Tips for using namespaces in PHP
With the development of PHP, the amount of code continues to increase, and the organizational structure of the code becomes more and more important. When using PHP, namespace is a very important concept. It can help us better organize the code, solve problems such as naming conflicts, and improve the readability and maintainability of the code. This article will introduce how to use namespaces in PHP, as well as some common usage tips.
1. The basic concept of namespace
Namespace is a way to organize related classes, interfaces, functions, etc. In PHP, the namespace is defined using the keyword "namespace" and is defined as follows:
namespace MyNamespace;
This example defines a namespace named "MyNamespace". Classes, interfaces, functions, etc. can be defined in this namespace.
For example, in order to define a class named MyClass in the MyNamespace namespace, you can use the following syntax:
namespace MyNamespace; class MyClass { // 类定义 }
2. How to use the namespace
Namespace provides It is a way to group code. Different namespaces are independent of each other, and classes or functions with the same name can be defined in different namespaces.
- General usage
For a PHP application, there are usually multiple namespaces. In order to use a class or function in a namespace, you need to use a fully qualified name (Fully Qualified Name, FQN). For example:
<?php namespace MyNamespace; class MyClass {} ?> <?php // 使用MyNamespace命名空间中的MyClass类 $obj = new MyNamespaceMyClass(); ?>
- Use of use keyword
In order to simplify the code, you can use the keyword use to refer to a class or function in a namespace. For example:
<?php // 引用MyNamespace命名空间中的MyClass类 use MyNamespaceMyClass; $obj = new MyClass(); ?>
In the above example, the use keyword defines an alias MyClass and references the MyNamespaceMyClass class as the alias MyClass, so that MyClass can be used directly to represent MyNamespaceMyClass in subsequent code.
In addition to referencing a single class, you can also use the use keyword to reference multiple classes in batches. For example:
<?php // 批量引用多个命名空间中的类 use MyNamespace1Class1; use MyNamespace2Class2; $obj1 = new Class1(); $obj2 = new Class2(); ?>
- Define sub-namespace
You can define sub-namespaces within a namespace. For example:
<?php namespace MyNamespace; class MyClass {} namespace MyNamespaceSubNamespace; class SubClass {} ?>
In the above example, MyNamespaceSubNamespace is a sub-namespace of MyNamespace, in which classes, functions, etc. that cannot be defined in the MyNamespace namespace can be defined.
- Global namespace
Code that does not define a namespace in PHP belongs to the global namespace. You can use backslash () to refer to a class or function in the global namespace. For example:
<?php // 引用全局命名空间中的strlen函数 $len = strlen("hello world"); ?>
3. Namespace skills
- Mapping of namespaces and file paths
Normally, one namespace should correspond to one File Directory. This makes it easier to manage code and conforms to the conventional code organization method. For example, if the file directory corresponding to the namespace "MyNamespace" is "src/MyNamespace", you can use the following code to reference it:
<?php use MyNamespaceMyClass; require_once "src/MyNamespace/MyClass.php"; $obj = new MyClass(); ?>
- Namespace and automatic loading
When using namespaces, you can combine automatic loading to simplify the code. Autoloading can automatically load class files based on class names without manual inclusion. For example:
<?php // 定义自动加载函数 spl_autoload_register(function($class){ require_once str_replace("\", "/", $class).'.php'; }); // 引入MyClass类 use MyNamespaceMyClass; $obj = new MyClass(); ?>
In the above example, the spl_autoload_register function is used to define an automatic loading function to load the corresponding class file according to the class name. The MyClass class can be used directly in subsequent code without manually introducing the corresponding file.
- Namespace and Composer
Composer is a PHP dependency management tool that can easily manage the dependencies of various libraries and toolkits. When using Composer, you can reference classes and functions in libraries and toolkits through namespaces. For example:
<?php require_once "vendor/autoload.php"; use GuzzleHttpClient; $client = new Client(); ?>
In the above example, Composer is used to manage the dependencies of the GuzzleHttp library, and the namespace GuzzleHttp is used to reference the Client class in the library.
Conclusion
The namespace in PHP is a very important concept, which can help us better organize the code and avoid problems such as naming conflicts. This article introduces the basic concepts and usage of namespaces, as well as common usage techniques. I hope readers can learn from this article how to use namespaces rationally and improve the readability and maintainability of code.
The above is the detailed content of Tips for using namespaces in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


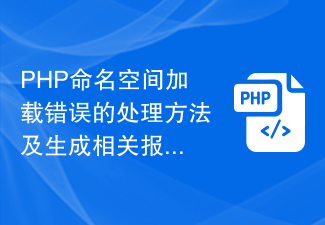
How to handle PHP namespace loading errors and generate related error prompts. In PHP development, namespace is a very important concept. It can help us organize and manage code and avoid naming conflicts. However, when using namespaces, sometimes some loading errors occur. These errors may be caused by incorrect namespace definitions or incorrect paths to loaded files. This article will introduce some common namespace loading errors, give corresponding processing methods, and how to generate relevant error prompts. 1. Namespace determination

With the development of PHP, the amount of code continues to increase, and the organizational structure of the code becomes more and more important. When using PHP, namespace is a very important concept. It can help us better organize the code, solve problems such as naming conflicts, and improve the readability and maintainability of the code. This article will introduce how to use namespaces in PHP, as well as some common usage tips. 1. Basic concepts of namespaces Namespaces are a way of organizing related classes, interfaces, functions, etc. In PHP, namespaces use the keyword "n
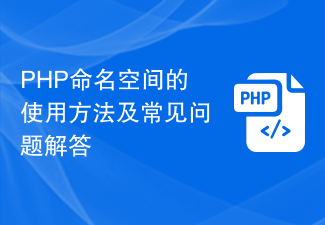
With the development of the PHP language, the concept of namespace is gradually introduced into PHP. Namespace is a method of organizing code structure to avoid naming conflicts and code confusion. In this article, we will explore how to use PHP namespaces and answer frequently asked questions. 1. Definition of namespace Namespace is a new feature introduced in PHP5.3, which allows developers to better organize their code. A namespace is an identifier (Identifier
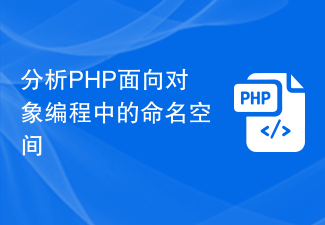
PHP is a very commonly used scripting language that is widely used in web development. As the size of the project increases, the complexity of the code also increases. In order to better manage and organize the code, PHP introduces the concept of namespace. This article will analyze the namespace in PHP object-oriented programming and give corresponding code examples. The concept of namespace A namespace is a mechanism for logically grouping code, similar to the role of a folder (directory) in a file system. It prevents naming conflicts between different classes
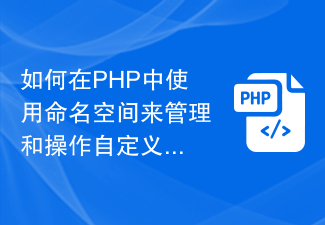
How to use namespaces in PHP to manage and manipulate custom data types In PHP, namespaces are a mechanism used to resolve naming conflicts and organize code. By using namespaces, we can classify related classes, interfaces, functions, and constants into a specific namespace to achieve better code organization and management. In this article, we will focus on how to use namespaces to manage and manipulate custom data types. We will explain the specific steps in detail through code examples. First, we need to create a named
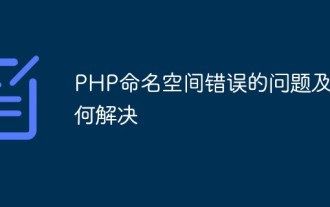
With the development of modern programming languages, namespaces have become a widely used concept. In the PHP language, namespaces have also been widely used and have become a necessary feature, which can help us avoid naming conflicts, organize code structure, etc. But this also brings about some problems, one of which is the namespace error problem. This article will discuss the PHP namespace error problem and provide solutions. 1. What is a PHP namespace? Before introducing the PHP namespace error problem, we need to first understand

How to use namespaces in PHP to manage and operate database-related data types Introduction: In PHP, database operations are an important part of development. Using namespaces to manage and operate database-related data types can improve code maintainability and readability. This article will introduce how to use namespaces in PHP to manage and operate database-related data types, and provide relevant code examples. 1. The concept of namespace Namespace is a technology used to solve naming conflict problems. By encapsulating functions, classes, interfaces, etc.
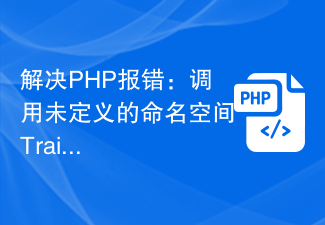
Solve PHP error: calling undefined namespace Trait. During the development process of using PHP, if the error of calling undefined namespace Trait occurs, it is usually caused by namespace-related problems. This article will introduce the cause and solution of this problem, and attach relevant code examples. Cause Analysis In PHP, it is a common practice to use namespaces to organize and manage code. The role of namespace is to avoid naming conflicts and facilitate code maintenance and expansion. When we are using Trait
