ffmpeg golang transcoding
As video becomes more widely used in modern media, many applications require video transcoding between different platforms and devices. In the process, FFmpeg and Golang have become the transcoding tools of choice for many developers. This article will introduce the basic concepts and usage of FFmpeg and Golang, and how to combine them for efficient video transcoding.
FFmpeg Introduction
FFmpeg is an open source cross-platform video and audio codec library that can be used to process various video formats. It provides a command line tool that allows developers to directly use its features such as format conversion, video cutting, real-time transcoding, etc.
Golang Introduction
Golang is a modern programming language, first developed by Google and open source. It is widely regarded as an efficient, simple and secure programming language, especially suitable for use in network and cloud computing applications.
Combining FFmpeg and Golang
Golang can use CGO technology to call C language libraries, which makes it easy to use the functions in FFmpeg. By using FFmpeg's command line tool, we can easily transcode videos to different formats such as mp4, webm, etc.
However, by directly calling the FFmpeg command line tool, you need to fork a child process, and then wait for the child process to exit to obtain the results. This method is inefficient and is not conducive to program expansion and maintenance.
Therefore, Golang provides a tool called cgo to easily allow us to use C code in Golang programs, and then conveniently use the functions of FFmpeg. In the following example, we will show how to encapsulate the functions of FFmpeg through cgo technology.
First, we need to define a structure in Golang to represent the AVFrame type in FFmpeg.
type AVFrame struct { data [8]*uint8 linesize [8]int32 best_effort_timestamp int64 pkt_pts int64 }
Next, we need to define some C function interfaces to call FFmpeg functions. For example, we can define a function to open an audio or video file:
// #cgo LDFLAGS: -lavformat -lavcodec -lavutil // #include <libavformat/avformat.h> // #include <libavcodec/avcodec.h> // #include <libavutil/avutil.h> import "C" func av_open_input_file(pFormatContext **C.AVFormatContext, filename string, fmt *C.AVInputFormat, buf_size int, pFormatParams **C.AVFormatParameters) int { cfilename := C.CString(filename) defer C.free(unsafe.Pointer(cfilename)) result := C.av_open_input_file(pFormatContext, cfilename, fmt, C.int(buf_size), pFormatParams) return int(result) }
In the above code, we use the comment directive #cgo LDFLAGS to tell the Golang compiler that it needs to link the FFmpeg library file. At the same time, we also use the unsafe.Pointer type provided by CGO to pass pointer objects to C code.
Of course, in order to be able to use other functions provided by FFmpeg, other C function interfaces need to be defined. To simplify the example introduction, only a simple interface function is listed here.
Once we define these interface functions, we can easily use these interface functions in Golang code to take advantage of various functions of FFmpeg.
For example, we can use the following code to convert an audio file in WAV format to mp3 format:
func main() { var pFormatContext *C.AVFormatContext var inputFormat *C.AVInputFormat var formatParams *C.AVFormatParameters filename := "input.wav" if ret := av_open_input_file(&pFormatContext, filename, inputFormat, 0, &formatParams); ret != 0 { log.Fatalf("Could not open input file %s, error code=%d ", filename, ret) } if ret := C.avformat_find_stream_info(pFormatContext, nil); ret < 0 { log.Fatalf("Could not find stream info, error code=%d ", ret) } audioStreamIndex := -1 for i := 0; i < int(pFormatContext.nb_streams); i++ { st := (*C.AVStream)(unsafe.Pointer(uintptr(unsafe.Pointer(pFormatContext.streams)) + uintptr(i)*unsafe.Sizeof(*pFormatContext.streams))) if st.codec.codec_type == C.AVMEDIA_TYPE_AUDIO { audioStreamIndex = i break } } if audioStreamIndex == -1 { log.Fatalf("Could not find audio stream ") } audioStream := (*C.AVStream)(unsafe.Pointer(uintptr(unsafe.Pointer(pFormatContext.streams)) + uintptr(audioStreamIndex)*unsafe.Sizeof(*pFormatContext.streams))) audioCodecContext := (*C.AVCodecContext)(unsafe.Pointer(audioStream.codec)) audioCodec := C.avcodec_find_decoder(audioCodecContext.codec_id) if audioCodec == nil { log.Fatalf("Unsupported codec type, codec_id=%d ", audioCodecContext.codec_id) } if ret := C.avcodec_open2(audioCodecContext, audioCodec, nil); ret < 0 { log.Fatalf("Could not open audio codec, error code=%d ", ret) } tempFilePath := "temp.raw" tempFile, _ := os.Create(tempFilePath) defer tempFile.Close() defer os.Remove(tempFilePath) packet := (*C.AVPacket)(C.malloc(C.sizeof_AVPacket)) defer C.free(unsafe.Pointer(packet)) frame := (*C.AVFrame)(C.avcodec_alloc_frame()) defer C.av_free(unsafe.Pointer(frame)) for { if ret := C.av_read_frame(pFormatContext, packet); ret < 0 { break } if packet.stream_index == C.int(audioStreamIndex) { if ret := C.avcodec_decode_audio4(audioCodecContext, frame, (*C.int)(nil), packet); ret > 0 { numSamples := int(frame.nb_samples) dataPtr := uintptr(unsafe.Pointer(frame.data[0])) dataSlice := (*[1 << 30]byte)(unsafe.Pointer(dataPtr)) dataSize := numSamples * int(audioCodecContext.channels) * int(C.av_get_bytes_per_sample(audioCodecContext.sample_fmt)) tempFile.Write(dataSlice[:dataSize]) } } C.av_free_packet(packet) } tempFile.Close() outputFilePath := "output.mp3" cmd := exec.Command("ffmpeg", "-y", "-f", "s16le", "-ar", strconv.Itoa(int(audioCodecContext.sample_rate)), "-ac", strconv.Itoa(int(audioCodecContext.channels)), "-i", tempFilePath, "-f", "mp3", outputFilePath) stdout, _ := cmd.StdoutPipe() cmd.Start() for { buf := make([]byte, 1024) n, err := stdout.Read(buf) if err != nil || n == 0 { break } } cmd.Wait() }
In the above example, we first use the av_open_input_file function to open the audio file, and then use the avformat_find_stream_info function Get audio stream information.
Next, we traverse all streams to find the audio stream and use the avcodec_open2 function to open the audio decoder. After that, we use the av_read_frame function to read the audio data frame by frame and write the audio data to a temporary file.
Finally, we use the FFmpeg command line tool to convert the audio data in the temporary file into an audio file in mp3 format.
Conclusion
By combining Golang and FFmpeg, we can easily implement an efficient video transcoding program and use Golang's elegant syntax and built-in functions. Although using cgo technology may require some knowledge of the C language, it is not difficult to implement and the results are significant. If you need high performance and portability when developing a video transcoding program, combining Golang and FFmpeg may be a good choice.
The above is the detailed content of ffmpeg golang transcoding. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
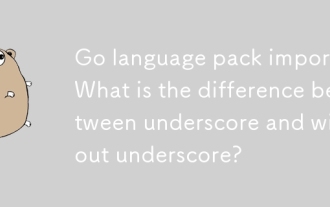
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
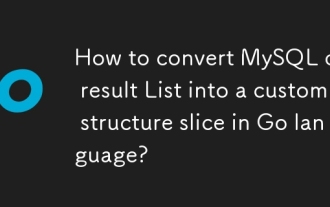
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
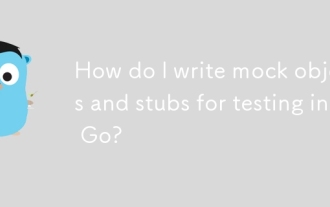
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
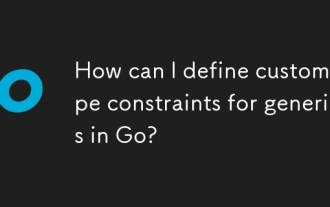
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
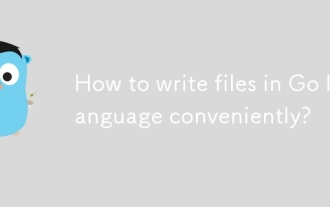
This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
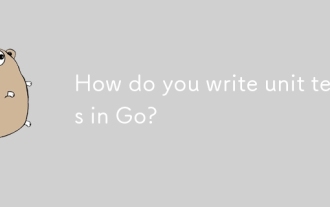
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
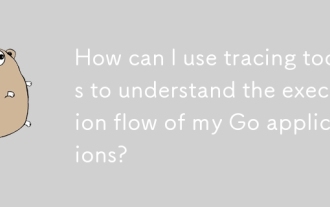
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
