nodejs receives post request parameters
Node.js, as a server-side JavaScript running environment, is very common when processing HTTP requests. Among them, receiving the parameters of the post request is a very basic thing. Next, we will learn how to use Node.js to receive and parse post request parameters.
1. HTTP request in Node.js
In Node.js, we can create an HTTP server through the built-in http module. Here is a simple example of how to create a simple HTTP server:
const http = require('http'); const server = http.createServer((req, res) => { res.end('Hello World!'); }); server.listen(3000, () => { console.log('Server running at http://localhost:3000/'); });
This server simply responds with a Hello World!
string. We can use the curl
command to test:
curl http://localhost:3000/
We can see that after running the curl command, the server will reply with a Hello World!
string.
2. Post request in HTTP request
In HTTP requests, GET request and POST request are the two most common and basic request methods. For HTTP GET requests, its parameters will be passed in the URL, while POST requests will send key-value pair data in the body of the request.
Of course, although the GET request can also carry parameters in the body, this method is not safe. POST requests can avoid this situation.
3. Node.js handles POST requests
When the server receives the POST request, we need to get the parameters from the request body. Here is the most common way to handle POST requests using Node.js:
const http = require('http'); const server = http.createServer((req, res) => { if(req.method === 'POST') { let postData = ''; req.on('data', chunk => { postData += chunk.toString(); }); req.on('end', () => { console.log('postData:', postData); res.end('Hello World!'); }) } else { res.end('Hello World!'); } }); server.listen(3000, () => { console.log('Server running at http://localhost:3000/'); });
The main idea here is that when the client sends a POST request, we listen for on
req data
event and end
event. In the data
event, we continuously read the body of the request and store the data in a variable in the form of a string. After the end
event is triggered, we can process the received parameters.
4. Parse the request parameters
After obtaining the parameters in the POST request, we need to parse the parameters. Generally speaking, parameters in POST requests are sent in key-value form, which is the style used by our common form data.
In Node.js, we can parse these parameters by using the querystring
module. Here is an example:
const http = require('http'); const querystring = require('querystring'); const server = http.createServer((req, res) => { if(req.method === 'POST') { let postData = ''; req.on('data', chunk => { postData += chunk.toString(); }); req.on('end', () => { console.log('postData:', postData); const body = querystring.parse(postData); console.log('body:', body); res.end('Hello World!'); }) } else { res.end('Hello World!'); } }); server.listen(3000, () => { console.log('Server running at http://localhost:3000/'); });
In the above example, we first used the built-in querystring
module of Node.js. In the end
event, we parse the received POST parameters using the querystring.parse()
method, and then output it to the console for viewing.
5. Use Express to process POST requests
In addition to using Node.js’s built-in http module to process POST requests, we can also use the popular server-side framework Express. In Express, we can use the body-parser
middleware to process parameters in POST requests. Here is an example using Express and body-parser
:
const express = require('express'); const bodyParser = require('body-parser'); const app = express(); // 将JSON请求体解析中间件,放在路由之前 app.use(bodyParser.json()); // 处理URL编码请求体的中间件 app.use(bodyParser.urlencoded({extended: false})); app.post('/', (req, res) => { console.log('body:', req.body); res.send('Hello World!'); }); app.listen(3000, () => { console.log('Server running at http://localhost:3000/'); });
In this example, we first use the Express framework and use body-parser
in the middle software to handle parameters in POST requests. In the post
route, we can directly obtain the parameters in the POST request through req.body
and output them to the console and response.
Summary
To process POST requests in Node.js, we need to use the built-in http module of Node.js or the popular framework Express, and then implement parameter parsing and processing. For beginners, it is best to first understand how to use the http module in Node.js before considering using popular frameworks. At the same time, when processing POST requests, we also need to consider security issues to ensure that the transmitted parameters will not be obtained by third parties.
The above is the detailed content of nodejs receives post request parameters. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
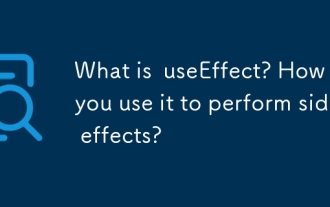
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
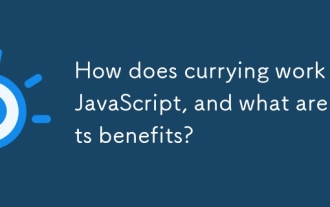
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
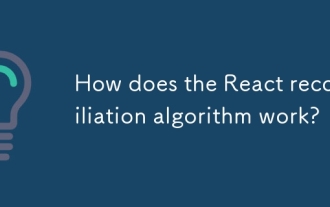
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
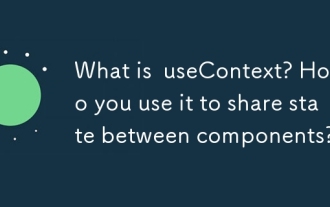
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
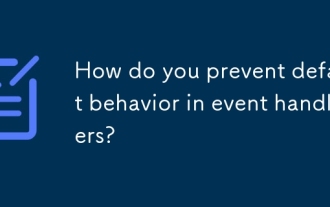
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
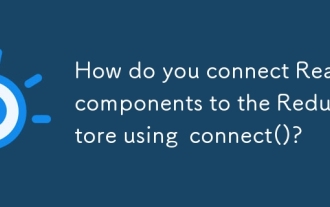
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.
