How to set up javascript prompt box
JavaScript is a very powerful scripting language that can be used to create interactive prompt boxes in web pages. A tooltip can present information to the user, such as a warning or error message, or simply request confirmation from the user. This article explores how to use JavaScript to create different types of tooltips and explains how to customize the appearance and behavior of these tooltips.
Create a basic JavaScript tooltip
First, we will create a simple JavaScript tooltip that displays a message and an OK button. The following is the code of this prompt box:
alert("Hello, World!");
In this code, the alert() function is used to create the prompt box. It accepts a string as parameter, which is the message you want to display in the prompt box. In this example, we display a simple message "Hello, World!" to the tooltip.
You can copy this code into your HTML document and load it in the web page. When the web page loads, a tooltip will automatically pop up to show the user a simple message. The user can click the OK button on the prompt box to close the prompt box and return to the web page.
Create a prompt box with confirm and cancel buttons
You can use a JavaScript prompt box to request a confirmation action from the user. Here is an example that shows how to create a prompt box with confirm and cancel buttons:
var result = confirm("Are you sure you want to delete this file?"); if (result) { // 用户点击了确认按钮 } else { // 用户点击了取消按钮 }
In this example, we use the confirm() function to create the prompt box. It accepts a string as parameter, which is the information you need to confirm to the user. In this example, we show the user a question asking if they are sure they want to delete the file.
When the user clicks the confirm button on the prompt box, the confirm() function will return a Boolean value true. When the user clicks the cancel button, the confirm() function will return false. Based on the return value, we can perform corresponding logic in the code to respond to the user's operation.
Create a prompt box with an input box
In addition to displaying information and requesting user confirmation, JavaScript prompt boxes can also be used as input boxes. The following is an example showing how to create a prompt box with an input box:
var name = prompt("Please enter your name:", ""); if (name != null) { // 用户输入了一个名字 } else { // 用户点击了取消按钮 }
In this example, we use the prompt() function to create a prompt box. It accepts two parameters: a string used to display a message to the user asking for input information, and an optional default value. In this example, we show the user a message asking them to enter their name.
When the user clicks the OK button on the prompt box, the prompt() function will return the string entered by the user. When the user clicks the cancel button, the prompt() function returns null. Based on the return value, we can perform corresponding logic in the code to respond to the user's operation.
How to customize the appearance and behavior of tooltips
While JavaScript tooltips provide a simple and convenient way to display and request information, their appearance and behavior are limited. If you need more control, you can use JavaScript to create a custom tooltip. The following is an example that shows how to create a custom prompt box:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Custom Dialog Box</title> <style> #overlay { display: none; position: fixed; top: 0; left: 0; width: 100%; height: 100%; background-color: rgba(0,0,0,0.5); z-index: 99999; } #dialog { position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%); width: 400px; background-color: #fff; border: 1px solid #000; padding: 20px; box-shadow: 0 0 10px rgba(0,0,0,0.5); z-index: 999999; } #dialog h2 { margin-top: 0; } #dialog p { margin-bottom: 20px; } #dialog input[type="text"] { display: block; width: 100%; margin-bottom: 20px; } #dialog button { display: block; margin: 0 auto; } </style> </head> <body> <button onclick="showDialog()">Show Dialog</button> <div id="overlay"> <div id="dialog"> <h2>Custom Dialog Box</h2> <p>Please enter your name:</p> <input type="text" id="name"> <button onclick="hideDialog()">OK</button> <button onclick="cancelDialog()">Cancel</button> </div> </div> <script> function showDialog() { document.getElementById("overlay").style.display = "block"; } function hideDialog() { var name = document.getElementById("name").value; alert("Hello, " + name + "!"); document.getElementById("overlay").style.display = "none"; } function cancelDialog() { document.getElementById("overlay").style.display = "none"; } </script> </body> </html>
In this example, we create a custom dialog box that contains a title, a message, an input box and two buttons (OK and Cancel). We use CSS to define the appearance of the dialog box, and JavaScript to define the behavior of the dialog box. When the user clicks the Show Dialog button, we display this custom dialog box to the user. When the user clicks the OK button, we will get the text in the input box and display a message using the alert() function. When the user clicks the cancel button, we will hide the dialog box without performing any other actions.
This example is just the beginning of customizing the prompt box. You can change the appearance and behavior of the dialog box yourself to suit your needs. By using JavaScript and CSS, you can create very complex custom tooltips to meet your specific needs.
Summary
JavaScript provides several different ways to create prompt boxes, including the alert(), confirm(), and prompt() functions. You can use these functions to present information to the user, request a confirmation action, or obtain input. If you need more control, you can use JavaScript and CSS to customize the appearance and behavior of the tooltip. No matter which method you choose, JavaScript provides a very powerful and convenient way to create interactive tooltips.
The above is the detailed content of How to set up javascript prompt box. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
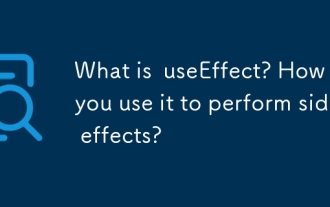
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
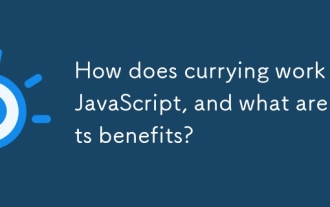
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
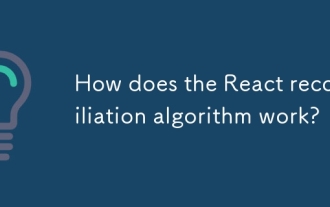
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
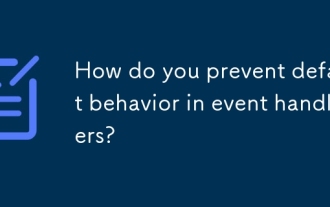
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
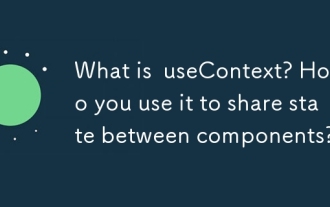
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
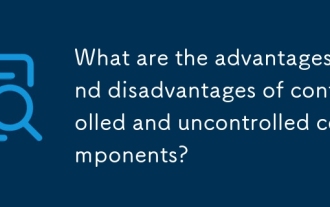
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
