golang api build
Golang is a programming language specifically designed for building high-concurrency services and network applications. Golang has efficient concurrency and network programming capabilities, which makes it the first choice of many developers. To build a Golang API, we need to master many basic concepts and skills, from routing processing to database access and more. This article will introduce how to use Golang to build APIs.
1. Environment preparation
Before you start building the Golang API, you need to install Golang on your computer. You can download the latest version of Golang through the Golang official website (https://golang.org/), or install it through a package manager, such as apt-get or yum, etc. After the installation is complete, enter "go version" in the terminal to verify whether the installation was successful.
2. Dependency package installation
Use the "go get" command in the terminal to install the required dependency packages. Dependent packages are some libraries we often use in the API, such as "gorilla/mux" and "gorm". The installation method is as follows:
go get github.com/gorilla/mux go get github.com/jinzhu/gorm go get github.com/go-sql-driver/mysql
3. Routing processing
All API requests need to be completed through routing. One of the most popular routing packages in Golang is gorilla/mux, so we will use that as well.
In order to use gorilla/mux, we need to import the package and create a new mux object. In this example, we will create two routes, one to display the API homepage and another to handle our GET requests.
package main import ( "fmt" "net/http" "github.com/gorilla/mux" ) func main() { router := mux.NewRouter() router.HandleFunc("/", Index) router.HandleFunc("/tasks", getTasks).Methods("GET") http.ListenAndServe(":8000", router) } func Index(w http.ResponseWriter, r *http.Request) { fmt.Fprintln(w, "Welcome to my API!") } func getTasks(w http.ResponseWriter, r *http.Request) { fmt.Fprintln(w, "You have requested all tasks") }
In the above code, we created two processing functions, Index and getTasks. The Index function is used to display the API homepage, and getTasks handles all GET requests.
4. Database access
Now we will add database functionality to the API. In this example, we will use a MySQL database and use the gorm library to manage the data.
First, we need to create a database connection. To do this, we need to create a Database struct that contains the connection details. Next, we will create a new "openDB" function to open the connection.
... import ( "database/sql" "log" _ "github.com/go-sql-driver/mysql" "github.com/jinzhu/gorm" ) type Database struct { *gorm.DB } func (db *Database) openDB() { dsn := "username:password@tcp(127.0.0.1:3306)/godb?charset=utf8&parseTime=True" conn, err := sql.Open("mysql", dsn) if err != nil { log.Fatal(err) } db.DB = gorm.Open("mysql", conn) }
We now need to create a new Database object in the main function and open the connection using the openDB function.
db := new(Database) db.openDB()
Next, we will create a Task Model to associate with our task list. A Model is just a regular Go struct that will be mapped to a table in our MySQL database.
type Task struct { Id int `gorm:"primary_key;auto_increment"` Name string `gorm:"not null"` Content string `gorm:"not null"` }
In order to create a new task, we will create a handler function called "createTask". This function will receive JSON data from the HTTP request, parse it, and insert it into our database.
func createTask(w http.ResponseWriter, r *http.Request) { db := new(Database) db.openDB() task := &Task{} task.Name = r.FormValue("name") task.Content = r.FormValue("content") db.Create(&task) fmt.Fprintln(w, "Task successfully created") }
We see that the "createTask" function creates a new Database object and Task object at the beginning. It then parses the JSON data from the HTTP request and inserts it into the database by calling the db.Create() method.
Finally, we will update our route "router.HandleFunc" to include the new route.
router.HandleFunc("/tasks", createTask).Methods("POST")
5. Middleware
Middleware is a common technology used to perform operations before or after processing a request. They are widely used not only in Golang but also in other languages. Let’s see how to use middleware to add some additional functionality in Golang API.
In Golang, middleware is a function that takes an HTTP processing function as a parameter. They allow some logic to be executed before or after the request is processed. In the following example, we will create a middleware called "withLogging" that will log the details of all API requests.
func withLogging(next http.Handler) http.Handler { return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { log.Println(r.Method, r.URL.Path) next.ServeHTTP(w, r) }) }
We see that a new http.Handler function is returned in the function, which records the requested method and URL path. The next.ServeHTTP(w, r) statement calls the next handler, ensuring that the handler continues to process API requests.
Next, we'll update our routes and handlers to include the new logging middleware.
router := mux.NewRouter() router.Use(withLogging) router.HandleFunc("/", Index) router.HandleFunc("/tasks", createTask).Methods("POST") router.HandleFunc("/tasks", getTasks).Methods("GET")
Now, add the "router.Use(withLogging)" statement before the route, and we will execute the withLogging middleware on all routing requests.
6. Summary
In this article, we introduced how to use gorilla/mux to handle routing in Golang, use gorm to manage MySQL database, and how to use middleware to enhance our API. By understanding these basic concepts and skills, we can start building more complex Golang APIs.
The above is the detailed content of golang api build. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


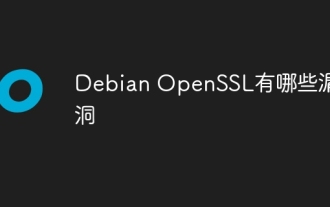
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
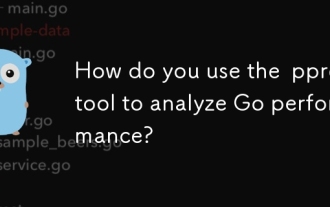
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
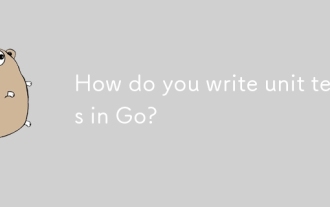
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
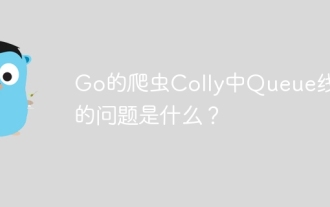
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
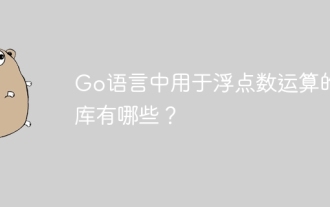
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
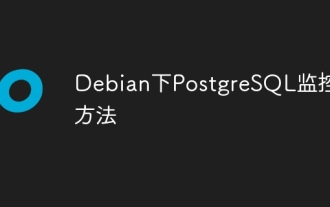
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
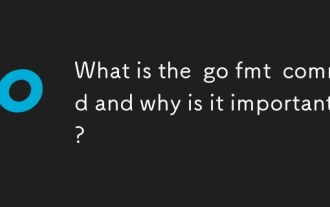
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
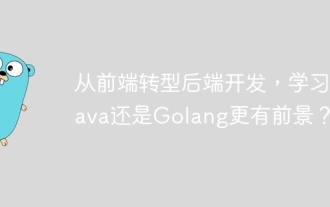
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
