Getting Started with PHP: Multi-Process Communication
As a server-side language, PHP often needs to handle a large number of concurrent requests. In order to better cope with high concurrency scenarios, PHP supports multi-process and multi-thread programming. This article will introduce the basic concepts, common methods and implementation techniques of PHP multi-process communication, and help PHP beginners quickly master multi-process programming skills.
1. Basic concepts of multi-process communication
- Process
Process (process) is a basic concept in the operating system and refers to a running program instance. Each process has its own independent memory space, running status and resource usage, without interfering with each other. In PHP, each PHP script can be regarded as a process. - Inter-Process Communication
Inter-Process Communication (IPC, Inter-Process Communication) refers to the way of data exchange and coordination between different processes. In multi-process programming, inter-process communication is the key to achieving process synchronization and data sharing. - Process Synchronization
Process synchronization (process synchronization) refers to the process of coordination and synchronization between multiple processes to complete a certain task. In multi-process programming, process synchronization is an important means to ensure the sequential execution of processes, avoid resource competition, and avoid deadlocks. - Data sharing
Data sharing (data sharing) refers to the way in which multiple processes share the same data. In multi-process programming, data sharing is the core goal to achieve inter-process communication and collaborative task completion.
2. Common methods for PHP to implement multi-process communication
- Named pipe
Named pipe (named pipe) is a special file type used on the same computer Communication between different processes on the machine. Named pipes can safely pass one or more structured sets of data. In PHP, named pipes can be created using the posix_mkfifo() function. - Shared memory
Shared memory (shared memory) refers to the same memory area that multiple processes can read and write together. In PHP, you can use the shmop_* series of functions to achieve operations such as creating, reading, writing, and deleting shared memory. - Semaphore
Semaphore (semaphore) is a mechanism in the operating system for process synchronization and mutual exclusion. In PHP, you can use the sem_* series of functions to implement resource allocation, access, and release operations between processes. - Message queue
Message queue (message queue) is a way of communication between processes, which can asynchronously transmit structured messages between multiple processes. In PHP, you can use the msg_* series of functions to implement operations such as creating, reading, writing, and deleting message queues. - Socket Socket
Socket (socket) is a method of inter-process communication that can communicate between different machines. In PHP, you can use the socket_* series of functions to implement Socket programming and complete TCP/IP network communication and data transmission.
3. PHP multi-process programming implementation skills
1. Use the pcntl_fork() function to create a child process
In PHP, you can use the pcntl_fork() function to create a child process. The parent process will copy its own memory space to the child process, but the memory of the child process is independent of the parent process. You can use the pcntl_wait() function to wait for the child process to complete the task to ensure that the result returned is correct.
2. Use signal processing functions to handle process exceptions
In PHP multi-process programming, child processes may exit abnormally for various reasons. In order to catch these exceptions and handle them in time, you can register a signal handling function. When the child process receives a signal, it will automatically call the corresponding signal processing function for exception handling.
3. Reasonable use of mutex locks
In multi-process programming, in order to avoid resource competition and data inconsistency, mutex locks (mutex) need to be used to achieve synchronous access between processes. When using mutex locks, avoid deadlocks and resource leaks.
4. Avoid a large number of IO operations
In multi-process programming, if a large number of IO operations are processed, it is easy to cause system crashes and performance degradation. Therefore, when writing PHP multi-process programs, you should try to avoid frequent IO operations, such as network communication and disk reading and writing.
4. Summary
This article introduces the basic concepts, common methods and implementation techniques of PHP multi-process communication. When implementing multi-process programming, you need to pay attention to important aspects such as communication methods between processes, process synchronization and data sharing issues, signal processing, and mutex locks. Only by flexibly mastering various communication methods and techniques can we write high-quality, high-performance PHP multi-process programs.
The above is the detailed content of Getting Started with PHP: Multi-Process Communication. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


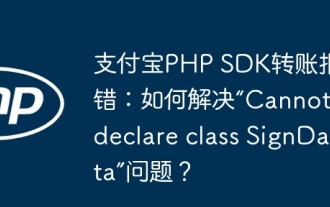
Alipay PHP...
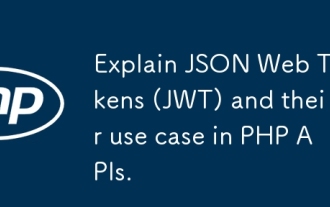
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
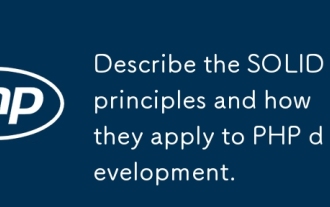
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
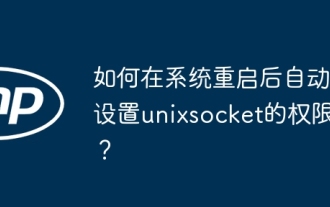
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
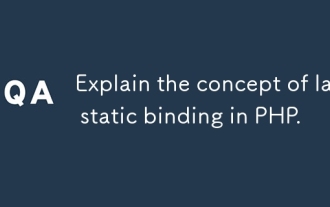
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
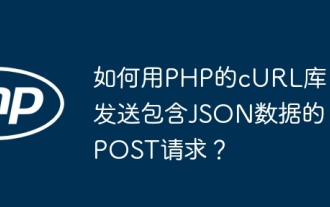
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
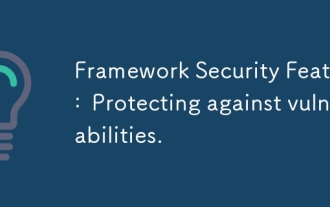
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
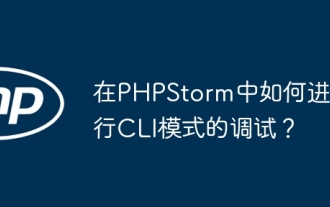
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
