two arrays nested loops in php?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


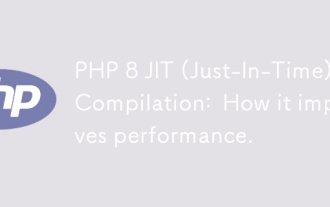
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
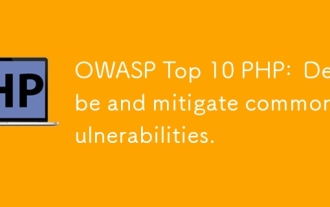
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
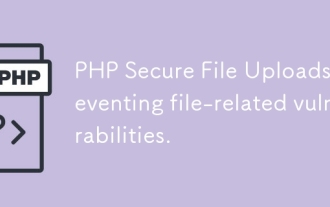
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
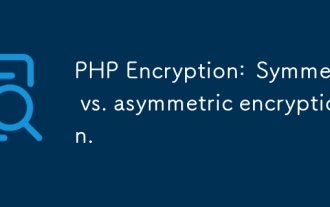
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
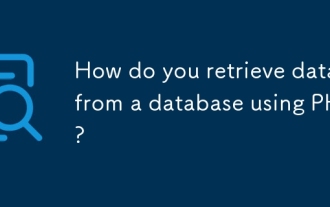
Article discusses retrieving data from databases using PHP, covering steps, security measures, optimization techniques, and common errors with solutions.Character count: 159
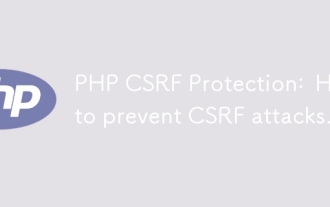
The article discusses strategies to prevent CSRF attacks in PHP, including using CSRF tokens, Same-Site cookies, and proper session management.
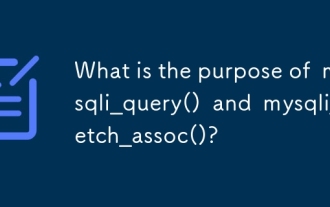
The article discusses the mysqli_query() and mysqli_fetch_assoc() functions in PHP for MySQL database interactions. It explains their roles, differences, and provides a practical example of their use. The main argument focuses on the benefits of usin
