String conversion dimensional array php
In PHP programming, we often need to convert strings to arrays. Sometimes, we need to split a string into multiple elements according to specific delimiters and then form an array. In this article, we will explain how to convert a string to an array using PHP functions.
- explode function
PHP’s explode function is the most common way to split a string into multiple substrings and store them in an array. This function accepts two parameters: delimiter and source string. For example:
$str = "apple,banana,orange"; $arr = explode(",", $str); print_r($arr);
Output result:
Array ( [0] => apple [1] => banana [2] => orange )
In the example, use comma as the separator. The explode function splits the string into three elements: apple, banana, and orange, and stores them into the array $arr.
- str_split function
PHP’s str_split function splits a string into individual characters and stores them into an array. This function accepts one parameter: the source string. For example:
$str = "hello"; $arr = str_split($str); print_r($arr);
Output result:
Array ( [0] => h [1] => e [2] => l [3] => l [4] => o )
In the example, the string hello is split into five elements: h, e, l, l and o, and they are stored in the array $arr.
- preg_split function
PHP’s preg_split function is similar to the explode function, but it uses regular expressions as delimiters. This function accepts two parameters: regular expression and source string. For example:
$str = "the quick brown fox jumps over the lazy dog"; $arr = preg_split("/s+/", $str); print_r($arr);
Output result:
Array ( [0] => the [1] => quick [2] => brown [3] => fox [4] => jumps [5] => over [6] => the [7] => lazy [8] => dog )
In the example, use regular expressions to split the string into multiple elements according to spaces and store them in the array $arr.
- sscanf function
PHP’s sscanf function extracts data from a string. This function accepts two parameters: source string and format string. The format string describes the format of the data to be extracted. For example:
$str = "John Doe,35"; sscanf($str, "%s,%d", $name, $age); echo "Name: $name, Age: $age";
Output:
Name: John Doe, Age: 35
In the example, the format string specifies two data items: a string and an integer. The sscanf function extracts these data from the source string and stores them in the variables $name and $age respectively.
- json_decode function
PHP’s json_decode function parses a JSON-formatted string into an array or object. This function accepts one parameter: JSON string. For example:
$str = '{"name":"John", "age":35}'; $arr = json_decode($str, true); print_r($arr);
Output result:
Array ( [name] => John [age] => 35 )
In the example, convert the JSON string to an array. The json_decode function parses it and stores it in the array $arr.
- unserialize function
PHP’s unserialize function converts a serialized string into a PHP variable or object. This function accepts one parameter: the serialized string. For example:
$str = 'a:2:{i:0;s:5:"apple";i:1;s:6:"orange";}'; $arr = unserialize($str); print_r($arr);
Output result:
Array ( [0] => apple [1] => orange )
In the example, convert the serialized string into an array. The unserialize function parses it and stores it in the array $arr.
Summary
PHP provides many functions to convert strings to arrays. It is important to choose the appropriate function according to your needs. Using these functions, we can quickly and easily convert strings to arrays to better complete programming tasks.
The above is the detailed content of String conversion dimensional array php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
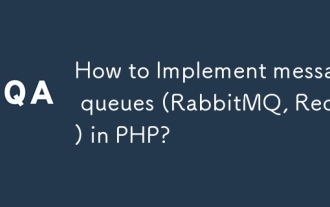
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
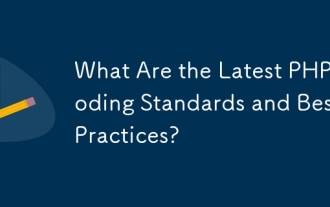
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
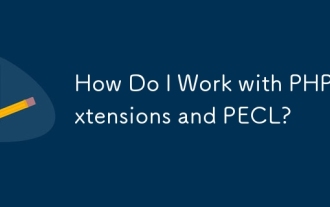
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
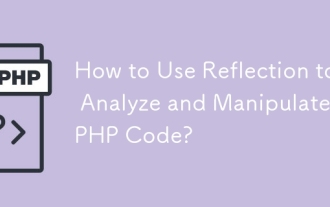
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
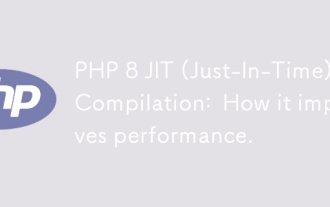
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
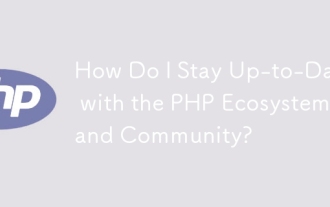
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
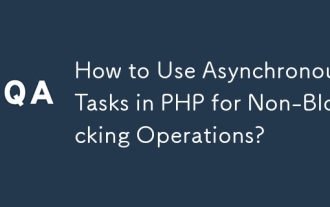
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
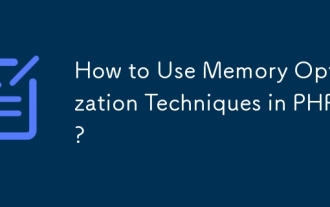
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v
