Getting Started with PHP: Form Processing
PHP is a very common server-side programming language that is widely used, especially in web development. By using PHP, we can access databases, create dynamic pages, collect data from forms, etc. This article will introduce how to use PHP to process forms.
- Prerequisite knowledge
Before we start learning PHP, we need to master some basic knowledge. First, we need to understand HTML forms and form elements, including text boxes, drop-down boxes, radio buttons, multi-select boxes, etc. Secondly, we need to be familiar with the HTTP protocol, HTTP requests and HTTP responses. Finally, we need to have some programming experience and understand basic concepts such as variables, functions, and control structures.
- Submit form data
First, we need to write the form containing the form elements in HTML. The form data will be sent to the server side via HTTP request. We can submit form data using POST or GET method. The main difference between these two methods is the way the data is sent.
When submitting a form using the POST method, the form data will be included in the body part of the HTTP request, while the GET method places the form data in the query string of the URL. The GET method is not suitable for forms containing large amounts of data because the URL has a length limit.
For example, the following code is a simple HTML form:
<form action="process.php" method="post"> <label for="name">姓名:</label> <input type="text" name="name" id="name"> <br> <label for="email">邮箱:</label> <input type="email" name="email" id="email"> <br> <input type="submit" value="提交"> </form>
When we submit the form, the server will pass the data to the PHP script named "process.php" for processing.
- Processing form data
Before receiving form data, we need to perform validation and filtering. This can be done by writing JavaScript, but client-side validation is not secure because an attacker can disable JavaScript or tamper with client-side validation rules.
On the server side, we can use PHP's built-in functions for verification and filtering. For example, the trim() function can remove spaces before and after the string, the stripslashes() function can remove backslashes, and the htmlentities() function can Escape HTML entities, etc. We should choose the appropriate function for processing according to the specific situation.
To process form data, we can use $_POST or $_GET arrays. These two arrays contain all the data passed when submitting the form. For example, if we submit the above form using the POST method, then we can use the following code to get the form data:
$name = $_POST['name']; $email = $_POST['email'];
After processing the form data, we need to store or further process it. For example, in the above example, we can store the form data into a database:
// 连接数据库 $conn = mysqli_connect('localhost', 'root', 'password', 'mydb'); // 检查连接是否成功 if (!$conn) { die('数据库连接失败:' . mysqli_connect_error()); } // 插入数据 $sql = "INSERT INTO users (name, email) VALUES ('$name', '$email')"; if (mysqli_query($conn, $sql)) { echo "数据已成功插入"; } else { echo "数据插入失败:" . mysqli_error($conn); } // 关闭连接 mysqli_close($conn);
This example assumes that we already have experience using a MySQL database. If you are using another type of database, you will need to change the connection information and SQL statements accordingly.
- Data Validation and Security
When dealing with form data, we need to ensure that the information contained in it is valid and secure. Server-side validation is an important way to achieve this. In addition, we should also pay attention to the following aspects:
- For required fields, you need to check whether they have values.
- For numeric fields, it is necessary to determine whether they belong to a certain interval.
- For other fields, you need to check whether their length, format, type and other information meet the requirements.
- For fields containing sensitive information, they need to be securely encoded to avoid SQL injection and XSS attacks.
- Summary
This article introduces the basic steps of PHP form processing. To process form data, we first need to write an HTML form and submit the data using the POST or GET method. On the server side, we use PHP's built-in functions and arrays to validate and filter the form data. Finally, we can store the form data into the database and perform other processing on it.
To ensure the security and validity of form data, we need to verify and filter it to prevent security issues such as SQL injection and XSS attacks. By mastering the basic knowledge of PHP form processing, we can write more secure, reliable, and easy-to-use web applications.
The above is the detailed content of Getting Started with PHP: Form Processing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


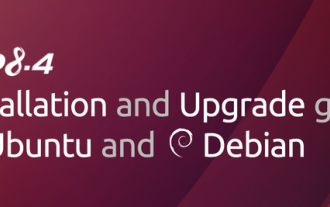
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
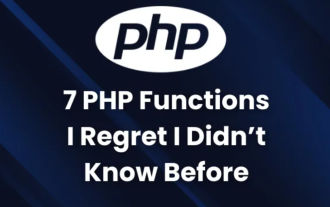
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
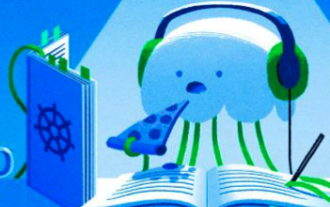
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
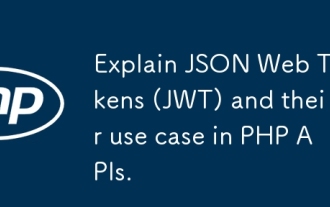
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
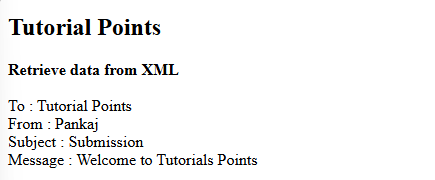
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
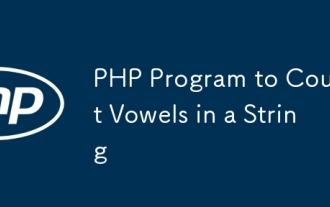
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
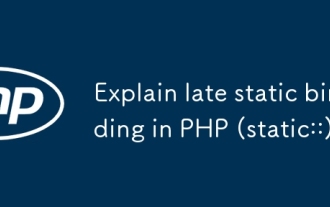
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
