How to quickly remove a two-dimensional array in php
In the development of PHP, it is often necessary to process data, such as deduplication, sorting, filtering, etc. Among them, processing two-dimensional arrays is a very common operation. So, how to quickly remove duplicate data in a two-dimensional array? Below we will introduce you to several implementation methods.
Method 1: Use array_unique() function
array_unique() function can quickly remove duplicate data in the array. The specific implementation method is as follows:
$array = array( array('id' => 1, 'name' => '小明'), array('id' => 2, 'name' => '小红'), array('id' => 3, 'name' => '小明') ); $newArray = array_map("serialize", $array); $newArray = array_unique($newArray); $newArray = array_map("unserialize", $newArray); print_r($newArray);
Output result:
Array ( [0] => Array ( [id] => 1 [name] => 小明 ) [1] => Array ( [id] => 2 [name] => 小红 ) )
Method 2: Use loop traversal
Loop through the array, compare each sub-array with the previous sub-array, and delete it if they are the same. The specific code implementation is as follows:
$array = array( array('id' => 1, 'name' => '小明'), array('id' => 2, 'name' => '小红'), array('id' => 3, 'name' => '小明') ); for($i = 0; $i < count($array) - 1; $i++){ for($j = $i + 1; $j < count($array); $j++){ if($array[$i] == $array[$j]){ unset($array[$j]); } } } print_r($array);
Output result:
Array ( [0] => Array ( [id] => 1 [name] => 小明 ) [1] => Array ( [id] => 2 [name] => 小红 ) )
Method 3: Use the array_walk() function
The array_walk() function allows us to customize the function for processing arrays. The specific implementation method is as follows:
$array = array( array('id' => 1, 'name' => '小明'), array('id' => 2, 'name' => '小红'), array('id' => 3, 'name' => '小明') ); $tempArr = array(); array_walk($array, function($value, $key) use (&$tempArr) { if(!in_array($value, $tempArr)){ array_push($tempArr, $value); } }); print_r($tempArr);
Output results:
Array ( [0] => Array ( [id] => 1 [name] => 小明 ) [1] => Array ( [id] => 2 [name] => 小红 ) )
The above are three methods to quickly remove duplicate data in two-dimensional arrays. The specific method to use depends on the actual situation. If it is just simple deduplication, you can use the array_unique() function; if the amount of data is relatively small, you can use loop traversal; if the amount of data is large, you can use the array_walk() function.
The above is the detailed content of How to quickly remove a two-dimensional array in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


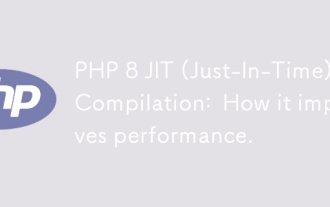
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
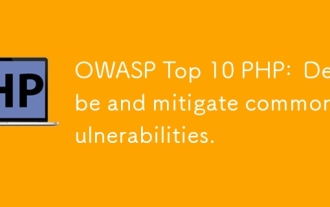
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
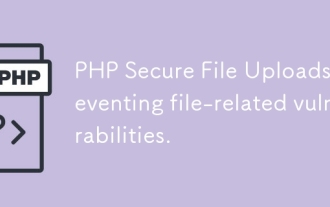
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
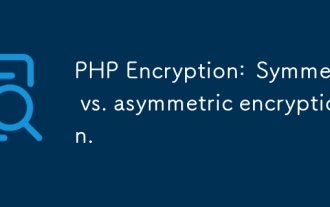
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
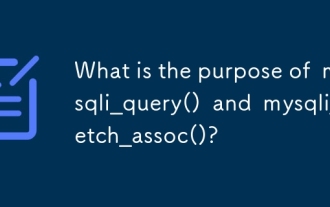
The article discusses the mysqli_query() and mysqli_fetch_assoc() functions in PHP for MySQL database interactions. It explains their roles, differences, and provides a practical example of their use. The main argument focuses on the benefits of usin
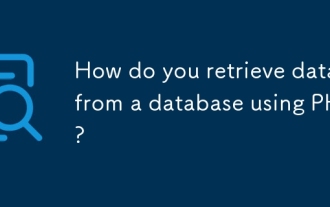
Article discusses retrieving data from databases using PHP, covering steps, security measures, optimization techniques, and common errors with solutions.Character count: 159
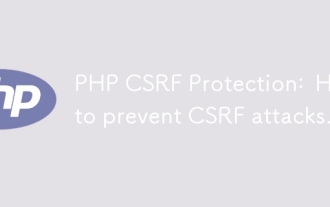
The article discusses strategies to prevent CSRF attacks in PHP, including using CSRF tokens, Same-Site cookies, and proper session management.
