How to execute laravel queue all the time
In development using Laravel, queues are often an essential part. It can greatly improve the concurrent processing capabilities of applications, allowing a large number of tasks to be effectively managed and processed. However, in actual development, we often encounter such a problem: the queue is unstable, the queue suddenly stops when there are many tasks, or the queue cannot automatically restart when an exception occurs in the task. At this time we need some skills to enable the queue to run stably. Here are several methods to help you solve these problems.
1. Supervisor daemon process
Supervisor is a process control system written in Python. It is a common tool for managing processes on Linux servers. It can help us keep running in the background. Monitor a process. If the process exits for some reason, it can be restarted with the host process. This ensures that your queue can always run.
Install supervisor
Centos system execution
yum install -y supervisor
Ubuntu system execution
apt-get install -y supervisor
Open the configuration file
vim /etc/supervisord.conf
Add our laravel-queue configuration section
[program:laravel-queue]
command=php /path/to/artisan queue:work redis --sleep=3 --tries=3
directory=/path/to/your/project
autostart=true
autorestart=true
user=www-data
Description:
- program: Define the name of the process
- command: Define the running command
- autostart: Auto-start at boot
- autorestart: Automatic restart
- user: The user running the process
Save the modification and load the configuration
supervisorctl reload
Start the queue
supervisorctl start laravel-queue
This way you can implement the daemon process for the queue. If the queue stops, the process will automatically restart to ensure that the queue is always running. Of course, you can also run multiple work processes through configuration in the configuration file to improve queue processing capabilities.
2. Use Hashicorp’s Nomad to integrate Marathon
Hashicorp’s Nomad operates similarly to Marathon. They are both open source tools for distributed task orchestration. Nomad is able to orchestrate a set of different types of tasks by abstracting their location and decisions. Compared with Marathon, Nomad has the advantages of lightweight, high user experience, and easy configuration.
Using Nomad requires a docker environment. If you have not installed docker, you can install it through apt-get first:
apt-get install -y docker.io
- Create a Nomad Job
Create the job.nomad file in the project root directory and create a Nomad Job through configuration:
job "laravel_app" {
datacenters = ["dc1" ]
type = "service"
group "app" {
task "laravel-queue" { driver = "docker" config { image = "your_docker_image" command = ["php", "artisan", "queue:work", "--tries", "1", "--timeout", "30"] args = [ "--queue=critical,high,default,email,chat,sms", "--sleep=3" ] ports = ["http"] } resources { cpu = 500 memory = 128 network { mbits = 10 port "http" {} } } service { name = "laravel-queue" port = "http" check { type = "http" path = "/status" interval = "10s" timeout = "2s" } } restart { attempts = 10 interval = "5m" delay = "25s" mode = "failures" } }
}
}
The above configuration file uses docker in stand-alone mode Run it and modify it according to the actual situation in the production environment.
- Start Nomad Job
Execute the following command to start Nomad Job:
nomad run job.nomad
That’s it. The start of the queue. Nomad will run our job on a node and can monitor the job at the same time; when a problem occurs with the task, Nomad can quickly restart the task and update the status. Nomad Job can automatically migrate between different nodes in the cluster, which can reduce the load on a certain node and improve the robustness of the overall application.
3. Using AWS SQS
AWS's SQS (Simple Queue Service) is a service for message queues, which can efficiently deliver and process messages between distributed applications. , and allows horizontal scaling of message processing capabilities between applications. In the Laravel framework, SQS can be easily integrated into our applications. We only need to install the Laravel Queue package that supports SQS. At the same time, in AWS SQS, each queue has a minimum life cycle (TTL). If it is not successfully executed within this time period, the task will be deleted by SQS. This ensures that a task will eventually be executed successfully.
Installing SQS
Integrating SQS in Laravel is relatively simple. You only need to install the Laravel Queue package that supports SQS. It can be installed directly through Composer:
composer require "aws/aws-sdk-php ^3.0"
Configure SQS
Add the following content to the .env configuration file:
QUEUE_DRIVER=sqs
AWS_ACCESS_KEY_ID=your_aws_access_key
AWS_SECRET_ACCESS_KEY=your_aws_secret_key
AWS_DEFAULT_REGION=us-west-2
SQS_PREFIX=your_queue_prefix
Replace your_aws_access_key, your_aws_secre t_key and your_queue_prefix for you my own.
Start queue
php artisan queue:work sqs --queue=default --tries=3 --sleep=3 --timeout=60
The above command will start An SQS queue, and uses the default default queue, where the --tries parameter indicates the number of error attempts for the queue task, --sleep indicates the rest time after the queue processes the task, and --timeout indicates the automatic retry time when the queue task times out. The queue will continuously loop to obtain tasks and execute them:
When an error occurs, the queue will automatically retry until the number of error attempts of the queue task is exhausted or the execution is successful.
Summarize
In this article, we introduce several methods to keep the Laravel queue executing. Through daemon processes, task orchestration, third-party services, etc., we can provide more stable and efficient operation support for the queue. Each method has its own advantages and scope of application. Which method to choose depends on your actual situation.
The above is the detailed content of How to execute laravel queue all the time. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
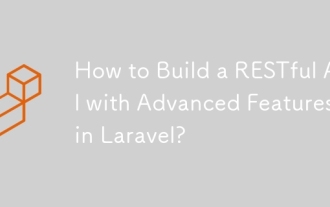
This article guides building robust Laravel RESTful APIs. It covers project setup, resource management, database interactions, serialization, authentication, authorization, testing, and crucial security best practices. Addressing scalability chall
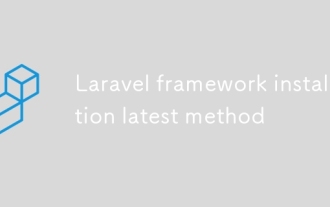
This article provides a comprehensive guide to installing the latest Laravel framework using Composer. It details prerequisites, step-by-step instructions, troubleshooting common installation issues (PHP version, extensions, permissions), and minimu
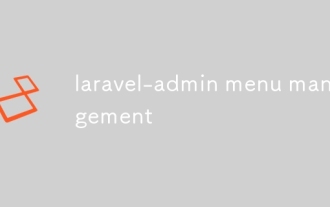
This article guides Laravel-Admin users on menu management. It covers menu customization, best practices for large menus (categorization, modularization, search), and dynamic menu generation based on user roles and permissions using Laravel's author
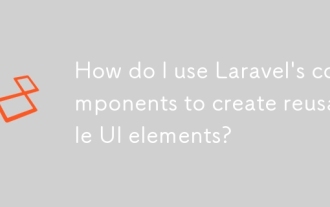
The article discusses creating and customizing reusable UI elements in Laravel using components, offering best practices for organization and suggesting enhancing packages.
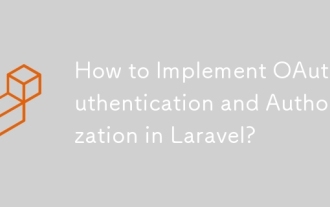
This article details implementing OAuth 2.0 authentication and authorization in Laravel. It covers using packages like league/oauth2-server or provider-specific solutions, emphasizing database setup, client registration, authorization server configu

This article guides Laravel developers in choosing the right version. It emphasizes the importance of selecting the latest Long Term Support (LTS) release for stability and security, while acknowledging that newer versions offer advanced features.
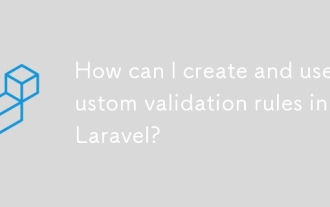
The article discusses creating and using custom validation rules in Laravel, offering steps to define and implement them. It highlights benefits like reusability and specificity, and provides methods to extend Laravel's validation system.
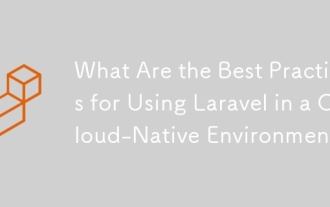
The article discusses best practices for deploying Laravel in cloud-native environments, focusing on scalability, reliability, and security. Key issues include containerization, microservices, stateless design, and optimization strategies.
