golang http implementation
Golang is a fast, simple, efficient and safe programming language that is ideal for building distributed systems and network programming. In Golang, a built-in http package is provided for building web applications based on HTTP protocol. This article will introduce how to use Golang's http package to implement a simple web server.
Basic knowledge
Before using the http package, you need to understand some basic concepts and terminology. Here are some important concepts:
- HTTP Protocol: HTTP is a protocol used to transfer data between clients and servers. It can use different methods (GET, POST, PUT, DELETE, etc.) to send requests and receive responses.
- HTTP request: An HTTP request consists of a request line, request headers and request body. The request line contains information such as method, URI and protocol version, the request header contains some custom or standard header information, and the request body contains the request data.
- HTTP response: An HTTP response consists of a response line, response headers, and response body. The response line contains status code and status description, the response header contains some custom or standard header information, and the response body contains response data.
Implementation steps
Now, let’s take a look at how to use Golang’s http package to implement a simple web server. The following are the implementation steps:
- Import http package
Before starting, you need to import the http package at the beginning of the code file:
import "net/http"
- Create a processor function
In Golang, a processor function is a function that accepts http.ResponseWriter and *http.Request parameters. Its role is to process requests and return responses to the client. The following is an example of a handler function:
func handlerFunc(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello World!") }
This function accepts an http.ResponseWriter parameter and an http.Request parameter. http.ResponseWriter is used to send a response to the client, http.Request contains the request information sent by the client.
The function of this processor function is to send a string "Hello World!" to the client.
- Create a router
In Golang's http package, a router is used to dispatch requests to different processor functions. The method to create a router is:
router := http.NewServeMux()
Note that this method returns an object of type *http.ServeMux, which is used to store the mapping relationship between routing rules and processor functions.
- Create routing rules
When creating routing rules, you need to use the router's HandleFunc() method, which accepts two parameters: path and processor function. The following is an example of a routing rule:
router.HandleFunc("/", handlerFunc)
The function of this routing rule is to map the root path "/" to the handler function handlerFunc. When the client requests the root path, the server will call handlerFunc to process the request and return response.
- Start the server
The last step is to start the server, listen for client connections and process requests. Use the ListenAndServe() method in Golang to start the server. This method accepts two parameters: server address and router. The following is an example of starting a server:
http.ListenAndServe(":8080", router)
This server will listen on the local port 8080 and use the router to handle client requests.
Complete code
The following is a sample code for a complete web server:
package main import ( "fmt" "net/http" ) func handlerFunc(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello World!") } func main() { router := http.NewServeMux() router.HandleFunc("/", handlerFunc) fmt.Println("Server started on http://localhost:8080") http.ListenAndServe(":8080", router) }
Test
In the directory where the code file is located, use the following command Start the server:
go run main.go
Then, visit http://localhost:8080 in the browser, and you will see the "Hello World!" string, indicating that the server has been successfully started and can handle the request.
Summary
This article introduces how to use Golang’s http package to implement a simple web server. This server can listen for client requests and return responses. Web applications based on the HTTP protocol can be easily built using the http package, which is one of the very practical features in Golang. I hope this article can help you learn more about Golang's http package and how to build a web server.
The above is the detailed content of golang http implementation. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
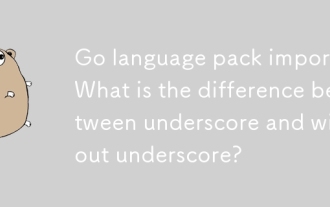
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t
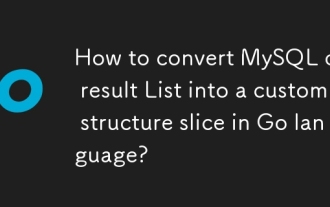
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
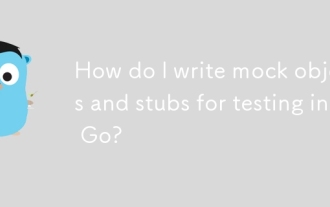
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
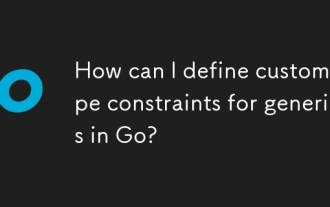
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
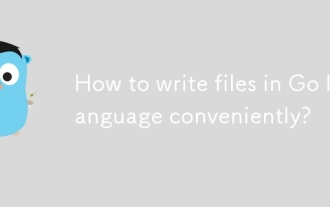
This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
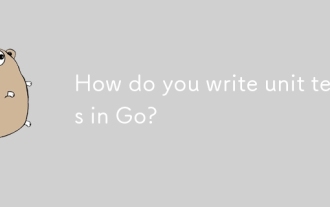
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
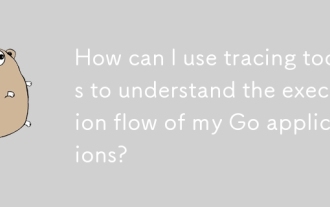
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
