golang plugin settings
In the development of Golang, plug-ins are often used to enhance the functionality of the program. The setting of plug-ins is also something that needs to be dealt with frequently during development. This article will introduce how to set up plugins in Golang projects.
- Basic concept of plug-in
Plug-in is an independent module loaded in the main program, which can enhance the function of the main program. In Golang, a plug-in can be a package (compiled into a .so file) or an executable program (compiled into a .a file). Plug-ins can be dynamically loaded and unloaded, so that the functionality of the main program can be dynamically increased or decreased as needed.
- How to load plug-ins
In Golang, there are two ways to load plug-ins: static loading and dynamic loading.
Static loading refers to linking the plug-in code into the main program during compilation. This method is suitable for cases where the plug-in is a package. If the plug-in is an executable program, you need to use dynamic loading.
Dynamic loading refers to dynamically loading the plug-in code into the main program at runtime. This method is suitable for the case where the plug-in is an executable program. In Golang, dynamic loading can be achieved using the standard library of plug-ins.
- Plug-in setup steps
In order to set up the plug-in, we need to follow the following steps:
3.1 Step 1: Create the plug-in
We can create a package as a plug-in and then compile it into a .so file by command go build -buildmode=plugin plugin.go. Or we can create an executable program as a plug-in, and then compile it into a .so file by command go build -buildmode=shared -o plugin.so plugin.go.
3.2 Step 2: Load the plug-in
In the main program, we can use the standard library of the plug-in to load the plug-in. First, we need to use the plug-in's Open function to open the plug-in file. We can then use the Lookup function of the plugin object to find the symbol (function or variable) in the plugin. Finally, we can call functions in the plug-in or use variables in the plug-in through the obtained symbols.
The following is a sample code:
package main import ( "plugin" ) func main() { // 打开插件文件 p, err := plugin.Open("plugin.so") if err != nil { panic(err) } // 查找插件中的函数 f, err := p.Lookup("Foo") if err != nil { panic(err) } // 调用插件中的函数 f.(func())() }
In this sample code, we open a plug-in file named plugin.so and find the symbol named Foo. Finally, we call the Foo function. Note that we need to use type assertion to convert f to func() type so that we can call the Foo function.
4. Summary
Through the introduction of this article, we have learned how to set up plug-ins in Golang projects. During development, you can flexibly choose static loading or dynamic loading to use plug-ins as needed to enhance the functionality of the program. At the same time, we also introduced how to create and load plug-ins to help us better understand the concept and use of plug-ins.
The above is the detailed content of golang plugin settings. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
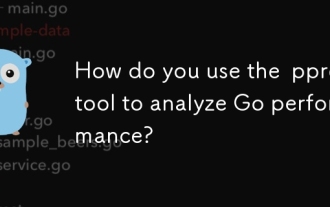
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
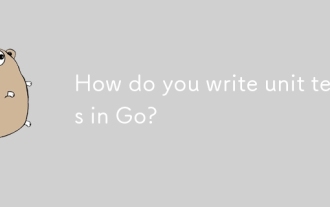
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
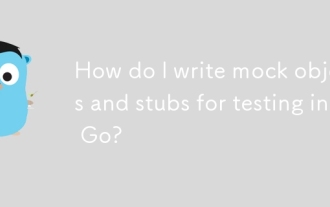
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
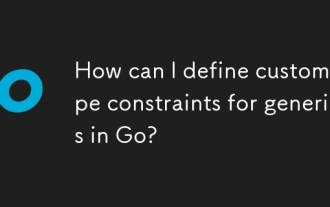
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
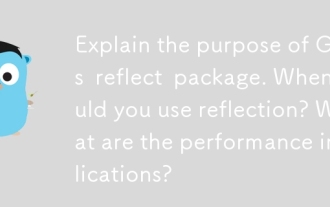
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
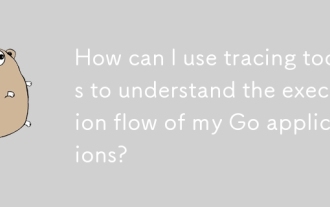
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
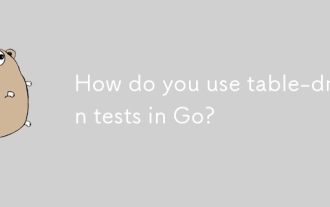
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
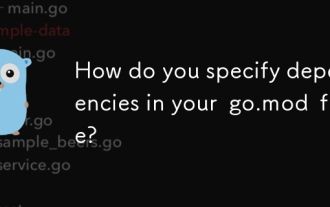
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
