


PHP determines whether a string exists in a multidimensional array
PHP is a powerful programming language that can easily create and manipulate multi-dimensional arrays. When dealing with multi-dimensional arrays, we often need to determine whether a specific string exists in the array. This article will introduce how to use PHP to determine whether a specified string exists in a multidimensional array.
First of all, we need to understand what a multidimensional array is. A multidimensional array is an array that contains other arrays. In PHP, multidimensional arrays can be implemented using nested arrays. For example, the following is an example of a two-dimensional array:
$students = array( array("name"=>"张三", "age"=>18, "class"=>"一班"), array("name"=>"李四", "age"=>19, "class"=>"二班"), array("name"=>"王五", "age"=>20, "class"=>"三班") );
This array contains three sub-arrays, and each sub-array contains three key-value pairs, representing the student's name, age and class respectively. If we want to determine whether a student's name exists in this array, we can follow the following steps:
- Use
foreach
to loop through each sub-array in the array. - While traversing the subarray, use
foreach
again to loop through each key-value pair in the current subarray. - Determine whether the value in the current key-value pair is equal to the string to be found.
- If the specified string is found, exit the loop and return
true
; otherwise, continue searching until the entire array is traversed.
The following is an example of using code to implement the above steps:
function searchArrayValue($array, $searchValue) { foreach ($array as $subArray) { foreach ($subArray as $key => $value) { if ($value === $searchValue) { return true; } } } return false; } // 在$students数组中查找姓张的学生是否存在 if (searchArrayValue($students, "张")) { echo "找到了姓张的学生!"; } else { echo "没有找到姓张的学生。"; }
In this example, we define a searchArrayValue
function to implement the search operation. This function accepts two parameters, the first is the array to be found, and the second is the string to be found. Inside the function, we first use foreach
to traverse each sub-array, and then use another foreach
to traverse each key-value pair in the current sub-array. In each key-value pair, we determine whether the value is equal to the string to be found. If equal, the specified string is found, and the function returns true
. If the entire array is traversed and no one is found, false
is returned to indicate that it cannot be found.
It should be noted that when using ===
to determine whether they are equal, the comparison operator must be three equal signs, not two equal signs. This is because in PHP, two equal signs will perform type conversion, which may cause misjudgment. Using three equal signs ensures that no type conversion is performed, and true
will be returned only if the type and value are equal.
If what we want to judge is a multi-dimensional associative array, we can also operate in a similar way. For example, the following is an example of a three-dimensional associative array:
$employees = array( "部门A" => array( array("name" => "张三", "gender" => "男", "salary" => 5000), array("name" => "李四", "gender" => "女", "salary" => 6000), ), "部门B" => array( array("name" => "王五", "gender" => "男", "salary" => 7000), array("name" => "赵六", "gender" => "女", "salary" => 8000), ) );
This array represents employee information in two departments. Each employee contains three attributes: name, gender, and salary. If we want to find whether female employees exist in the entire array, we can do it as follows:
function searchArrayValue2($array, $searchValue) { foreach ($array as $subArray) { foreach ($subArray as $value) { foreach ($value as $key => $val) { if ($val === $searchValue) { return true; } } } } return false; } // 在$employees数组中查找女性员工是否存在 if (searchArrayValue2($employees, "女")) { echo "找到了女性员工!"; } else { echo "没有找到女性员工。"; }
In this example, we define a function named searchArrayValue2
, and the previous The function is similar, except that the multi-dimensional array passed in this time is an associative array rather than an index array. Inside the function, we use three foreach
loops to traverse each department, each employee and each attribute, and determine whether it is equal to the string to be found. Similarly, if the string is found, true
is returned to indicate that the search was successful. Otherwise, false
is returned to indicate that the search failed.
To sum up, using PHP to determine whether a string exists in a multi-dimensional array is a basic operation and is often used in practical applications. Through the introduction of this article, readers can master the method of judging the existence of strings in multi-dimensional arrays, and can flexibly apply it to their own projects.
The above is the detailed content of PHP determines whether a string exists in a multidimensional array. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


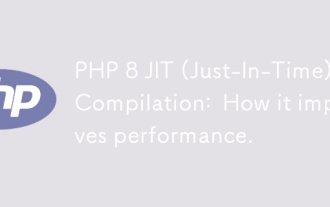
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
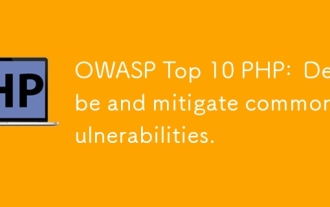
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
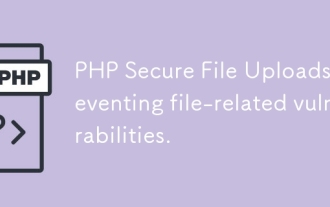
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
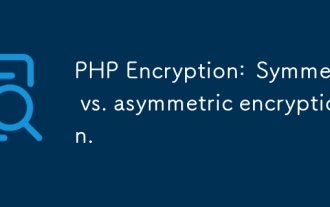
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
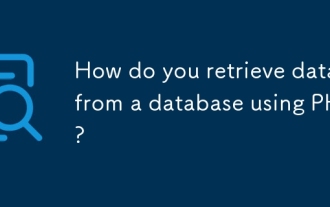
Article discusses retrieving data from databases using PHP, covering steps, security measures, optimization techniques, and common errors with solutions.Character count: 159
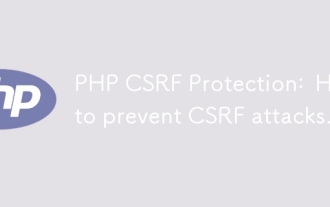
The article discusses strategies to prevent CSRF attacks in PHP, including using CSRF tokens, Same-Site cookies, and proper session management.

Prepared statements in PHP enhance database security and efficiency by preventing SQL injection and improving query performance through compilation and reuse.Character count: 159
