golang delete request
With the rapid development of the Internet, Web applications are becoming more and more popular. With the rapid development of web applications and the Internet, more and more developers are turning their attention to using the Go programming language. As an efficient, simple and easy-to-learn programming language, Go is highly praised for its performance in the field of web application development. Among them, using golang to initiate delete requests has become one of the skills that Golang developers need to master.
1. What is a DELETE request?
DELETE request is one of the HTTP protocols. Like GET and POST requests, it is a way to communicate with the server. DELETE requests are generally used to delete a resource on the server, such as deleting data from a database. DELETE requests can pass parameters and data just like GET and POST requests.
Every HTTP request has a request method, and the DELETE request also has its own request method type: DELETE. DELETE requests can send data via the request body or request URL.
2. Use golang to initiate a DELETE request
The way to initiate a DELETE request using the Go language is very similar to using GET and POST requests. The following is the general form of writing a delete request:
client := new(http.Client) req, err := http.NewRequest("DELETE", "https://your-url-here.com/delete/resource", nil) // 为请求添加请求头部信息 req.Header.Add("Authorization", "Bearer "+TOKEN) req.Header.Set("Content-Type", "application/json") res, err := client.Do(req) ...
As can be seen from the above code, we use the http.NewRequest function to create a DELETE request. This function needs to pass three parameters: request method type, request URL and request body. Because DELETE requests usually do not require a request body, we can use nil to indicate that this is an empty request.
Once the request is created, we can add request header information to the request through the req.Header.Add function. In this example, we add an Authorization as a request header and pass a TOKEN as a parameter. In addition, we also set the Content-Type of the request to application/json.
After creating the request, we can use http.Client to send the request and receive the response. If the request is successful, the response will contain detailed information about the server's processing.
3. Use golang’s Gin framework to handle DELETE requests
Gin is a very popular web application framework based on the Go programming language. The Gin framework is very simple to use and very efficient at the same time. When using the Gin framework to handle DELETE requests, you need to use a gin.Context type instance to handle the request.
In the Gin framework, we can use the DELETE function to handle DELETE requests. The following is a simple DELETE request processing function written based on the Gin framework:
func handleDelete(c *gin.Context) { resourceName := c.Param("resource") // 基于resourceName执行对服务器上的资源的删除操作 ... c.JSON(http.StatusOK, gin.H{"message": "Resource deleted successfully"}) }
In the above code, we define a processing function named handleDelete. In this function, we use the c.Param("resource") statement to obtain the parameter (resource) value in the request URL. We can then perform operations that handle deleting the resource. At the end, we use the c.JSON function to respond to the client with a status code and a message.
4. Summary
In Go language programming, initiating a DELETE request is a very basic and common task. We can use the http library and the functions provided in the Gin framework to send DELETE requests and handle DELETE requests. Using the HTTP library and Gin framework, we can easily create reliable and efficient web applications, helping us better complete web application development work.
The above is the detailed content of golang delete request. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


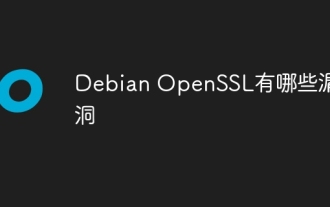
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
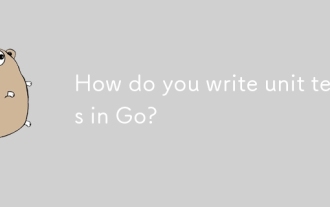
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
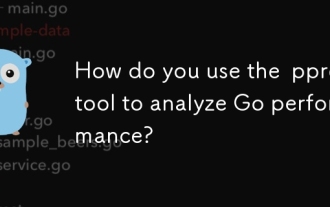
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
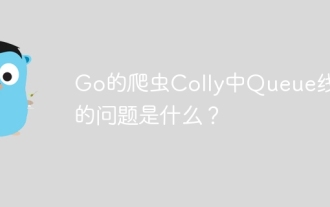
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
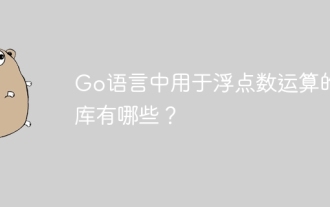
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
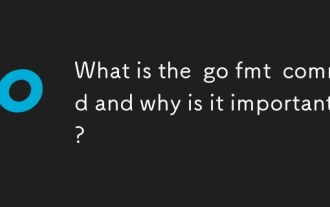
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
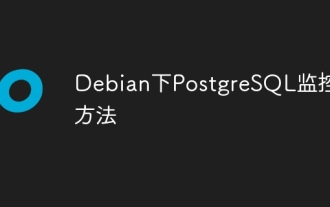
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
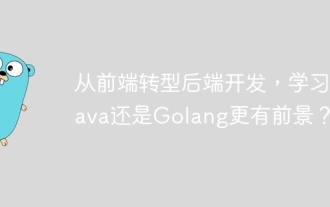
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
