jquery date sets current date
In the development of web applications, it is often necessary to use date pickers, and jQuery is a very popular JavaScript library that also provides a wealth of date picker plug-ins. Before using the plug-in, we can also use jQuery's date operation method to set the current date. Next, this article will introduce how to use jQuery to set the current date.
1. Get the current date and time
In JavaScript, we can use the Date object to get the current date and time, and format the date as needed. In jQuery, you can also use the Date object to obtain the current date and time, and read and modify date and time values through jQuery's selector. The following is a code example for obtaining the current time and date:
//获取当前时间 var now = new Date(); var time = now.getTime();//获取值为从1970年1月1日到当前日期的毫秒数 var formattedTime = now.toLocaleTimeString();//格式化成本地时间格式,例如:"上午10:08:30" var formattedDate = now.toLocaleDateString();//格式化当前日期,例如:"2021/10/29" //获取当前年月日 var year = now.getFullYear();//当前年份,例如:2021 var month = now.getMonth()+1;//当前月份(注意:月份从0开始,因此需要加1),例如:10 var date = now.getDate();//当前日期,例如:29
The above code is the method for obtaining the current time and date. You can adjust it according to your needs.
2. Set the current date
On the basis of obtaining the current time and date, we can use jQuery's selector to obtain the corresponding date input box and set it to the current date. The following is a sample code:
<!DOCTYPE html> <html> <head> <title>设置当前日期</title> <meta charset="utf-8"/> <script src="http://cdn.staticfile.org/jquery/1.7.2/jquery.min.js"></script> <script type="text/javascript"> $(document).ready(function() { //获取当前日期 var now = new Date(); var year = now.getFullYear(); var month = now.getMonth()+1; var date = now.getDate(); var today = year + '-' + month + '-' + date; //设置日期输入框的值为当前日期 $('#date_input').val(today); }); </script> </head> <body> <label>请输入日期:</label> <input type="text" id="date_input"/> </body> </html>
In the above code, through the ready() method of jQuery, the current date is obtained after the page is loaded, and formatted into the form of 'YYYY-MM-DD', and Assign it to the calendar input box.
It should be noted that when setting the value of the date input box, you need to use jQuery's selector to obtain the corresponding input box. Only when the corresponding element is obtained can it be modified.
3. Summary
In this article, we introduced how to use jQuery to get the current date and how to assign it to the date input box. By studying this article, we can learn how to use jQuery to simplify our development work and how to quickly implement date operations.
In actual development, obtaining the current date may not be the most commonly used method. It is more based on business needs to obtain the specified date, and jQuery also provides a wealth of date plug-ins, which can be used to implement complex date operations. Therefore, when using jQuery, you must be familiar with the related methods and plug-ins of date operations, so that you can better cope with various needs.
The above is the detailed content of jquery date sets current date. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.

React is the preferred tool for building interactive front-end experiences. 1) React simplifies UI development through componentization and virtual DOM. 2) Components are divided into function components and class components. Function components are simpler and class components provide more life cycle methods. 3) The working principle of React relies on virtual DOM and reconciliation algorithm to improve performance. 4) State management uses useState or this.state, and life cycle methods such as componentDidMount are used for specific logic. 5) Basic usage includes creating components and managing state, and advanced usage involves custom hooks and performance optimization. 6) Common errors include improper status updates and performance issues, debugging skills include using ReactDevTools and Excellent
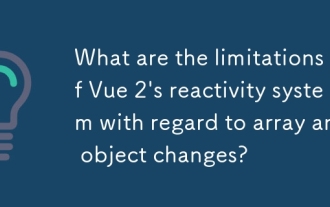
Vue 2's reactivity system struggles with direct array index setting, length modification, and object property addition/deletion. Developers can use Vue's mutation methods and Vue.set() to ensure reactivity.

React components can be defined by functions or classes, encapsulating UI logic and accepting input data through props. 1) Define components: Use functions or classes to return React elements. 2) Rendering component: React calls render method or executes function component. 3) Multiplexing components: pass data through props to build a complex UI. The lifecycle approach of components allows logic to be executed at different stages, improving development efficiency and code maintainability.
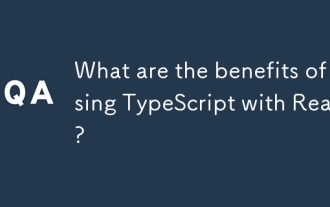
TypeScript enhances React development by providing type safety, improving code quality, and offering better IDE support, thus reducing errors and improving maintainability.
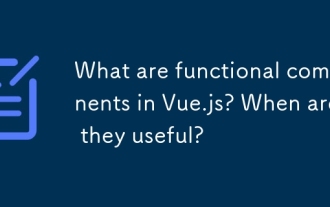
Functional components in Vue.js are stateless, lightweight, and lack lifecycle hooks, ideal for rendering pure data and optimizing performance. They differ from stateful components by not having state or reactivity, using render functions directly, a
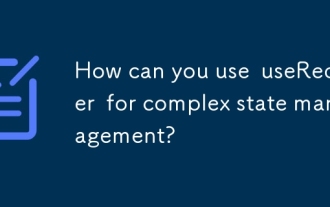
The article explains using useReducer for complex state management in React, detailing its benefits over useState and how to integrate it with useEffect for side effects.

React is a JavaScript library for building user interfaces, with its core components and state management. 1) Simplify UI development through componentization and state management. 2) The working principle includes reconciliation and rendering, and optimization can be implemented through React.memo and useMemo. 3) The basic usage is to create and render components, and the advanced usage includes using Hooks and ContextAPI. 4) Common errors such as improper status update, you can use ReactDevTools to debug. 5) Performance optimization includes using React.memo, virtualization lists and CodeSplitting, and keeping code readable and maintainable is best practice.
