nodejs build grpc
Preface
gRPC is Google’s open source, high-performance cross-language RPC framework. Its goal is to allow client applications to call server-side methods just like calling local functions. It supports multiple programming languages, including: C, Languages such as Python, Java, Go, Node.js and more.
Using gRPC in Node.js can greatly facilitate our interaction between the server and the client, and while ensuring high performance, it can also provide data security and confidentiality. This article will introduce how to use Node. js to build gRPC server and client.
Step One: Install Node.js and gRPC
First, you need to ensure that Node.js and npm have been installed locally. Then, enter the following command in the terminal to install gRPC:
npm install -g grpc
After the installation is complete, you can see the version information of gRPC in the package.json file.
Step 2: Define the .proto file
gRPC defines the service through proto-file, which is used to define the service interface, message format, etc. . Next, we first define a simple service that allows the client to send a message to the server and return a modified message after receiving the message. Create a file named example.proto and define the following content:
syntax = "proto3"; package example; service Example { rpc ModifyMessage (Message) returns (Message){} } message Message { string content = 1; }
Step 3: Generate code
Run the following command in the directory where the example.proto file is located to generate the corresponding code :
grpc_tools_node_protoc --js_out=import_style=commonjs,binary:./ --grpc_out=./ --plugin=protoc-gen-grpc=`which grpc_tools_node_protoc_plugin` example.proto
grpc_tools_node_protoc is used here to generate the code required for Node.js, and the output directory is specified.
The generated code includes: example_pb.js and example_grpc_pb.js.
Step 4: Implement the server
The server-side code is as follows:
const grpc = require("grpc"); const example = require("./example_pb"); const exampleService = require("./example_grpc_pb"); const server = new grpc.Server(); function modifyMessage(call, callback) { const response = new example.Message(); response.setContent(call.request.getContent().toUpperCase()); callback(null, response); } server.addService(exampleService.ExampleService, { modifyMessage: modifyMessage, }); server.bind("localhost:50051", grpc.ServerCredentials.createInsecure()); console.log("Server running at http://localhost:50051"); server.start();
In this example, the server creates a new grpc server and adds A method named modifyMessage. This method receives a Message object as a parameter, converts the content field in the Message object to uppercase and returns it.
Finally, we use the bind() method to bind the service to localhost:50051 and start the server.
Step 5: Implement the client
The client code is as follows:
const grpc = require("grpc"); const example = require("./example_pb"); const exampleService = require("./example_grpc_pb"); const client = new exampleService.ExampleClient( "localhost:50051", grpc.credentials.createInsecure() ); const request = new example.Message(); request.setContent("Hello World!"); client.modifyMessage(request, function (err, response) { console.log("Modified message: ", response.getContent()); });
In this example, we create an ExampleClient object and use its modifyMessage() Method sends a Message object to the server. Finally, we output the response from the server, which has converted the letters in the string to uppercase letters.
Step 6: Run the service
Now, we only need to use the following command to start the service in the directory where the server code is located:
node server.js
Then, in the directory where the client code is located Run the following command in the directory:
node client.js
You should see the following output:
Modified message: HELLO WORLD!
At this point, we have successfully implemented a basic gRPC server and client interaction process.
Summary
This article introduces how to use Node.js to build gRPC server and client, and uses protobuf to define the data structure and message format between creating services and establishing connections. gRPC is a powerful cross-language RPC framework that is useful for applications that need to quickly transfer data between clients and servers.
The above is the detailed content of nodejs build grpc. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
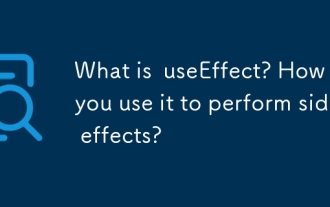
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
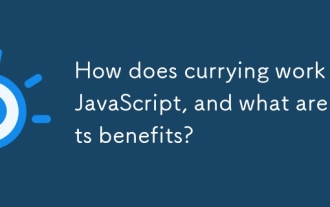
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
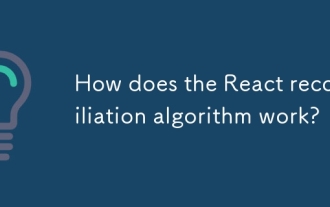
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
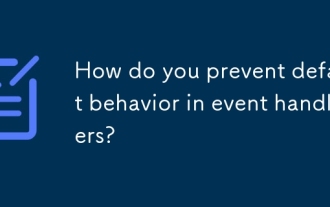
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
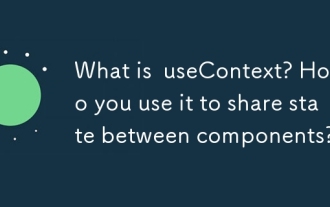
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
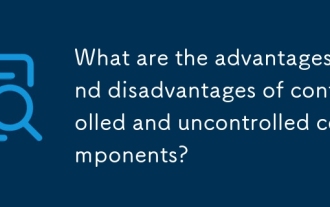
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
