What are the knowledge points about Java reflection mechanism?
Class declaration cycle
java source code----->javac-------------->java bytecode file----- --------->java----------------->Class object (memory space: metaspace, local memory)------ ------------------new--------->Instantiated object----------------- -gc------------->Unload object
- Object.getClass() (memory stage)
- Test.class (metaspace)
- class.forName("Class full Name: Package name, class name"): You can get the object without entering the memory space (hard disk)
- Class.forName("Full name of the class"): mostly used in configuration files, define the class name in the configuration file, read the configuration file, and load the class
- Class name.class: mostly used for passing parameters
- Object name.getClass(): mostly used for object acquisition of class objects
package com.reflect; public class TestReflectPerson { public static void main(String[] args) throws ClassNotFoundException { //1.class.forName() Class class1=Class.forName("com.reflect.Person"); System.out.println(class1); //2.类名.class Class class2=Person.class; System.out.println(class2); //2.对象名.getClass() Class class3=new Person().getClass(); System.out.println(class3); System.out.println(class1==class2); //true System.out.println(class2==class3); //true } }
- Set value set (Object obj,Object value)
- Get the value get(Object obj)
//获得构造方法对象, Constructor cons1 = pcla.getDeclaredConstructor(String.class, int.class); Person p2 = (Person)cons1.newInstance("李四",19); System.out.println("p2:"+p2.getName());
package com.reflect; import java.lang.reflect.Constructor; import java.lang.reflect.Field; import java.lang.reflect.Method; public class TestReflectPerson { public static void main(String[] args) throws Exception { /* //1.class.forName() Class class1=Class.forName("com.reflect.Person"); System.out.println(class1); //2.类名.class Class class2=Person.class; System.out.println(class2); //2.类名.getClass() Class class3=new Person().getClass(); System.out.println(class3); System.out.println(class1==class2); System.out.println(class2==class3);*/ //获取对象 Class tclass=Class.forName("com.reflect.Person"); //通过类对象获取成员变量们 Field[] fields = tclass.getDeclaredFields(); System.out.println("获取Person对象的所有属性对象"); for (Field field:fields){ System.out.println(field); } //指定获取Person对象的属性对象 System.out.println("指定获取Person对象的属性对象"); Field age=tclass.getDeclaredField("age"); System.out.println("age:"+age); //通过类对象获取所有的构造方法 Constructor[] constructors = tclass.getDeclaredConstructors(); System.out.println("获取Person的所有构造方法对象"); for (Constructor constructor:constructors){ System.out.println(constructor); } //通过类对象获取无参的构造方法 Constructor constructor = tclass.getDeclaredConstructor(); System.out.println("constructor:"+constructor); //通过类对象获取有参的构造方法 Constructor constructor1 = tclass.getDeclaredConstructor(String.class,int.class); System.out.println("constructor1:"+constructor1); //通过类对象获取所有的成员方法 Method[] methods = tclass.getDeclaredMethods(); for (Method method:methods){ System.out.println("method:"+method); } //通过类对象获取getAge成员方法 Method getAge = tclass.getDeclaredMethod("getAge"); System.out.println("getAge:"+getAge); //通过类对象获取getAge成员方法 Method setAge = tclass.getDeclaredMethod("setAge", int.class); System.out.println("setAge:"+setAge); } }
package com.reflect; import java.lang.reflect.Field; public class TestField { public static void main(String[] args) throws Exception { Class pcla=Person.class; /*//获取公共访问权限的成员变量 Field[] fields = pcla.getFields(); for (Field field:fields){ System.out.println("getFild:"+field); } System.out.println(); //获取所有访问权限的成员变量 Field[] fielddes = pcla.getDeclaredFields(); for (Field field:fielddes){ System.out.println("field:"+field); }*/ Field name = pcla.getDeclaredField("name"); System.out.println(name); Person person=new Person(); //暴力反射:获取任意访问权限修饰符的安全检查 name.setAccessible(true); //获取公共成员变量的值 Object value = name.get(person); System.out.println(value); //获取任意访问权限的成员变量的值 Object value2 = name.get(person); System.out.println("value2:"+value2); //设置任意访问权限的成员变量的值 name.set(person,"张三"); Object value3=name.get(person); System.out.println("name:"+value3); } }
//暴力反射:获取任意访问权限修饰符的安全检查
name.setAccessible(true);
Copy after loginJudge processes and threads based on whether there is a main method
Process: It has its own main method and can be started by relying on its own main method. It is called a process
Thread: It does not have its own main method and needs to rely on Other tools to runFor example: servlet needs to be run with the help of tomcate. Tomcate has its own main methodThe background of reflection (remember)For example: in When the servlet is run with the help of the tool tomcate, tomacate cannot access the resources of the class when running the project, which results in reflection Why tomcate cannot get the new objectDetailed explanation: tomcate is impossible Called through new, because tomacate is generated and written first, and the class is written later, so tomcate does not know what the object of new is. You can obtain the file path through package scanning, but you cannot use new in this way. way, resulting in reflection. ate has its own main methodThe background of reflectionExample: When the servlet is run by using the tool tomacate, tomacate cannot access the class when running the project resources, resulting in reflectionWhy can’t tomcate get the new object? Detailed explanation: Tomcate cannot be called through new, because tomacate is generated and written first, and the class is written later, so tomcate does not know what the object of new is. It can be called through package scanning. to obtain the file path, but this method cannot use new, which results in reflection. When tomcate wants to call the doGet and doPost methods, because these two methods are not static, they must be called through the new object, but tomcate cannot create objects, so reflection is generated to obtain the file//暴力反射:获取任意访问权限修饰符的安全检查 name.setAccessible(true);
The above is the detailed content of What are the knowledge points about Java reflection mechanism?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




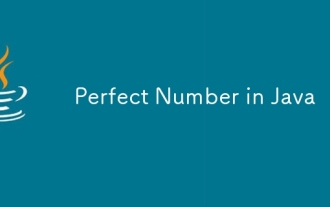
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
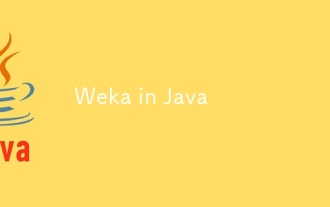
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
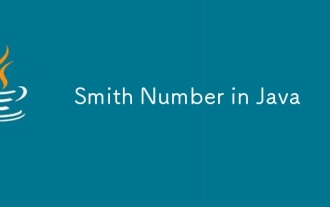
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
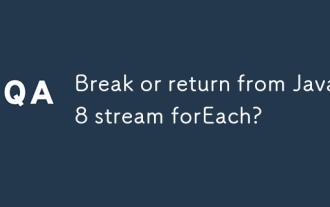
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
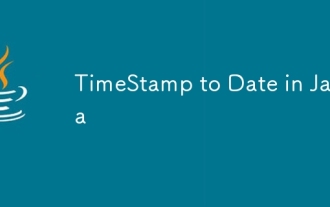
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
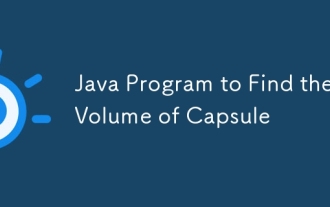
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
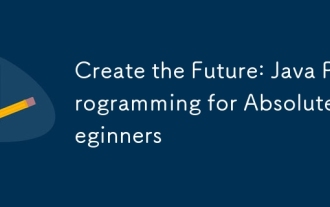
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
