


Example analysis of Java Fluent Mybatis aggregation query and apply method process
Data preparation
In order to aggregate the query conditions, several pieces of data have been added.
MIN
We try to get the minimum age.
Method implementation
@Override public Integer getAgeMin() { Map<String, Object> result = testFluentMybatisMapper .findOneMap(new TestFluentMybatisQuery().select.min.age("minAge").end()) .orElse(null); return result != null ? Convert.toInt(result.get("minAge"), 0) : 0; }
Control layer code
@ApiOperation(value = "获取最小年龄", notes = "获取最小年龄") @RequestMapping(value = "/getAgeMin", method = RequestMethod.GET) @ResponseBody public Result<Integer> getAgeMin() { try { return Result.ok(aggregateService.getAgeMin()); } catch (Exception exception) { return Result.error(ErrorCode.BASE_ERROR_CODE.getCode(), exception.getMessage(), null); } }
Debug code
Code description:
1. Why does age("minAge") need to add a string? Is it okay not to add it? The answer is yes, but the results you see returned are like this.
Yes, what’s in the parentheses is the alias of the aggregate query result. If you don’t pass it, the result will be embarrassing. It’s recommended to pass it.
MAX
When doing the max aggregation function, I will make it a little more complicated and add group by.
Define the return entity.
import lombok.AllArgsConstructor; import lombok.Builder; import lombok.Data; import lombok.NoArgsConstructor; /** @Author huyi @Date 2021/10/26 14:15 @Description: 聚合最大年龄返回体 */ @Data @AllArgsConstructor @NoArgsConstructor @Builder public class AggregateMaxAgeRsp { private String name; private Integer maxAge; }
Method implementation
@Override public List<AggregateMaxAgeRsp> getAgeMaxByName() { List<Map<String, Object>> result = testFluentMybatisMapper.listMaps( new TestFluentMybatisQuery() .select .name() .max .age("maxAge") .end() .groupBy .name() .end()); if (result != null && result.size() != 0) { List<AggregateMaxAgeRsp> list = new ArrayList<>(); result.forEach( x -> list.add(BeanUtil.fillBeanWithMapIgnoreCase(x, new AggregateMaxAgeRsp(), false))); return list; } else { return null; } }
Control layer code
@ApiOperation(value = "根据年龄分组并获取最大年龄", notes = "根据年龄分组并获取最大年龄") @RequestMapping(value = "/getAgeMaxByName", method = RequestMethod.GET) @ResponseBody public Result<List<AggregateMaxAgeRsp>> getAgeMaxByName() { try { return Result.ok(aggregateService.getAgeMaxByName()); } catch (Exception exception) { return Result.error(ErrorCode.BASE_ERROR_CODE.getCode(), exception.getMessage(), null); } }
Debug code
import lombok.AllArgsConstructor; import lombok.Builder; import lombok.Data; import lombok.NoArgsConstructor; /** @Author huyi @Date 2021/10/26 14:50 @Description: 聚合平均总和返回体 */ @Data @AllArgsConstructor @NoArgsConstructor @Builder public class AggregateAgeSumAvgAndCountRsp { private String name; private Integer sum; private Integer avg; private Integer count; }
@Override public List<AggregateAgeSumAvgAndCountRsp> getAgeSumAvgCountByName() { List<Map<String, Object>> result = testFluentMybatisMapper.listMaps( new TestFluentMybatisQuery() .select .name() .sum .age("sum") .avg .age("avg") .count("count") .end() .groupBy .name() .end()); if (result != null && result.size() != 0) { List<AggregateAgeSumAvgAndCountRsp> list = new ArrayList<>(); result.forEach( x -> list.add( BeanUtil.fillBeanWithMapIgnoreCase( x, new AggregateAgeSumAvgAndCountRsp(), false))); return list; } else { return null; } }
@ApiOperation(value = "根据年龄分组并获取年龄和、平均年龄、数量", notes = "根据年龄分组并获取年龄和、平均年龄、数量") @RequestMapping(value = "/getAgeSumAvgCountByName", method = RequestMethod.GET) @ResponseBody public Result<List<AggregateAgeSumAvgAndCountRsp>> getAgeSumAvgCountByName() { try { return Result.ok(aggregateService.getAgeSumAvgCountByName()); } catch (Exception exception) { return Result.error(ErrorCode.BASE_ERROR_CODE.getCode(), exception.getMessage(), null); } }
import lombok.AllArgsConstructor; import lombok.Builder; import lombok.Data; import lombok.NoArgsConstructor; import java.util.Date; /** @Author huyi @Date 2021/10/26 15:10 @Description: 聚合应用返回体 */ @Data @AllArgsConstructor @NoArgsConstructor @Builder public class AggregateApplyRsp { private String name; private Date createTime; private Integer minAge; private Date maxTime; }
@Override public List<AggregateApplyRsp> getApply() { List<Map<String, Object>> result = testFluentMybatisMapper.listMaps( new TestFluentMybatisQuery() .select .apply("name") .createTime("createTime") .apply("min(age) as minAge", "max(create_time) as maxTime") .end() .groupBy .name() .createTime() .end()); if (result != null && result.size() != 0) { List<AggregateApplyRsp> list = new ArrayList<>(); result.forEach( x -> list.add(BeanUtil.fillBeanWithMapIgnoreCase(x, new AggregateApplyRsp(), false))); return list; } else { return null; } }
@ApiOperation(value = "根据名字获取最小年龄,使用语句", notes = "根据名字获取最小年龄,使用语句") @RequestMapping(value = "/getApply", method = RequestMethod.GET) @ResponseBody public Result<List<AggregateApplyRsp>> getApply() { try { return Result.ok(aggregateService.getApply()); } catch (Exception exception) { return Result.error(ErrorCode.BASE_ERROR_CODE.getCode(), exception.getMessage(), null); } }
The above is the detailed content of Example analysis of Java Fluent Mybatis aggregation query and apply method process. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


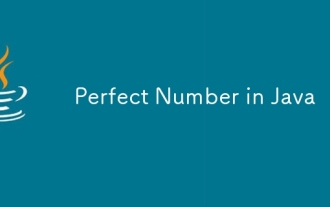
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
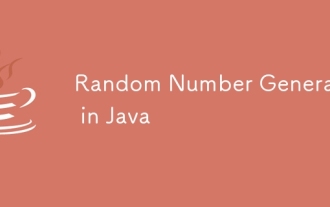
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
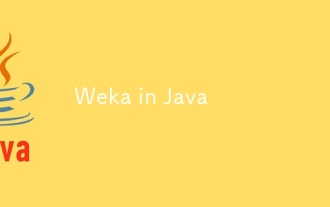
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
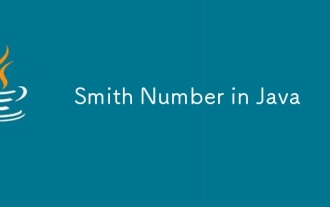
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
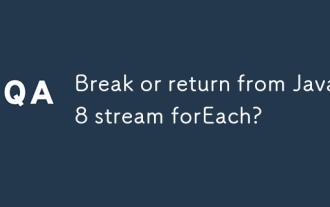
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
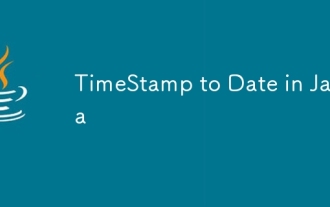
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
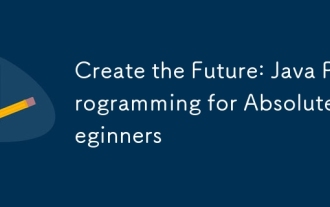
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
