


Detailed explanation of the attributes and related operations of elements in jQuery_jquery
Element attributes
The attributes of theelement can contain a lot of useful information, so how to set or get the value in the attribute is very important.
jQuery’s $.fn.attr method can be used as a setter and getter to set or get the value of an attribute. Similar to $.fn.css usage, $.fn.attr can accept either a single attribute at once or multiple attributes (objects):
$('a').attr('href', 'allMyHrefsAreTheSameNow.html'); $('a').attr({ 'title' : 'all titles are the same too!', 'href' : 'somethingNew.html' });
When writing objects in the above code, it is written in multiple lines, which is more readable.
$('a').attr('href'); // 返回选择其中第一个超链接的链接地址
Once there are elements in the selector's result set, these elements can be used as reference points to traverse other elements. For details on how jQuery traverses elements, see http://api.jquery.com/category/traversing/, such as:
$('h1').next('p'); $('div:visible').parent(); $('input[name=first_name]').closest('form'); $('#myList').children(); $('li.selected').siblings();
You can also use the $.fn.each method to process the elements in the result set one by one:
$('#myList li').each(function(idx, el) { console.log( 'Element ' + idx + 'has the following html: ' + $(el).html() ); });
Move, copy, delete elements
If you want to move the position of an element:
// 把第一个列表移至最后 var $li = $('#myList li:first').appendTo('#myList'); // 另外一种方法,也能达到同样效果 $('#myList').append($('#myList li:first'));
Copy an element
// 把第一个 li 做一份拷贝,然后放置列表的最后 $('#myList li:first').clone().appendTo('#myList');
If you want to copy the element's attributes, events and other information together when copying an element, just give the parameter true when calling $.fn.clone.
Let’s talk about deleting elements. There are two methods in jQuery to delete elements: $.fn.remove and $.fn.detach. Both methods can delete elements from the page, and the return values of these two methods are They are all deleted elements. The difference is that the element returned by $.fn.remove no longer contains some ancillary information of the element, such as id and class information, nor does it include events bound to the element. $.fn.detach is different. The ancillary information and events in the deleted element are also saved. Which one to use depends on the actual needs.
Create new element
jQuery can quickly replace new elements:
$('<p>这是一个新段落</p>'); $('<li class="new">新列表元素</li>'); $('<a/>', { html : '这是一个 <strong>新</strong> 超链接', 'class' : 'new', href : 'foo.html' });
Note that in the JavaScript object passed above, the second attribute class inside is quoted. Because class is a reserved word of JavaScript, html and href are not, so there is no need to add quotes.
After creating a new element, the new element will not be automatically added to the page. To add it to the page, you can use the following method:
var $myNewElement = $('<p>New element</p>'); $myNewElement.appendTo('#content'); $myNewElement.insertAfter('ul:last'); // 此操作会把 p 元素从 #content 中移除 $('ul').last().after($myNewElement.clone()); // 当然也可以克隆一个出来,现在 #content 中有两个 p 了哦
Strictly speaking, it is not necessary to save the newly created element in a variable. It can be added directly to the page after creation. But many times the newly created element is used multiple times, so it needs to be cached in a variable so that it does not have to be created repeatedly.
You can even create it when adding an element to the page, but there is no way to get a reference to the newly created element in this case:
$('ul').append('<li>list item</li>');
Adding new elements to the page is very simple, but if you need to add many, many new elements to the page, there may be performance issues. Because every time an element is added to the page, the HTML of the entire page must be concatenated as a string, which is very performance-consuming. In this case, there are usually the following solutions:
var myItems = [], $myList = $('#myList'); for (var i=0; i<100; i++) { myItems.push('<li>item ' + i + '</li>'); } $myList.append(myItems.join(''));

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


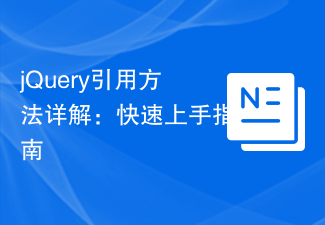
Detailed explanation of jQuery reference method: Quick start guide jQuery is a popular JavaScript library that is widely used in website development. It simplifies JavaScript programming and provides developers with rich functions and features. This article will introduce jQuery's reference method in detail and provide specific code examples to help readers get started quickly. Introducing jQuery First, we need to introduce the jQuery library into the HTML file. It can be introduced through a CDN link or downloaded
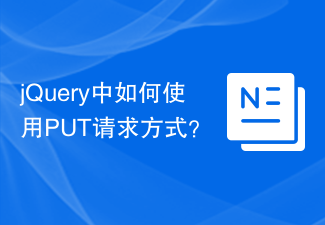
How to use PUT request method in jQuery? In jQuery, the method of sending a PUT request is similar to sending other types of requests, but you need to pay attention to some details and parameter settings. PUT requests are typically used to update resources, such as updating data in a database or updating files on the server. The following is a specific code example using the PUT request method in jQuery. First, make sure you include the jQuery library file, then you can send a PUT request via: $.ajax({u
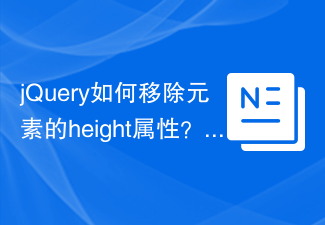
How to remove the height attribute of an element with jQuery? In front-end development, we often encounter the need to manipulate the height attributes of elements. Sometimes, we may need to dynamically change the height of an element, and sometimes we need to remove the height attribute of an element. This article will introduce how to use jQuery to remove the height attribute of an element and provide specific code examples. Before using jQuery to operate the height attribute, we first need to understand the height attribute in CSS. The height attribute is used to set the height of an element
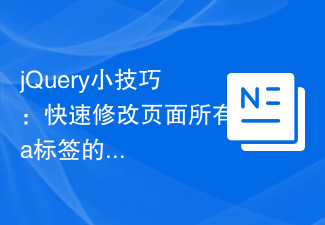
Title: jQuery Tips: Quickly modify the text of all a tags on the page In web development, we often need to modify and operate elements on the page. When using jQuery, sometimes you need to modify the text content of all a tags in the page at once, which can save time and energy. The following will introduce how to use jQuery to quickly modify the text of all a tags on the page, and give specific code examples. First, we need to introduce the jQuery library file and ensure that the following code is introduced into the page: <
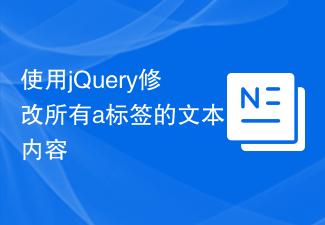
Title: Use jQuery to modify the text content of all a tags. jQuery is a popular JavaScript library that is widely used to handle DOM operations. In web development, we often encounter the need to modify the text content of the link tag (a tag) on the page. This article will explain how to use jQuery to achieve this goal, and provide specific code examples. First, we need to introduce the jQuery library into the page. Add the following code in the HTML file:
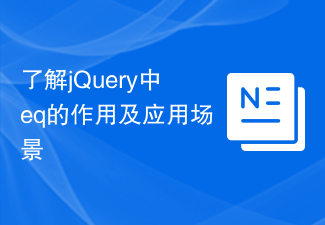
jQuery is a popular JavaScript library that is widely used to handle DOM manipulation and event handling in web pages. In jQuery, the eq() method is used to select elements at a specified index position. The specific usage and application scenarios are as follows. In jQuery, the eq() method selects the element at a specified index position. Index positions start counting from 0, i.e. the index of the first element is 0, the index of the second element is 1, and so on. The syntax of the eq() method is as follows: $("s
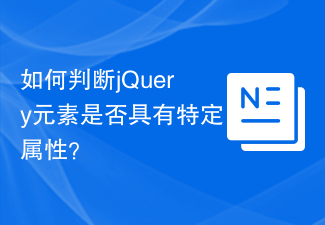
How to tell if a jQuery element has a specific attribute? When using jQuery to operate DOM elements, you often encounter situations where you need to determine whether an element has a specific attribute. In this case, we can easily implement this function with the help of the methods provided by jQuery. The following will introduce two commonly used methods to determine whether a jQuery element has specific attributes, and attach specific code examples. Method 1: Use the attr() method and typeof operator // to determine whether the element has a specific attribute
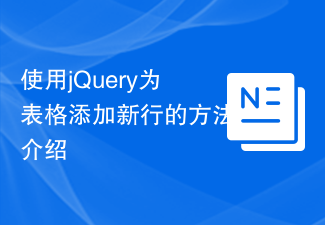
jQuery is a popular JavaScript library widely used in web development. During web development, it is often necessary to dynamically add new rows to tables through JavaScript. This article will introduce how to use jQuery to add new rows to a table, and provide specific code examples. First, we need to introduce the jQuery library into the HTML page. The jQuery library can be introduced in the tag through the following code:
