


Covariance, contravariance, extends and super selection methods of Java generics
To understand covariance and contravariance, we must first introduce:
According to the Liskov substitution principle, if C is a subclass of P, then P can replace C, that is P p = new C();
C inherits from P, denoted as C < P
What is invariant
If F is invariant , when C
Except for example, Integer is a subclass of Number, according to the Liskov substitution principle
Number number = new Integer(1); //correct
But if you write like this, an error will be reported
List<Number> list = new ArrayList<Integer>(1); //error
Although Number and Integer have an inheritance relationship: Integer < Number, in Java, generics are unchanged by default, so they can also be regarded as List<Number>
and List<Integer>
There is no inheritance relationship
What is covariance
If F is covariant, when When C <= P, then F(C) <= F(P)
Java provides an extends to convert invariance into covariance, for example:
List<? extends Number> list = new ArrayList<Integer>(1); //corrent
At this time, List<? extends Number>
can be regarded as the parent class of ArrayList<Integer>
? extend Number
Can be seen as a type range, representing a certain subclass of Number
Arrays are covariant by default
Number[] numbers = new Integer[3];
What is contravariance
If F is contravariant. When C <= P, then F(C) >= F(P)
Java provides a super to convert the invariant into a covariant Change, for example:
List<? super Number> list = new ArrayList<Object>(1); //corrent
At this time, List<? super Number>
can be regarded as the parent class of ArrayList<Object>
extends and super
First, let’s look at the implementation of Collection.add:
public interface List<E> extends Collection<E> { boolean add(E e); }
Will the following code report an error? ? extends Number
does not match the Integer
type
List<? extends Number> list = new ArrayList<Integer>(); // correct list.add(Integer.valueOf(1)); //error
First of all, when the add method is called, the generic E
automatically becomes <? extends Number>
The second line reports an error, which means ? extends Number
is not the parent class of Integer
. Here we need to distinguish List<? extends Number>
is the parent class of ArrayList<Integer>
.
? extends Number
can be regarded as a certain type in a type range, representing a certain subclass of Number, but it is not clear which subclass it is. It may be Float or Short. , or it may be a subclass of Integer (Integer is modified by final and cannot have subclasses. This is just a hypothetical situation). It only determines its upper bound as Number and does not determine the lower bound (there may be ? extends Number
< Integer
), therefore ? extends Number
is not the parent class of Integer
change the above code A slight modification will make it correct:
List<? super Number> list = new ArrayList<Object>(); // correct list.add(Integer.valueOf(1)); //correct
First of all, because of the inversion, List<? super Number>
is the parent class of ArrayList<Object>
. One line is correct.
The second line: ? super Number
is the parent class of Integer
, the reason is: ? super Number
represents a certain parent class of Number , it may be Serializable
or it may be Object
But no matter which one it is, the parent class of Number must be the parent class of Integer, so the second line is also correct
Use extends Or is it super?
The copy method of java.util.Collections (JDK1.7) gives us the answer:
public static <T> void copy(List<? super T> dest, List<? extends T> src) { int srcSize = src.size(); if (srcSize > dest.size()) throw new IndexOutOfBoundsException("Source does not fit in dest"); if (srcSize < COPY_THRESHOLD || (src instanceof RandomAccess && dest instanceof RandomAccess)) { for (int i=0; i<srcSize; i++) dest.set(i, src.get(i)); } else { ListIterator<? super T> di=dest.listIterator(); ListIterator<? extends T> si=src.listIterator(); for (int i=0; i<srcSize; i++) { di.next(); di.set(si.next()); } } }
To convert from generics When a class gets data, use extends;
When you want to write data to a generic class, use super;
You need to both fetch and write , no wildcards are needed (that is, neither extends nor super are used)
private static <E> E getFirst(List<? extends E> list){ return list.get(0); } private static <E> void setFirst(List<? super E> list, E firstElement){ list.add(firstElement); } public static void main(String[] args) { List<Integer> list = new ArrayList<Integer>(); setFirst(list, 1); Number number = getFirst(list); }
The above is the detailed content of Covariance, contravariance, extends and super selection methods of Java generics. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


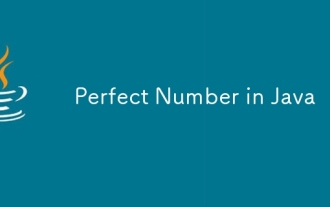
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
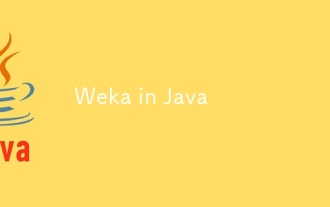
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
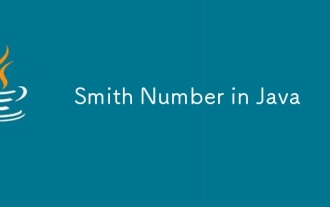
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
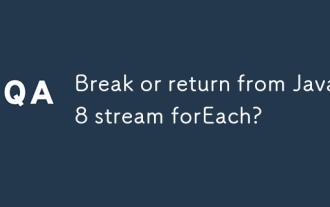
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
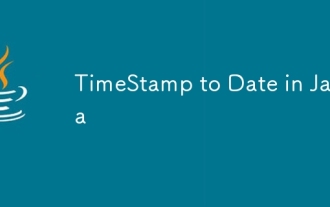
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
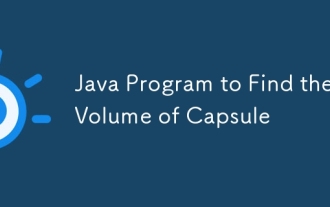
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
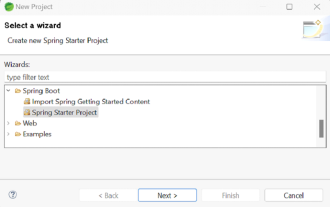
Spring Boot simplifies the creation of robust, scalable, and production-ready Java applications, revolutionizing Java development. Its "convention over configuration" approach, inherent to the Spring ecosystem, minimizes manual setup, allo
