golang query mysql
In modern software development, processing large amounts of data is a very common task. Storing and querying this data in MySQL, one of the most common databases, is one of the essential skills for framework developers. In this article, we will explore how to query MySQL using Golang, a programming language developed by Google, and how to use open source third-party libraries to connect, query, and manage data.
Why use Golang to query MySQL?
Golang is a fast, reliable and scalable open source programming language that is widely used in building high-performance networking and concurrent applications. Due to its efficient memory management and static type checking, Golang is suitable for handling large amounts of concurrent reads and writes, computationally intensive operations, and large-scale data processing tasks.
MySQL is a relational database that is often used to store large-scale data. Its advantages include flexible data structure, good data integrity and high scalability. It not only supports standard SQL language, but also supports numerous data query and connection methods.
Therefore, combining Golang with MySQL will produce the advantages of both parties, and is a very good choice for modern applications such as data storage and query. Golang also has many third-party libraries to choose from, which can help connect, query and manage the MySQL database, making it more convenient to query the MySQL database. Next, we will explain how to use the third-party library-Go-MySQL-Driver to connect to the MySQL database.
Query MySQL using Go-MySQL-Driver
Go-MySQL-Driver is a popular open source library used in Golang to connect and operate MySQL. It provides a simple API to easily connect to a MySQL instance and perform efficient data query operations. These operations include selecting, inserting, deleting and updating existing data, etc. The following will introduce in detail how to use Go-MySQL-Driver to connect and query the MySQL database.
Step 1: Install the Go-MySQL-Driver library
To start using Go-MySQL-Driver, you first need to install the Go-MySQL-Driver library in your Golang project. Install Go-MySQL-Driver in your project using the following command:
go get github.com/go-sql-driver/mysql
Step 2: Connect to MySQL server and get data
Once the Go-MySQL-Driver library is installed, you can use the following code segment to connect to the MySQL server. The following is a simple example:
package main import ( "database/sql" "fmt" _ "github.com/go-sql-driver/mysql" ) func main() { // 打开数据库连接,创建一个 db 变量 // 第一个参数:指定使用 mysql 协议来连接数据库 // 第二个参数:root 是 MySQL 的超级管理员账户名称 // 第三个参数:mypass 是 MySQL 的管理员账户密码 // 第四个参数:localhost 是 MySQL 数据库服务器的主机地址 // 第五个参数:3306 是 MySQL 数据库服务器的端口号 db, err := sql.Open("mysql", "root:mypass@tcp(localhost:3306)/") // 检查是否连接成功 if err != nil { panic(err.Error()) } // 关闭数据库连接 defer db.Close() // 定义一个查询语句并执行 rows, err := db.Query("SELECT name, age FROM users") if err != nil { panic(err.Error()) } defer rows.Close() // 打印查询结果 for rows.Next() { var name string var age int if err := rows.Scan(&name, &age); err != nil { panic(err.Error()) } fmt.Printf("Name: %s, Age: %d ", name, age) } }
In this example, we first use the sql.Open
function to open a connection to the MySQL database instance. db
The variable represents a connection handle, which stores other information that is needed for any operation performed in the MySQL database.
Once the connection is established, we use the db.Query
function to query the database. This function is used to execute SQL queries and returns a sql.Rows
structure. sql.Rows
is a result set used for iterative execution results.
In this example, we executed a very simple SQL query statement "SELECT name, age FROM users"
and used the .Scan
method to store the results in the corresponding variables and then iterate over the results and print them.
Step 3: Perform INSERT, UPDATE and DELETE operations
In addition to queries, Go-MySQL-Driver also provides APIs for performing INSERT, UPDATE and DELETE operations. The following is an example:
package main import ( "database/sql" "fmt" _ "github.com/go-sql-driver/mysql" ) func main() { db, err := sql.Open("mysql", "root:mypass@tcp(localhost:3306)/") if err != nil { panic(err.Error()) } // 关闭数据库连接 defer db.Close() // 准备一个语句,对数据表进行插入操作 stmt, err := db.Prepare("INSERT INTO users(name, age) VALUES(?, ?)") if err != nil { panic(err.Error()) } // 插入数据 res, err := stmt.Exec("Tom", 20) if err != nil { panic(err.Error()) } // 获取插入数据的上一条语句的 ID id, err := res.LastInsertId() if err != nil { panic(err.Error()) } fmt.Printf("Inserted record with ID %d ", id) }
This example demonstrates how to use db.Prepare
to create a prepared statement that will insert specific information during subsequent executions.
This example uses the ?
placeholder, as described above, rather than referencing the actual variable in the SQL statement. They will be replaced when stmt
is executed. This method helps avoid the possibility of SQL injection.
When stmt
is ready, we input the data into stmt
and insert it using the stmt.Exec()
method. The stmt.Exec()
method returns the result sql.Result
, which includes the number of rows affected and the ID of the last inserted row.
In this example, we use the res.LastInsertId()
method to retrieve the ID.
Conclusion
The above is a simple example of querying MySQL using Golang and Go-MySQL-Driver. It can be predicted that more tasks can be done by combining Golang and MySQL. For example, using this combination in abstract multi-tier applications can efficiently handle a large number of data storage and query tasks.
Of course, Go-MySQL-Driver may not be the only way to connect to and operate MySQL. Golang has a wealth of third-party libraries, including SQLX, GORM, etc. This requires you to analyze your specific situation and choose the library that is most suitable for your use.
Finally, I hope you can integrate the information provided in this article into your next MySQL project and unleash the power of Golang and MySQL.
The above is the detailed content of golang query mysql. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


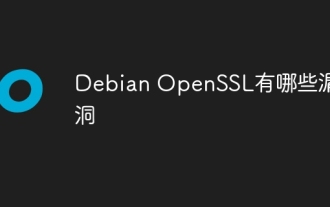
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
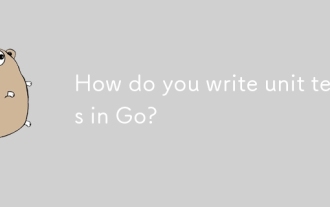
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
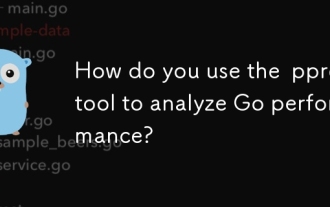
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
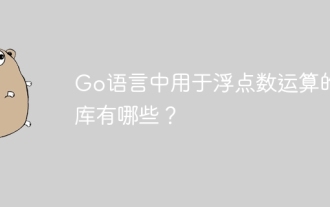
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
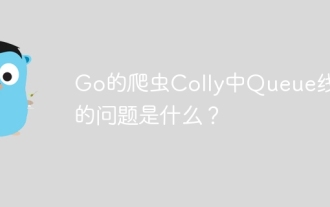
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
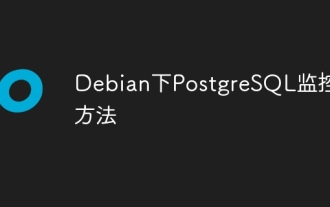
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
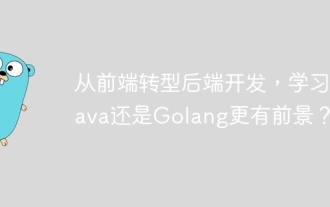
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
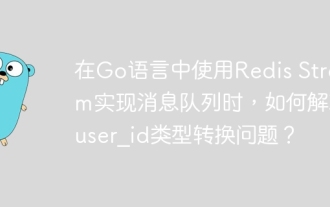
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
