PHP determines whether a string contains array elements
In PHP programming, it is often necessary to determine whether a string contains elements in an array. This kind of operation is very common in actual development, and is usually implemented through string functions and array functions.
- Using the string function strpos
The string function strpos in PHP can be used to determine whether a string contains another string. Returns the index of the containing position if included, false otherwise.
Sample code:
$str = 'hello world'; $needle = 'world'; if (strpos($str, $needle) !== false) { echo '包含'; } else { echo '不包含'; }
The output result of the above code is "includes".
With this method, we can use a foreach loop to iterate through each element in the array and then find the element in the string. But there is a problem with this method, that is, if there are the same elements in the array, it is impossible to tell where the element appears in the string.
- Using the regular expression preg_match
Another method is to use the regular expression function preg_match. This function is used to find content in a string that matches a specified pattern. You can use regular expressions to concatenate array elements into a matching pattern and then search for that pattern in a string.
Sample code:
$str = 'hello world'; $needle = array('world', 'foo'); $pattern = '/' . implode('|', $needle) . '/'; if (preg_match($pattern, $str, $matches)) { echo '包含匹配项:' . $matches[0]; } else { echo '不包含'; }
The output of the above code is "Contains matches: world".
- Use the array function in_array
The array function in_array in PHP can also be used to determine whether a string contains elements in an array. This function is used to check whether a value exists in the array and returns true if it exists, otherwise it returns false.
Sample code:
$str = 'hello world'; $needle = array('world', 'foo'); if (in_array($needle, $str)) { echo '包含'; } else { echo '不包含'; }
The output result of the above code is "not included".
Note: Pay attention to the parameter order of in_array and strpos functions, because their parameter order is different.
Conclusion
In actual development, the above three methods can be used to determine whether a string contains elements in an array. The performance and complexity of different methods vary, and the most appropriate method can be selected based on the specific situation. If you need to match multiple array elements, the regular expression preg_match is the most flexible method; if you only need to judge a single element, it will be simpler and more effective to use the string function strpos or the array function in_array.
The above is the detailed content of PHP determines whether a string contains array elements. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
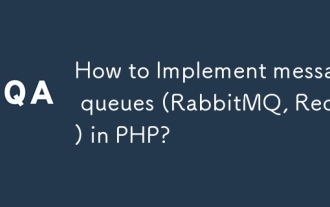
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
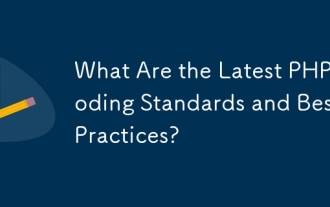
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
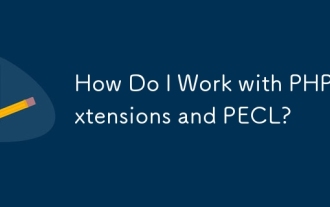
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
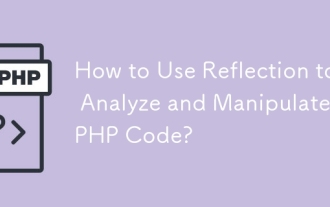
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
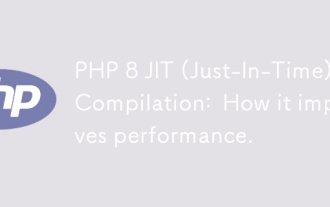
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
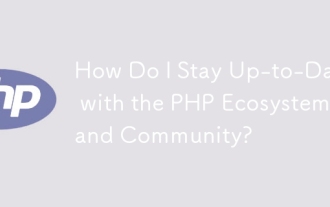
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
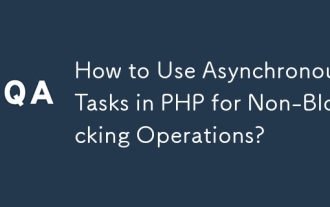
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
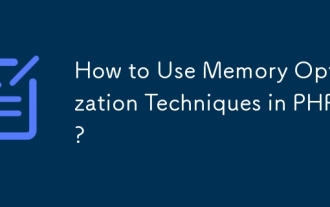
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v
