thinkphp overrides base class methods
In the ThinkPHP5 framework, the base class (Base) is a very important class. It is the parent class of all controllers and contains many important methods, such as: controller initialization, template rendering, template output, etc. In actual development, we sometimes need to rewrite some methods in the base class to meet our specific business needs. So, how to override base class methods in the ThinkPHP5 framework? I'll go into detail below.
The first step is to find the base class file
In the ThinkPHP5 framework, the base class (Base) file is in "thinkController.php". The default directory of this file is "/thinkphp/library/think/", and the file can also be found through "topthink/framework" installed by composer. Therefore, we need to find the base class file of the controller before we can modify it.
The second step is to write a custom method
Before overriding the base class method, we need to write a custom method. The code logic of this method needs to be written according to actual business needs, and it can be called anywhere needed in the controller. For example, we need to determine whether the current user is logged in before the controller outputs. If the current user is not logged in, jump to the login page.
The specific operations are as follows:
<?php namespace appindexcontroller; use thinkController; class Base extends Controller { public function _initialize() { parent::_initialize(); // ... } public function index() { // ... } public function checkLogin() { // 判断用户是否登录 if(!session('?user_id')) { $this->redirect('user/login'); } } }
In the above sample code, we wrote a custom method named "checkLogin" to determine whether the user is logged in. When the user is not logged in, this method will jump to the specified login page through the redirect method.
The third step is to rewrite the base class method
After writing our own custom method, we can rewrite the base class method. The base class methods in the ThinkPHP5 framework are all protected or public type methods. You can usually achieve your own business needs by inheriting the base class and overriding the base class methods. For example, we need to determine whether the current user is logged in before the controller outputs. If already logged in, there is no need to jump.
The specific operations are as follows:
<?php namespace appindexcontroller; use thinkController; class Base extends Controller { public function _initialize() { parent::_initialize(); $this->checkLogin(); // 调用自定义方法检测用户是否登录 } public function index() { // ... } // 重写基类的redirect方法 protected function redirect($url, $params = array(), $code = 302, $with_prefix = false) { // 当前用户已登录,则直接输出模板 if(session('?user_id')) { parent::redirect($url, $params, $code, $with_prefix); } // 当前用户未登录,则跳转至登录页面 else { parent::redirect('user/login'); } } }
In the above example code, we override the redirect method of the base class to determine whether the current user is logged in. When the user is logged in, the template will be output directly; when the user is not logged in, it will automatically jump to the login page.
Summary
In the ThinkPHP5 framework, rewriting base class methods can help us realize our own business needs. Overriding base class methods needs to follow the signature and semantics of the base class method, and the implementation of the base class method needs to be carefully analyzed before modification. Rewriting the base class method needs to be done in the controller. The specific steps include: first writing your own custom method; then rewriting the base class method; and finally calling the custom method where needed.
The above is the detailed content of thinkphp overrides base class methods. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


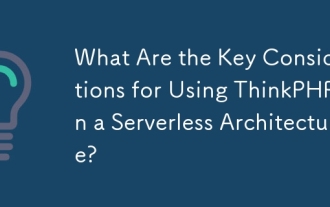
The article discusses key considerations for using ThinkPHP in serverless architectures, focusing on performance optimization, stateless design, and security. It highlights benefits like cost efficiency and scalability, but also addresses challenges
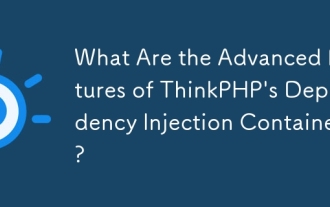
ThinkPHP's IoC container offers advanced features like lazy loading, contextual binding, and method injection for efficient dependency management in PHP apps.Character count: 159
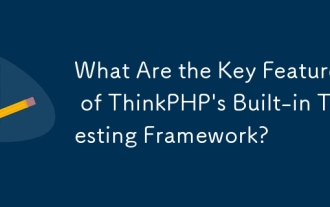
The article discusses ThinkPHP's built-in testing framework, highlighting its key features like unit and integration testing, and how it enhances application reliability through early bug detection and improved code quality.
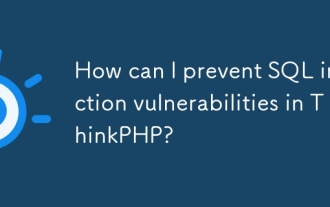
The article discusses preventing SQL injection vulnerabilities in ThinkPHP through parameterized queries, avoiding raw SQL, using ORM, regular updates, and proper error handling. It also covers best practices for securing database queries and validat
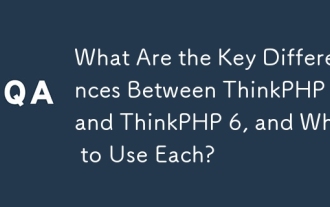
The article discusses key differences between ThinkPHP 5 and 6, focusing on architecture, features, performance, and suitability for legacy upgrades. ThinkPHP 5 is recommended for traditional projects and legacy systems, while ThinkPHP 6 suits new pr
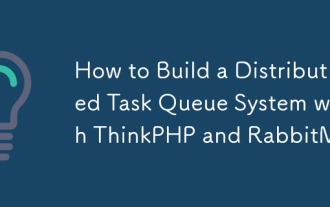
The article outlines building a distributed task queue system using ThinkPHP and RabbitMQ, focusing on installation, configuration, task management, and scalability. Key issues include ensuring high availability, avoiding common pitfalls like imprope
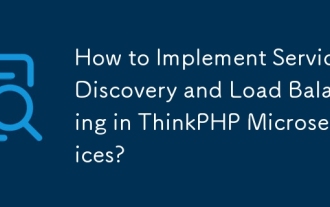
The article discusses implementing service discovery and load balancing in ThinkPHP microservices, focusing on setup, best practices, integration methods, and recommended tools.[159 characters]
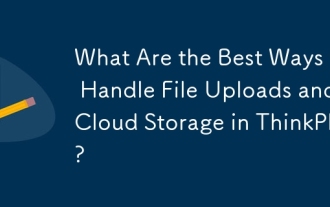
The article discusses best practices for handling file uploads and integrating cloud storage in ThinkPHP, focusing on security, efficiency, and scalability.
