How to define functions in PHP
Defining functions in PHP allows us to better organize code, reuse code, and improve code readability and maintainability. Here are some steps and considerations to help you define functions in PHP.
- Function name:
The function name is the key to defining the function. It should usually be concise, clear and easy to understand, and can reflect the function and role of the function. In PHP language, the function name should be a string starting with a letter or underscore. It is recommended to use camel case naming method (CamelCase) or underscore naming method (snake_case). - Function parameters:
Function parameters refer to the values passed into the function and are used to perform specific operations in the function. Parameters can be defined or not defined in PHP. Example:
1 2 3 |
|
Use a parameter list enclosed in parentheses after the function name, and separate multiple parameters with commas. When defining a function, you can specify default values for parameters.
1 2 3 |
|
When defining a function, we can declare what types of parameters we expect.
1 2 3 |
|
- Function return value:
The function return value refers to the value returned after the function is executed. In PHP, you can define a return value or not. Example:
1 2 3 |
|
In the function body, use the return statement to return the result of the function, which can be a value of any data type. When defining a function, we can declare what data types the function expects to return.
1 2 3 |
|
- Function scope:
Function scope refers to the access scope of variables defined in the function. In PHP, variables and parameters defined within a function are local variables by default and can only be accessed within the function. If we want to access internal variables outside the function, we need to use the global or static keywords. Example:
1 2 3 4 5 6 7 8 9 10 |
|
Use global inside a function to access global variables outside the function, and use static inside a function to define static variables.
- Function call:
After defining the function, we can call the function where needed. In PHP, functions can be called directly by name, and the parameters required by the function need to be passed in when calling. Example:
1 |
|
The above are some steps and precautions that need to be paid attention to when defining functions in PHP. Defining functions can make our code clearer, easier to read, and easier to maintain, improves code reuse, and allows us to complete code writing faster.
The above is the detailed content of How to define functions in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


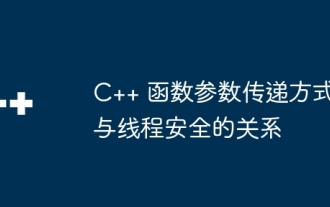
Function parameter passing methods and thread safety: Value passing: Create a copy of the parameter without affecting the original value, which is usually thread safe. Pass by reference: Passing the address, allowing modification of the original value, usually not thread-safe. Pointer passing: Passing a pointer to an address is similar to passing by reference and is usually not thread-safe. In multi-threaded programs, reference and pointer passing should be used with caution, and measures should be taken to prevent data races.
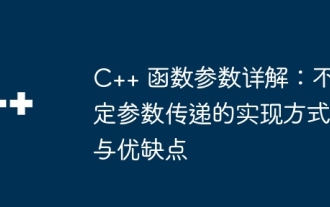
C++ indefinite parameter passing: implemented through the... operator, which accepts any number of additional parameters. The advantages include flexibility, scalability, and simplified code. The disadvantages include performance overhead, debugging difficulties, and type safety. Common practical examples include printf() and std::cout, which use va_list to handle a variable number of parameters.
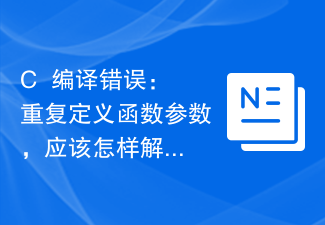
As an efficient programming language, C++ is widely used in various fields because of its reliability. However, in the process of writing code, we often encounter some compilation errors, and repeated definition of function parameters is one of them. This article will detail the reasons and solutions for repeatedly defining function parameters. What is repeatedly defining function parameters? In C++ programming, function parameters refer to variables or expressions that appear in function definitions and declarations and are used to accept actual parameters passed when a function is called. When defining a function's argument list, each argument must be
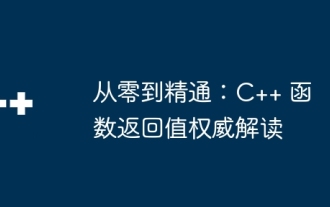
The return value type of a C++ function specifies the return value type after the function call, located after the function name and before the parentheses. The return value expression is an expression used to calculate and return the function value in the function body, usually placed in the return statement. Functions can also throw exceptions, and the type of exception thrown needs to be specified in the function declaration. Best practices for using function return values include choosing an appropriate return type, clearly specifying the return type, setting exception types, and using explicit return statements.
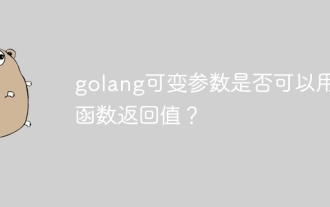
In Go language, variable parameters cannot be used as function return values because the return value of the function must be of a fixed type. Variadics are of unspecified type and therefore cannot be used as return values.
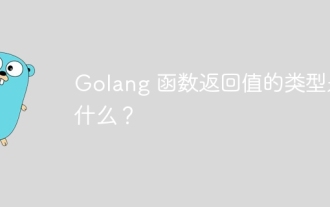
Go functions can return multiple values of different types. The return value type is specified in the function signature and returned through the return statement. For example, a function can return an integer and a string: funcgetDetails()(int,string). In practice, a function that calculates the area of a circle can return the area and an optional error: funccircleArea(radiusfloat64)(float64,error). Note: If the function signature does not specify a type, a null value is returned; it is recommended to use a return statement with an explicit type declaration to improve readability.
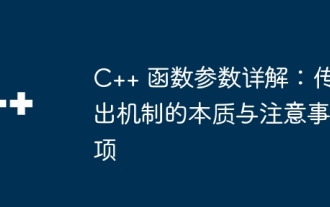
There are two ways to pass function parameters in C++: call by value (which does not affect the actual parameters) and call by reference (which affects the actual parameters). Passing out parameters is achieved by passing a reference or pointer, and the function can pass the value to the caller by modifying the variable pointed to by the parameter reference or pointer. Please note when using: The outgoing parameters must be clearly declared, can only correspond to one actual parameter, and cannot point to local variables within the function. When calling by passing a pointer, be careful to avoid wild pointers.
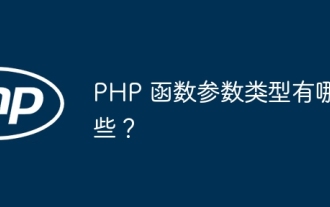
PHP function parameter types include scalar types (integers, floating point numbers, strings, Boolean values, null values), composite types (arrays, objects) and special types (callback functions, variable parameters). Functions can automatically convert parameters of different types, but they can also force specific types through type declarations to prevent accidental conversions and ensure parameter correctness.
