Convert php array to string array
PHP is a very popular programming language, especially widely used in the field of web development. During the development process, it is often necessary to convert PHP arrays into string arrays. Here we will introduce in detail how to implement this process.
PHP array is a very flexible data type that can accommodate various types of data, such as numbers, strings, Boolean values, etc. In PHP, arrays can use array names and indexes to access elements. Array indexes can be integers or strings, which allows arrays to be used in a variety of different scenarios.
Sometimes, we need to convert a PHP array into a string array. This conversion can make it easier for us to process strings in the program. To convert a PHP array into a string array, you need to go through the following steps:
- Create a PHP array
First you need to create a PHP array, which can be done through a variety of way to achieve. For example, you can use the array() function to create an empty array, or you can directly assign a value to an array element to create a non-empty array. The following is an example of creating a non-empty array:
$numbers = array(1, 2, 3, 4, 5);
- Use the implode() function
The implode() function in PHP can concatenate array elements into a string . The implode() function requires two parameters: the delimiter and the array to be concatenated. The following is an example of using the implode() function to convert an array into a string:
$numbers = array(1, 2, 3, 4, 5); $string_array = implode(',', $numbers);
Here we use commas as delimiters to convert the array into a comma-separated string.
- Used in combination with the explode() function
Sometimes, we need to split a string into an array according to a certain delimiter. At this point you can use the explode() function in PHP. The explode() function requires two parameters: the delimiter and the string to be split. The following is an example of using the explode() function to convert a comma-delimited string into an array:
$string_array = '1,2,3,4,5'; $numbers = explode(',', $string_array);
Here we use commas as delimiters to convert a string into an array.
- Convert an associative array into a string array
In addition to ordinary arrays, there is also a special array in PHP - an associative array. Associative arrays store data in the form of key-value pairs, and any non-empty string can be used as a key. When converting an associative array into a string array, pay attention to the relationship between keys and values. The following is an example of converting an associative array into a string array:
$person = array('name' => 'Tom', 'age' => 21, 'gender' => 'male'); $string_array = array(); foreach ($person as $key => $value) { $string_array[] = "$key: $value"; }
Here we first create an associative array $person, and then use a foreach loop to traverse the array and convert each key-value pair into a String and added to a string array $string_array.
Summary:
In this article, we introduced how to convert a PHP array into a string array, including creating a PHP array, using the implode() function, using it in combination with the explode() function, and Convert associative array to string array. These methods can easily perform string processing in PHP programs, bringing more convenience to web development.
The above is the detailed content of Convert php array to string array. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
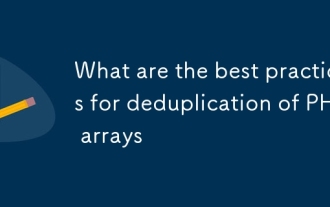
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
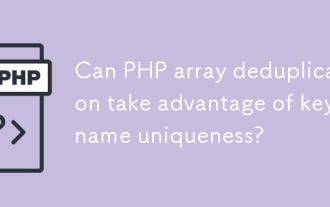
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
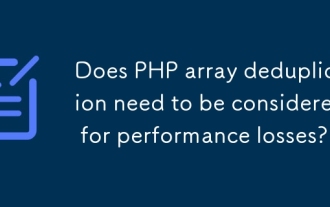
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
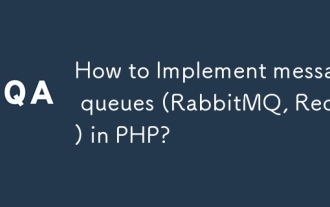
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
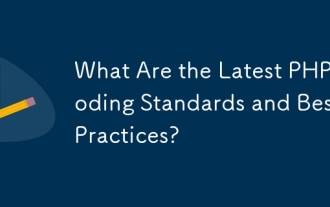
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
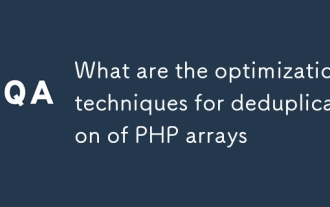
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
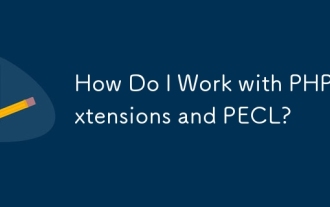
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
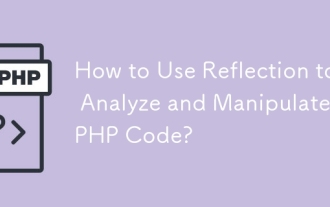
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
