How to write services in nodejs
With the development of Web technology, front-end work content is becoming more and more abundant, especially the development of single-page applications. And this kind of application usually needs to interact with the server side, get data and update the page. Therefore, the server has also become an integral part of front-end development, among which Node.js is a very excellent server-side development language.
In this article, I will introduce how to use Node.js to write services, including the following:
- Install and configure Node.js;
- Create and start Node.js service;
- Process HTTP requests and responses;
- Use the Express framework to manage services;
- Use MongoDB to store and manage data;
- Deploy Node .js served to online server.
- Installing and configuring Node.js
First, we need to install Node.js locally. You can go to the official website (https://nodejs.org/) to download and install the latest version. After the installation is complete, we need to configure the environment variable path to use Node.js.
- Create and start the Node.js service
After installing Node.js, we can create and start the Node.js service. To do this, we need to use the http module that comes with Node.js.
In your project directory, create a file named server.js. Use the following code to create and start a Node.js service.
const http = require('http');
http.createServer(function (req, res) {
res.write('Hello World!'); res.end();
}).listen(8080);
This code creates a local service and listens to the local 8080 port. When you visit http://localhost:8080/ in your browser, you will see "Hello World!". This is a simple Node.js service.
- Handling HTTP requests and responses
On the server side, requests and responses are very important. We can use the req and res objects provided by Node.js to handle requests and responses.
Use the following code to handle requests and responses.
const http = require('http');
http.createServer(function (req, res) {
res.writeHead(200, {'Content-Type': 'text/html'}); res.write('Hello World!'); res.end();
}).listen(8080);
In this code, we use the res.writeHead() method to set the response header and tell the browser that the server returns HTML type text. We then use the res.write() method to output the text content to the page. Finally, we use the res.end() method to tell the server that the response is finished and send the data to the client.
- Use the Express framework to manage services
The http module that comes with Node.js can create simple services, but if we need more complex services and more efficient management , we need to use third-party modules. Among them, Express is a commonly used Node.js development framework, which provides many useful functions and tools to build complex services.
First, we need to install the Express module. Execute the following command in the command line: npm install express --save
When completed, in the server.js file, create an Express application using the following code.
const express = require('express');
const app = express();
app.get('/', function (req, res) {
res.send('Hello World!');
});
app.listen(8080);
In this code, we use the require() method to introduce the Express module. We then create an Express application object using the express() method, and then use the app.get() method to create a routing rule that specifies that when the user accesses the '/' path, the server should return 'Hello World!'. Finally, the service is started using the app.listen() method.
- Use MongoDB to store and manage data
In the process of developing services, data is inevitable. In Node.js, you can use MongoDB to store and manage data.
First, we need to install MongoDB. You can find the relevant download links on the official website https://www.mongodb.com/.
After the installation is complete, we can use the mongoose module to connect and manage the MongoDB database. Use the following code to connect to the MongoDB database in Node.js.
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost/my_database', { useNewUrlParser: true });
In this code , we use the require() method to introduce the mongoose module, and use the mongoose.connect() method to connect to the local MongoDB database. After the connection is successful, we can use mongoose and the corresponding data model to interact with the MongoDB database.
- Deploy the Node.js service to the online server
After you complete the development of your Node.js service, you need to deploy it to the online server. Common cloud service providers include Alibaba Cloud, Tencent Cloud, AWS, etc. Here, we take Alibaba Cloud as an example to introduce how to deploy Node.js services to Alibaba Cloud.
First, you need to purchase an ECS instance on Alibaba Cloud and install Node.js and MongoDB in the instance. Then, you need to use the ssh tool to connect to the Alibaba Cloud ECS instance, upload your Node.js service code and install the corresponding modules and dependencies. Finally, you can use the pm2 module to manage and start your Node.js services.
First, install pm2 using the following command:
npm install pm2 -g
Then, start your Node.js service using the following command:
pm2 start server.js
You can also use pm2 to manage multiple Node.js services, for example:
pm2 start app1.js
pm2 start app2.js
pm2 start app3.js
Summary
This article introduces how to use Node.js to write services. Node.js provides many useful modules and functions to help us create and manage services. We can use the HTTP module to create simple services and handle requests and responses. We can use the Express framework to simplify the creation and management of services. We can also use MongoDB to store and manage data. Finally, we introduced how to deploy Node.js services to online servers.
In the process of learning Node.js development services, you need to master JavaScript, HTTP protocol, database and other technologies. But through continuous practice and learning, you will gradually become an excellent Node.js developer.
The above is the detailed content of How to write services in nodejs. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


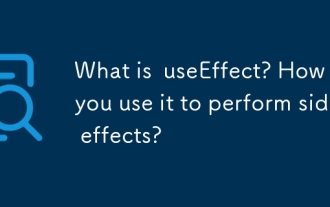
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
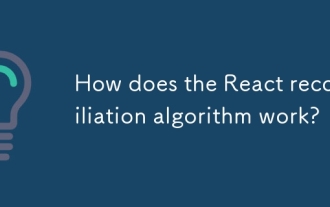
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
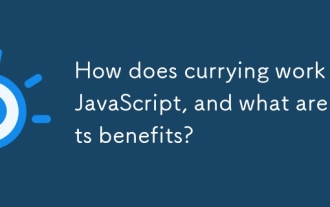
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
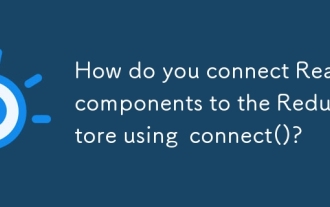
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.
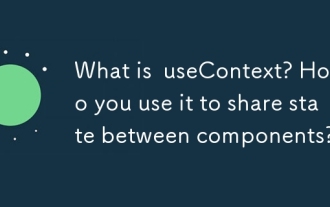
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
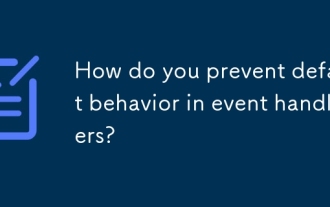
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
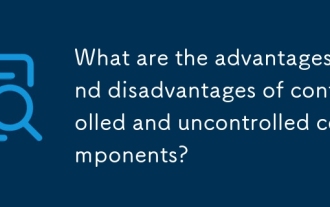
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
