How to interrupt code in nodejs
Node.js is a server-side JavaScript runtime environment based on event-driven, non-blocking I/O. Node.js has many advantages when dealing with highly concurrent, large-scale, real-time web applications. But when writing code, you may occasionally need to interrupt the running code. What should you do in this case?
In Node.js, you can use the following methods to interrupt code execution:
- Use the throw statement
In JavaScript, use the throw statement to throw an exception. In Node.js, all asynchronous operations are aborted when an exception is thrown. This is because all asynchronous functions in Node.js are callback-based, and when an exception is thrown, an error callback function is triggered, causing the program to end execution.
The following is a sample code:
try { // do something throw new Error('Custom error message'); } catch (err) { console.error(err); }
In the above code, use try-catch to wrap the code block that may throw an exception. When an exception is thrown, the catch code block will be entered and Output exception information.
- Using the process.exit() method
The process.exit() method can be used to immediately terminate the execution of the current Node.js process. When this method is called, the Node.js process terminates immediately and returns the specified exit code. If no exit code is specified, it defaults to 0.
The following is a sample code:
process.exit(1); // 1 为退出码
- Using the process.kill() method
The process.kill() method can be used to send a signal to terminate The current Node.js process. This method accepts two parameters: the process ID of the process to be terminated, and the name or number of the signal to be sent. The default signal is SIGTERM, but other signals can be used (such as SIGHUP, SIGINT, SIGQUIT, SIGKILL, etc.).
The following is a sample code:
process.kill(process.pid, 'SIGTERM');
- Using the setTimeout() and clearTimeout() methods
The setTimeout() method can be used to Call functions. If you need to cancel a function call within a specified time, you can use the clearTimeout() method. This method takes a timer identifier as a parameter to cancel the timer that has been created with the setTimeout() method.
The following is a sample code:
const timeoutId = setTimeout(() => { console.log('Function will be executed after 10 seconds'); }, 10000); // 10 秒后执行 clearTimeout(timeoutId); // 取消定时器
The above are several ways to interrupt code execution in Node.js. In actual development, choosing the appropriate method according to the specific situation can terminate code execution more efficiently.
The above is the detailed content of How to interrupt code in nodejs. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


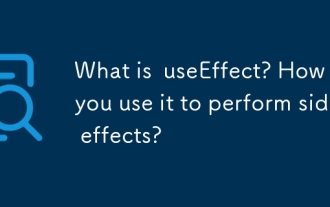
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
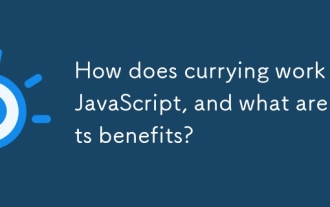
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
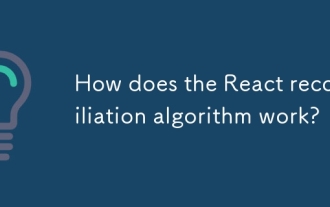
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
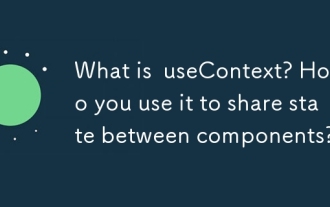
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
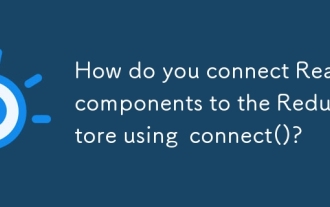
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.
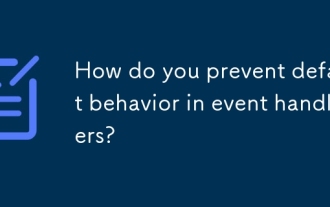
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
