How to use MySQL window functions to achieve list ranking
First, build a test table
create table praise_record( id bigint primary key auto_increment, name varchar(10), praise_num int ) ENGINE=InnoDB;
Then let chatGpt generate several pieces of test data for us
INSERT INTO praise_record (name, praise_num) VALUES ('John', 5); INSERT INTO praise_record (name, praise_num) VALUES ('Jane', 3); INSERT INTO praise_record (name, praise_num) VALUES ('Bob', 10); INSERT INTO praise_record (name, praise_num) VALUES ('Alice', 3); INSERT INTO praise_record (name, praise_num) VALUES ('David', 7); INSERT INTO praise_record (name, praise_num) VALUES ('oct', 7);
Then we can start to realize our needs: return the list of likes, And return the ranking
rank()
Use the rank() function to return the list of likes, rank() over()
## 注意这里返回的rank字段要用反引号包起来 select name, praise_num, rank() over (order by praise_num desc) as `rank` from praise_record; +-------+------------+------+ | name | praise_num | rank | +-------+------------+------+ | Bob | 10 | 1 | | David | 7 | 2 | | oct | 7 | 2 | | John | 5 | 4 | | Jane | 3 | 5 | | Alice | 3 | 5 | +-------+------------+------+
When When using the rank() function, the same number of likes will get the same ranking, and the ranking may jump, so the final ranking will not be continuous
dense_rank()
Use the dense_rank() function to return the list of likes, dense_rank() over()
select name, praise_num, dense_rank() over (order by praise_num desc) as `rank` from praise_record; +-------+------------+------+ | name | praise_num | rank | +-------+------------+------+ | Bob | 10 | 1 | | David | 7 | 2 | | oct | 7 | 2 | | John | 5 | 3 | | Jane | 3 | 4 | | Alice | 3 | 4 | +-------+------------+------+
The same as the rank() function, the same number of likes will return the same ranking. But the final ranking returned by dense_rank() is a continuous ranking
row_number()
row_number() function returns the list of likes, row_number() over()
select name, praise_num, row_number() over (order by praise_num desc) as `rank` from praise_record; +-------+------------+------+ | name | praise_num | rank | +-------+------------+------+ | Bob | 10 | 1 | | David | 7 | 2 | | oct | 7 | 3 | | John | 5 | 4 | | Jane | 3 | 5 | | Alice | 3 | 6 | +-------+------------+------+
The row_number() function is suitable for use when the returned list only requires serial numbers
The above three functions are all newly added to MySQL8.0, so in older versions such as MySQL5.7 we You can simulate it and learn the implementation principles of these three window functions
Simulation implementation of rank() function
select p1.name, p1.praise_num, count(p2.praise_num) + 1 as `rank` from praise_record p1 left join praise_record p2 on p1.praise_num < p2.praise_num group by p1.name, p1.praise_num order by `rank`; +-------+------------+------+ | name | praise_num | rank | +-------+------------+------+ | Bob | 10 | 1 | | David | 7 | 2 | | oct | 7 | 2 | | John | 5 | 4 | | Jane | 3 | 5 | | Alice | 3 | 5 | +-------+------------+------+
We can use self-joining method to make each score lower than the current Record count of row scores, and finally add 1 to the count value as the ranking of the current row to simulate the simulation implementation of rank()
dense_rank()
select p1.name, p1.praise_num, count(distinct p2.praise_num) + 1 as `dense_rank` from praise_record p1 left join praise_record p2 on p1.praise_num < p2.praise_num group by p1.name, p1.praise_num order by `dense_rank`; +-------+------------+------------+ | name | praise_num | dense_rank | +-------+------------+------------+ | Bob | 10 | 1 | | oct | 7 | 2 | | David | 7 | 2 | | John | 5 | 3 | | Jane | 3 | 4 | | Alice | 3 | 4 | +-------+------------+------------+
The implementation of dense_rank is similar to rank. The only difference is that distinct is added to deduplicate the number of likes, so that the rankings returned for different numbers of likes are continuous
Simulation implementation of row_number
##使用自定义变量得先初始化 set @rowNum = 0; select name, praise_num, @rowNum := @rowNum +1 as `row_number` from praise_record order by praise_num desc ; +-------+------------+------------+ | name | praise_num | row_number | +-------+------------+------------+ | Bob | 10 | 1 | | David | 7 | 2 | | oct | 7 | 3 | | John | 5 | 4 | | Jane | 3 | 5 | | Alice | 3 | 6 | +-------+------------+------------+
We can use A rowNum variable is used to record the row number. The data rowNUm of each row is 1, so that we can get the sequence number we want
The above is the detailed content of How to use MySQL window functions to achieve list ranking. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
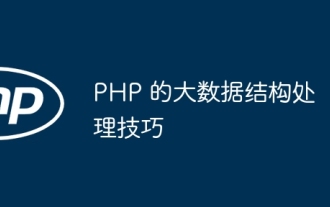
Big data structure processing skills: Chunking: Break down the data set and process it in chunks to reduce memory consumption. Generator: Generate data items one by one without loading the entire data set, suitable for unlimited data sets. Streaming: Read files or query results line by line, suitable for large files or remote data. External storage: For very large data sets, store the data in a database or NoSQL.
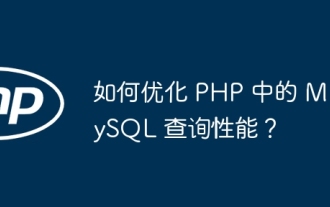
MySQL query performance can be optimized by building indexes that reduce lookup time from linear complexity to logarithmic complexity. Use PreparedStatements to prevent SQL injection and improve query performance. Limit query results and reduce the amount of data processed by the server. Optimize join queries, including using appropriate join types, creating indexes, and considering using subqueries. Analyze queries to identify bottlenecks; use caching to reduce database load; optimize PHP code to minimize overhead.
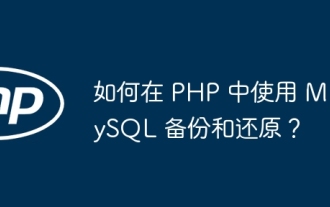
Backing up and restoring a MySQL database in PHP can be achieved by following these steps: Back up the database: Use the mysqldump command to dump the database into a SQL file. Restore database: Use the mysql command to restore the database from SQL files.
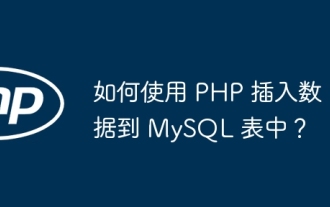
How to insert data into MySQL table? Connect to the database: Use mysqli to establish a connection to the database. Prepare the SQL query: Write an INSERT statement to specify the columns and values to be inserted. Execute query: Use the query() method to execute the insertion query. If successful, a confirmation message will be output.

One of the major changes introduced in MySQL 8.4 (the latest LTS release as of 2024) is that the "MySQL Native Password" plugin is no longer enabled by default. Further, MySQL 9.0 removes this plugin completely. This change affects PHP and other app
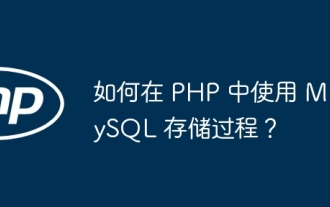
To use MySQL stored procedures in PHP: Use PDO or the MySQLi extension to connect to a MySQL database. Prepare the statement to call the stored procedure. Execute the stored procedure. Process the result set (if the stored procedure returns results). Close the database connection.

Creating a MySQL table using PHP requires the following steps: Connect to the database. Create the database if it does not exist. Select a database. Create table. Execute the query. Close the connection.
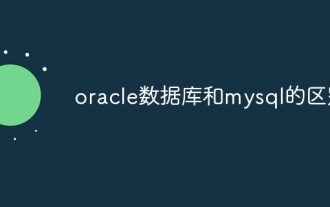
Oracle database and MySQL are both databases based on the relational model, but Oracle is superior in terms of compatibility, scalability, data types and security; while MySQL focuses on speed and flexibility and is more suitable for small to medium-sized data sets. . ① Oracle provides a wide range of data types, ② provides advanced security features, ③ is suitable for enterprise-level applications; ① MySQL supports NoSQL data types, ② has fewer security measures, and ③ is suitable for small to medium-sized applications.
