laravel query scope
Query scope in Laravel is a very important feature that allows you to define some commonly used query methods in the model for reuse throughout the entire application. These query scopes can be global (apply to the entire model) or local (apply only to a method of the model).
In this article, we will introduce the definition, use and some things that should be paid attention to the query scope in Laravel.
- Definition of query scope
Query scope is an anonymous function that receives a $query parameter, which is an Eloquent query builder instance. In this anonymous function, you can perform some query operations on the instance, such as adding where clauses, order by clauses, etc.
The following is a basic query scope definition example:
public function scopePublished($query) { return $query->where('status', '=', 'published'); }
In the above example, we define a query scope named published, which will automatically add a query scope when querying where clause to filter published models.
- Using query scope
Using query scope is very simple, just call the corresponding method of the model. For example, if we use the published query scope in the above example, we only need to call the following code:
$posts = Post::published()->get();
The above code will return a collection of all published Post instances.
If you need to pass parameters into the query scope, just add parameters in this method. For example:
public function scopeCategory($query, $categoryId) { return $query->where('category_id', '=', $categoryId); }
This query scope can be used to filter blog posts in a specific category.
$posts = Post::category(1)->get();
The above code will return all blog posts with category ID 1.
Note: Query scopes can be chained, so you can use multiple query scopes at the same time to obtain more precise results.
- Global definition of query scope
If you want to use the same query scope throughout the model, you can define a global query scope. To do this, simply call the boot method in your model and define a global query scope using the static::addGlobalScope method.
The following is a basic global query scope example:
protected static function boot() { parent::boot(); static::addGlobalScope('active', function (Builder $builder) { $builder->where('active', '=', 1); }); }
In the above example, we define a global query scope named active and limit it to the active field equal to 1 record.
Now, when you query the model in any method, the query scope will automatically take effect.
- Remove query scope
Sometimes we may need to remove the query scope of a specific model. Laravel provides the removeGlobalScope method to help us achieve this.
For example, assuming we have a global query scope named active, we can use the following method to remove it:
$users = User::withoutGlobalScope('active')->get();
The above code will return all queries that are not restricted by the active scope user.
Note: If you want to remove all global query scopes, you can use the withoutGlobalScopes method.
$users = User::withoutGlobalScopes()->get();
The above code will return all users not restricted by any global query scope.
Summary
Query scope is an extremely powerful feature in Laravel. By using query scopes, you can easily reuse common query operations throughout your application and organize your code more clearly. Learning the correct use of query scopes can not only improve your productivity, but also help you build more robust applications.
The above is the detailed content of laravel query scope. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

Both Django and Laravel are full-stack frameworks. Django is suitable for Python developers and complex business logic, while Laravel is suitable for PHP developers and elegant syntax. 1.Django is based on Python and follows the "battery-complete" philosophy, suitable for rapid development and high concurrency. 2.Laravel is based on PHP, emphasizing the developer experience, and is suitable for small to medium-sized projects.

How does Laravel play a role in backend logic? It simplifies and enhances backend development through routing systems, EloquentORM, authentication and authorization, event and listeners, and performance optimization. 1. The routing system allows the definition of URL structure and request processing logic. 2.EloquentORM simplifies database interaction. 3. The authentication and authorization system is convenient for user management. 4. The event and listener implement loosely coupled code structure. 5. Performance optimization improves application efficiency through caching and queueing.

PHP and Laravel are not directly comparable, because Laravel is a PHP-based framework. 1.PHP is suitable for small projects or rapid prototyping because it is simple and direct. 2. Laravel is suitable for large projects or efficient development because it provides rich functions and tools, but has a steep learning curve and may not be as good as pure PHP.

LaravelisabackendframeworkbuiltonPHP,designedforwebapplicationdevelopment.Itfocusesonserver-sidelogic,databasemanagement,andapplicationstructure,andcanbeintegratedwithfrontendtechnologieslikeVue.jsorReactforfull-stackdevelopment.
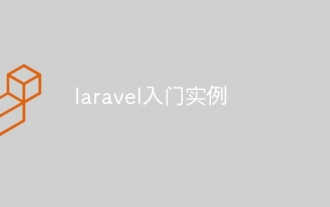
Laravel is a PHP framework for easy building of web applications. It provides a range of powerful features including: Installation: Install the Laravel CLI globally with Composer and create applications in the project directory. Routing: Define the relationship between the URL and the handler in routes/web.php. View: Create a view in resources/views to render the application's interface. Database Integration: Provides out-of-the-box integration with databases such as MySQL and uses migration to create and modify tables. Model and Controller: The model represents the database entity and the controller processes HTTP requests.
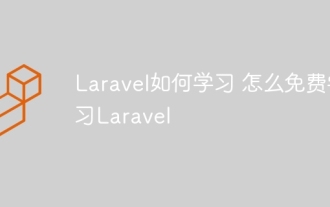
Want to learn the Laravel framework, but suffer from no resources or economic pressure? This article provides you with free learning of Laravel, teaching you how to use resources such as online platforms, documents and community forums to lay a solid foundation for your PHP development journey from getting started to master.

The Laravel development project was chosen because of its flexibility and power to suit the needs of different sizes and complexities. Laravel provides routing system, EloquentORM, Artisan command line and other functions, supporting the development of from simple blogs to complex enterprise-level systems.
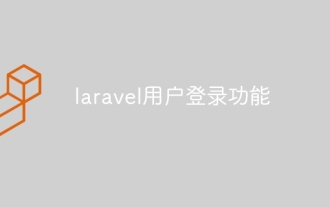
Laravel provides a comprehensive Auth framework for implementing user login functions, including: Defining user models (Eloquent model), creating login forms (Blade template engine), writing login controllers (inheriting Auth\LoginController), verifying login requests (Auth::attempt) Redirecting after login is successful (redirect) considering security factors: hash passwords, anti-CSRF protection, rate limiting and security headers. In addition, the Auth framework also provides functions such as resetting passwords, registering and verifying emails. For details, please refer to the Laravel documentation: https://laravel.com/doc
