


How does Python use ChatGPT to read .env to implement file configuration isolation and ensure private data security?
How Python reads the .env configuration file and implements a code encapsulation
You can use the dotenv library in Python to access the .env configuration file. The dotenv library allows you to import environment variables from .env files into Python scripts, making it easy to use them in your scripts.
The following is a basic code package for loading environment variables from the .env file:
import os from dotenv import load_dotenv def load_env(): # Load environment variables from .env file load_dotenv() # Get environment variables api_key = os.getenv("OPENAI_API_KEY") # Check if environment variables are present if not api_key: raise ValueError("Environment variables are missing.") # Return environment variables as dictionary return { "api_key": api_key }
First, this package function calls the load_dotenv() function to load the .env file. environment variables. Then, it uses the os.getenv() function to get the required environment variables. In this example we obtain an environment variable named OPENAI_API_KEY. Finally, it returns all environment variables as a dictionary. If the required environment variable is not found, a ValueError exception is raised.
To use this package, you just need to import and call it in the script that needs to use the environment variables:
from my_module import load_env env_vars = load_env() api_key = env_vars["api_key"]
This will return a dictionary containing the key values of all environment variables right. You can add or remove environment variables as needed and include them in the returned dictionary.
How does Python read the .env configuration file, obtain all items, and implement a code encapsulation
In Python, if you want to access all environment variables and read the .env configuration file, you can use dotenv library. Using this library, you can easily import environment variables from .env files into Python scripts.
The following is a basic code package for loading all environment variables from the .env file:
import os from dotenv import load_dotenv def load_env(): # Load environment variables from .env file load_dotenv() # Get all environment variables env_vars = os.environ # Return environment variables as dictionary return dict(env_vars)
All environment variables are loaded from the .env file. This package function uses The load_dotenv() function is installed. Next, it calls the os.environ variable to obtain all environment variables, and returns a dictionary containing the key-value pairs of all environment variables.
To use this package, you just need to import and call it in a script that needs to use environment variables:
from my_module import load_env env_vars = load_env() # Print all environment variables for key, value in env_vars.items(): print(f"{key}: {value}")
This will print the names and values of all environment variables. You can use environment variables as needed. Note that environment variable values are always strings. Use the corresponding data type conversion function, such as int() or float(), to convert them to the desired data type.
How does Python read the .env configuration file, get all the items, only read the items in .env, and implement a code encapsulation
Use the configparser module in Python to read. All items in the env configuration file. This module painlessly reads .env files and generates a dictionary containing all fields.
The following is a basic code wrapper for loading all items from the .env file:
import configparser def load_env(): # Load environment variables from .env file config = configparser.ConfigParser() config.read(".env") # Get all items from the .env file items = dict(config.items("default")) # Return items as a dictionary return items
All items are loaded into this wrapper function, using the configparser module from the .env file Obtain. A new configuration parser is created, using the ConfigParser() class, and loading the .env file via the read() method. It then calls the items() method to extract all the entries in the file, saving them into a dictionary.
To use this package, you just need to import and call it in a script that needs to use the items in the .env file:
from my_module import load_env env_vars = load_env() # Print all items in the .env file for key, value in env_vars.items(): print(f"{key}: {value}")
This will print all items in the .env file . Note that this method only reads items from the .env file and does not load them into environment variables. If you want to load them into environment variables, you can use the load_dotenv() function from the python-dotenv library.
The above is the detailed content of How does Python use ChatGPT to read .env to implement file configuration isolation and ensure private data security?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


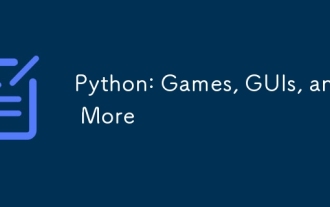
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
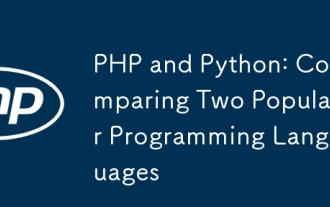
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
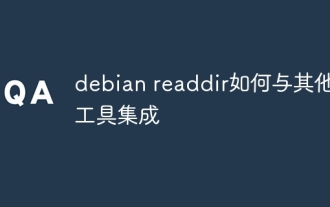
The readdir function in the Debian system is a system call used to read directory contents and is often used in C programming. This article will explain how to integrate readdir with other tools to enhance its functionality. Method 1: Combining C language program and pipeline First, write a C program to call the readdir function and output the result: #include#include#include#includeintmain(intargc,char*argv[]){DIR*dir;structdirent*entry;if(argc!=2){
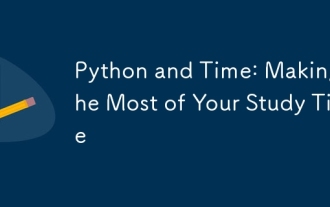
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
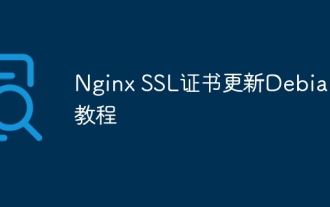
This article will guide you on how to update your NginxSSL certificate on your Debian system. Step 1: Install Certbot First, make sure your system has certbot and python3-certbot-nginx packages installed. If not installed, please execute the following command: sudoapt-getupdatesudoapt-getinstallcertbotpython3-certbot-nginx Step 2: Obtain and configure the certificate Use the certbot command to obtain the Let'sEncrypt certificate and configure Nginx: sudocertbot--nginx Follow the prompts to select
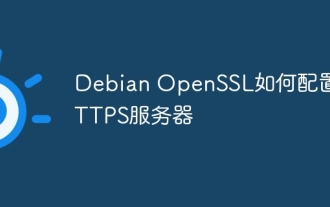
Configuring an HTTPS server on a Debian system involves several steps, including installing the necessary software, generating an SSL certificate, and configuring a web server (such as Apache or Nginx) to use an SSL certificate. Here is a basic guide, assuming you are using an ApacheWeb server. 1. Install the necessary software First, make sure your system is up to date and install Apache and OpenSSL: sudoaptupdatesudoaptupgradesudoaptinsta
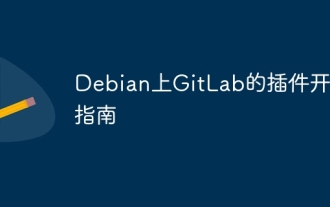
Developing a GitLab plugin on Debian requires some specific steps and knowledge. Here is a basic guide to help you get started with this process. Installing GitLab First, you need to install GitLab on your Debian system. You can refer to the official installation manual of GitLab. Get API access token Before performing API integration, you need to get GitLab's API access token first. Open the GitLab dashboard, find the "AccessTokens" option in the user settings, and generate a new access token. Will be generated
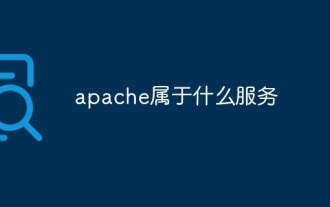
Apache is the hero behind the Internet. It is not only a web server, but also a powerful platform that supports huge traffic and provides dynamic content. It provides extremely high flexibility through a modular design, allowing for the expansion of various functions as needed. However, modularity also presents configuration and performance challenges that require careful management. Apache is suitable for server scenarios that require highly customizable and meet complex needs.
