How to traverse the serial numbers of an array in php
In PHP, traversing the serial numbers of an array is a common task. Array is an important data structure. In PHP, you can use different methods to traverse the sequence numbers of the array. The following will explain two common methods of traversing arrays and their advantages and disadvantages.
Method 1: Use a for loop to traverse the array sequence number
The for loop is a commonly used control flow statement, used to execute the same code block multiple times. In PHP, you can use a for loop to traverse the sequence numbers of an array. The for loop requires a start value, an end value, and a stride value. We can use the count() function to get the length of the array and then use a for loop to iterate through the entire array. The following is a sample code:
$arr = array('apple', 'banana', 'orange'); for ($i = 0; $i < count($arr); $i++) { echo $i . ': ' . $arr[$i] . '<br>'; }
The above code uses a for loop to traverse the serial numbers of the array $arr and output the serial numbers and corresponding elements.
Advantages: The for loop is a simple and intuitive way to traverse an array, with a small amount of code and easy to understand.
Disadvantages: Each loop needs to determine the length of the array. If the array is very large, this will cause performance problems.
Method 2: Use foreach loop to traverse array numbers
The foreach loop is one of the most commonly used methods to traverse arrays in PHP. Using a foreach loop, you only need to provide an array variable and a temporary variable to traverse the entire array. The following is a sample code:
$arr = array('apple', 'banana', 'orange'); foreach ($arr as $i => $value) { echo $i . ': ' . $value . '<br>'; }
The above code uses a foreach loop to traverse the serial numbers of the array $arr, and output the serial numbers and corresponding elements.
Advantages: Each loop does not need to determine the length of the array, which will improve the execution efficiency of the code. The foreach loop is also very concise and easy to understand and maintain.
Disadvantages: If you only need to traverse the serial number of the array, you need to use additional temporary variables, which will increase the complexity of the code.
Suggestion: If you need to traverse the sequence numbers and elements of the array, it may be more appropriate to use a foreach loop. If you only need to traverse the serial numbers of the array, it may be more intuitive to use a for loop.
Conclusion
In PHP, traversing the serial numbers of an array is a common task. You can use a for loop or a foreach loop to traverse the sequence numbers of the array. The for loop is simple and intuitive, while the foreach loop is more efficient and easy to understand and maintain. According to actual needs, you can choose an appropriate method to traverse the serial numbers of the array.
The above is the detailed content of How to traverse the serial numbers of an array in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


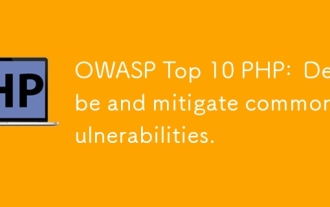
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
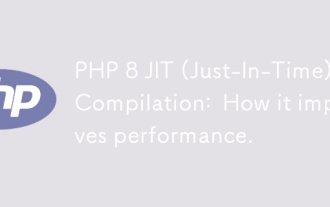
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
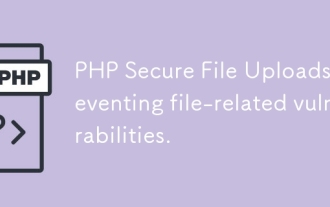
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
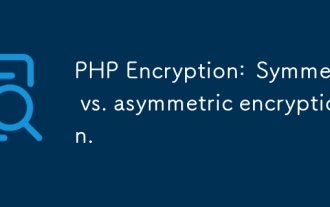
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
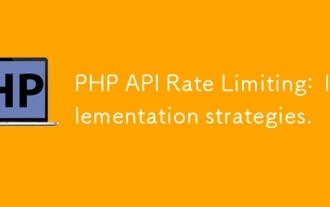
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
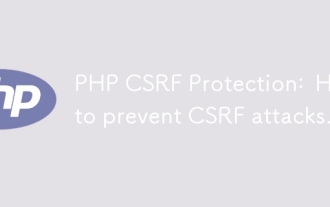
The article discusses strategies to prevent CSRF attacks in PHP, including using CSRF tokens, Same-Site cookies, and proper session management.

Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
