golang pipeline implementation
Golang is an efficient and simple programming language, and its concurrency mechanism has also become one of its hot topics. Among them, Golang's channel has become one of the necessary tools for efficient concurrency. This article will introduce the basic concepts of Golang pipelines and how to use pipelines to achieve concurrency.
1. The basic concept of pipeline
Golang’s pipeline can be regarded as a communication bridge, used to connect goroutines of different operations. In Golang, when multiple goroutines access the same resource at the same time, we need to use locks or pipes to coordinate their access. Locks have certain limitations, because when we use locks, they only allow one goroutine to access resources, and usually we need a more efficient way to coordinate the concurrent operations of goroutines, and then we need to use pipelines.
Pipeline is a concurrency-safe data structure that is very simple to use. In Golang, we can use the built-in function make() to create a pipeline as follows:
ch := make(chan int) // 其中 int 为传输值的类型
When creating a pipeline, we need to specify the type of value transferred by the pipeline. The value transmitted by the pipeline can be of any type, not limited to basic data types, structures, arrays, pointers, etc.
After creating the pipeline, we can use <- to send values to the pipeline and <-chan to receive values from the pipeline. For example:
ch <- 1 // 向管道发送值 x <- ch // 从管道接收值
It is worth noting that if no value is received when we receive a value from the pipe, the goroutine will block until another goroutine sends a value to the pipe. Likewise, when sending a value to a pipe, if the pipe is full, the goroutine will be blocked until another goroutine receives a value from the pipe.
2. Application scenarios of pipelines
Golang pipelines have a very wide range of application scenarios, such as:
- Data interaction between multiple goroutines. When multiple goroutines execute simultaneously, a mechanism is needed to synchronize and coordinate their operations. Pipes can be used as a tool for sharing data, helping data exchange and communication between different goroutines.
- Data current limiting control. In practical applications, we need to control the execution speed of the program to avoid tasks processing too much data at the same time and causing the program to crash. Using pipes can solve this problem very well.
- Resolve data synchronization between producers and consumers. Between producers and consumers, we usually need an intermediate link to coordinate their operations. The pipeline can be used as an intermediate link, allowing producers to wait for consumers to retrieve data, ensuring data synchronization between producers and consumers.
3. Use pipelines to achieve concurrency
In order to better understand how to use pipelines to achieve concurrent operations, let's look at a simple example: using pipelines to calculate average values. We accomplish this task by collaborating with multiple goroutines.
The code looks like this:
package main import ( "fmt" "math/rand" "time" ) var nums = make([]int, 100) var ch = make(chan int, 10) func main() { rand.Seed(time.Now().UnixNano()) for i := 0; i < 100; i++ { nums[i] = rand.Intn(100) } for _, num := range nums { ch <- num // 向管道发送值 } close(ch) // 关闭管道 sum := 0 cnt := 0 for val := range ch { sum += val cnt++ } avg := float64(sum) / float64(cnt) fmt.Println("平均值:", avg) }
In this example code, we first create a slice that stores 100 random integers. We then create a pipe of size 10 and send 100 integers into the pipe one after another. We then close the pipe and use a for-range loop to receive the values from the pipe, sum up all the values and finally calculate the average.
Through this simple example, we can see the advantages of using pipelines to achieve concurrent operations. It allows us to perform operations on multiple goroutines at the same time, and transferring data between different goroutines is more flexible and efficient. At the same time, by using pipelines, we can also solve some problems in concurrent operations, such as data synchronization and current limiting control.
4. Summary
Golang pipeline is an important tool to achieve efficient concurrent operations. Its simple implementation and efficient operating mechanism make it an important part of Golang concurrent programming. In practical applications, we can achieve efficient concurrent operations by using pipelines to solve data exchange, synchronization and control between multiple goroutines. At the same time, we also need to pay attention to problems that may arise when using pipelines, such as deadlocks, etc., to ensure that we can use pipelines efficiently and effectively to implement concurrent programming.
The above is the detailed content of golang pipeline implementation. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


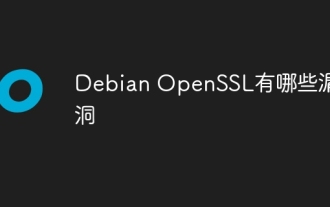
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
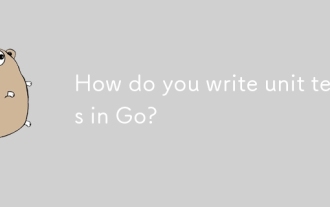
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
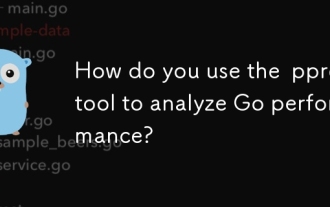
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
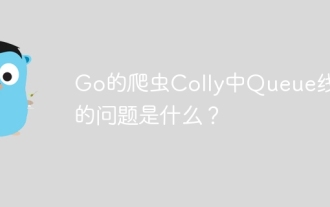
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
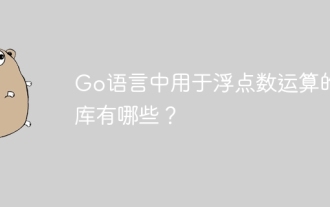
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
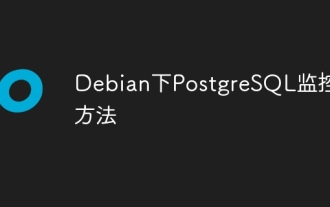
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
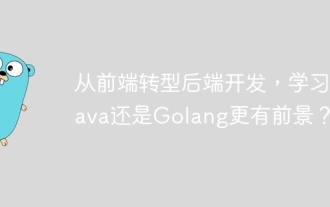
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
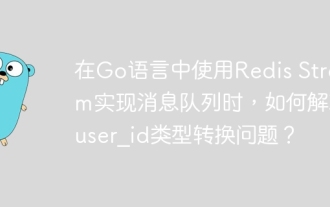
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
