Vue implements local jump
With the widespread application of Vue, we often need to implement local jump functions in single-page applications. This kind of jump mainly refers to the link jump within the page, such as realizing a "return to the top" function, or realizing the function of switching different content in a single-page application.
After some research, I found two more effective implementation methods, namely using anchor points and programming.
Using anchor points
Anchor point is an HTML syntax that can jump within the same page. The implementation principle is to add an id attribute to the specified element in the page, and then jump to the element within the page by adding an anchor point to the link.
It is also very simple to implement anchor point jump in Vue. First we need to add a unique id to the element we want to jump to, for example:
<div id="example">这里是文本内容</div>
Then we can jump by adding the corresponding anchor point to the link on the page:
<a href="#example">跳转到示例</a>
The code to implement anchor point jump in Vue is as follows:
this.$router.push({ path: '/', hash: '#example' })
Among them, $router
is the routing object provided in Vue-Router, path: '/'
means jumping to the current page, and hash: '#example'
means jumping to the id of the specified element.
Achieved programmatically
In addition to using anchor points, we can also implement local jumps programmatically. This implementation method will be more flexible in some special scenarios.
Vue-Router provides many APIs to implement programmatic navigation. Among them, the most commonly used APIs are the $router.push
method and the $router.replace
method. Both methods can be used to implement page jumps, but their implementation methods are slightly different.
$router.push
The method will add the URL of the current page to the browser's routing history and load a new page using the new URL, thereby achieving page jumps. The code is as follows:
this.$router.push('/path/to/destination')
The $router.replace
method does not add a new record to the browser history, but directly replaces the current URL. The code is as follows:
this.$router.replace('/path/to/destination')
If we want to implement the local jump function, we can first obtain the position information of the target element that needs to be jumped, and then use $router.push
or $router.replace
method implements jump.
To give a practical example, in a single-page application, we can implement a "return to top" function. First, we need to get the position information of the target element by writing a function:
function getTargetPosition() { const target = document.querySelector('#target') if (!target) return null const targetRect = target.getBoundingClientRect() return { x: targetRect.left + window.pageXOffset, y: targetRect.top + window.pageYOffset } }
Then, we can add a "return to top" button to the page. When the user clicks the button, a local jump can be achieved. Transfer:
<button @click="backToTop">返回顶部</button>
The corresponding Vue method is as follows:
methods: { backToTop() { const position = getTargetPosition() if (!position) return this.$router.push({ path: '/', query: { position } }) } }
In this method, we first obtain the position information of the target element, and then pass the $router.push
method Implement the jump. Note that this is different from anchor point jump. In the $router.push
method, we use the query
attribute to pass the position information of the target element.
Finally, in the target page, we can obtain the passed location information through $route.query
, so as to scroll the page to the specified position:
mounted() { const { position } = this.$route.query if (position) { window.scrollTo(position.x, position.y) } }
In this way, we can implement the local jump function in single-page applications.
Summary
For local jumps in single-page applications, we can achieve it by using anchor points or programmatically. Among them, the anchor point method is simpler and more intuitive, but may have limitations in some scenarios. The programming method can more flexibly control the behavior of page jumps, but the corresponding implementation code requires more work.
The above is the detailed content of Vue implements local jump. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.
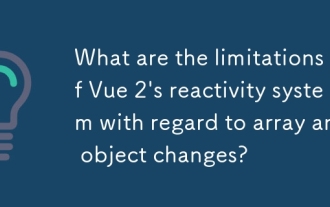
Vue 2's reactivity system struggles with direct array index setting, length modification, and object property addition/deletion. Developers can use Vue's mutation methods and Vue.set() to ensure reactivity.

React components can be defined by functions or classes, encapsulating UI logic and accepting input data through props. 1) Define components: Use functions or classes to return React elements. 2) Rendering component: React calls render method or executes function component. 3) Multiplexing components: pass data through props to build a complex UI. The lifecycle approach of components allows logic to be executed at different stages, improving development efficiency and code maintainability.
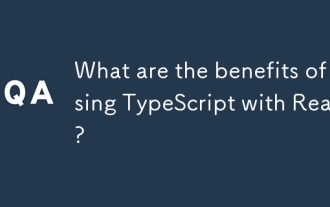
TypeScript enhances React development by providing type safety, improving code quality, and offering better IDE support, thus reducing errors and improving maintainability.

React is the preferred tool for building interactive front-end experiences. 1) React simplifies UI development through componentization and virtual DOM. 2) Components are divided into function components and class components. Function components are simpler and class components provide more life cycle methods. 3) The working principle of React relies on virtual DOM and reconciliation algorithm to improve performance. 4) State management uses useState or this.state, and life cycle methods such as componentDidMount are used for specific logic. 5) Basic usage includes creating components and managing state, and advanced usage involves custom hooks and performance optimization. 6) Common errors include improper status updates and performance issues, debugging skills include using ReactDevTools and Excellent
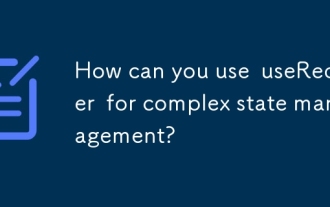
The article explains using useReducer for complex state management in React, detailing its benefits over useState and how to integrate it with useEffect for side effects.
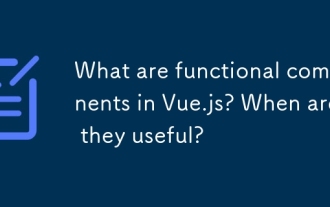
Functional components in Vue.js are stateless, lightweight, and lack lifecycle hooks, ideal for rendering pure data and optimizing performance. They differ from stateful components by not having state or reactivity, using render functions directly, a
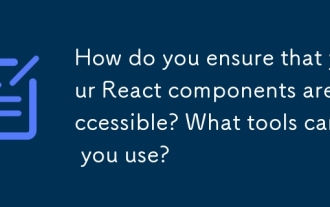
The article discusses strategies and tools for ensuring React components are accessible, focusing on semantic HTML, ARIA attributes, keyboard navigation, and color contrast. It recommends using tools like eslint-plugin-jsx-a11y and axe-core for testi
