api forwarding golang
With the popularity of modern software, developers often need to use application programming interfaces (APIs) to interact with different systems. Since APIs are often created by organizations or enterprises, when communicating between different systems, developers need to provide different code and protocol adapters to handle these interfaces, which often results in complex code and difficult maintenance. To simplify this process, developers can use golang to develop an API forwarder to make API communication between different systems simpler and easier to use.
API forwarding refers to forwarding requests from one system to another system. It is often used to integrate different applications and systems to enable data transfer and sharing functions. API forwarding can be used at different levels of the system, for example: from a front-end JavaScript application to the back-end you may need to communicate between multiple APIs. Therefore, the design of an API forwarder is very important.
Golang is a fast programming language with low system resource overhead, so it is very suitable for developing efficient API forwarders. In this article, we will introduce how to implement a simple API forwarder using golang.
First, we need to prepare the tools and environment required to build an API forwarder. We need a Go development environment to write and run code. Use the golang package manager to manage the dependencies required for your project. In order to build the API we also need to use an HTTP framework. One of the most popular HTTP frameworks in golang is the Gin framework, which is borrowed from Flask.
In the next steps, we will use the following steps to create a simple API forwarder:
- Create the main.go file and import the required packages and dependencies .
package main import ( "fmt" "net/http" )
- Create a function called handler that will handle all incoming requests and forward it.
func handler(w http.ResponseWriter, r *http.Request) { fmt.Println("Incoming request") // TODO: Write code to forward the request }``` 3. 我们需要一个接收请求并将其转发到指定位置的函数。在本例中,我们将使用 net/http 包中的 ReverseProxy 函数来执行此操作。
func forwardRequest(w http.ResponseWriter, r *http.Request) {
// TODO: Write code to forward the request
proxy := &httputil.ReverseProxy{Director: func(req *http.Request) {
req.URL.Scheme = "http" req.URL.Host = "localhost:5000" req.URL.Path = "/api/v1" + req.URL.Path
}}
proxy.ServeHTTP(w, r)
}
在上面的代码中,我们使用 ReverseProxy 函数将请求转发到指定的主机和端口。在这个例子中,我们将请求转发到 Python Flask 应用程序的本地主机和端口。我们还指定了一些请求路径,以便更好地定位转发到哪个特定的 API。 4. 在 handler 函数中,我们将检查每个传入的请求,然后将其转发到指定位置。
func handler(w http.ResponseWriter , r *http.Request) {
fmt.Println("Incoming request")
// Forward the request
forwardRequest(w, r)
}
在上面的代码中,我们将从 forwardRequest 函数中调用转发请求的代码。现在,我们已经准备好将请求从 golang 转发到 Flask 应用程序。 5. 最后,我们需要为我们的 API 转发器创建一个 HTTP 服务器。在启动 HTTP 服务器之前,我们需要设置服务器的端口和处理器函数。
func main () {
http.HandleFunc("/", handler)
fmt.Println("Starting server on :8080...")
http.ListenAndServe(":8080", nil)
}
在上面的代码中,我们设置了服务器的端口为 8080,并将所有请求传递给 handler 函数进行处理。 6. 最后,我们可以使用 go run 命令来启动我们的 API 转发器。
go run main.go
通过此过程,我们已经实现了一个可用于转发请求的简单 API 转发器。这个API转发简单,但也非常灵活。通过使用 Golang 开发 API 转发器,开发人员可以更快速构建更强大的 API。
The above is the detailed content of api forwarding golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
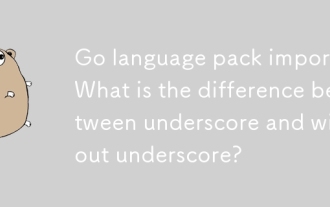
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
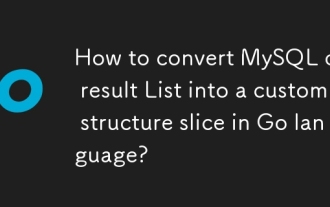
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
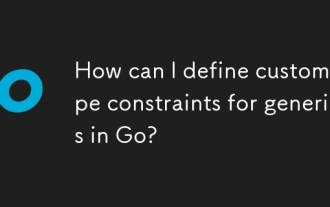
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
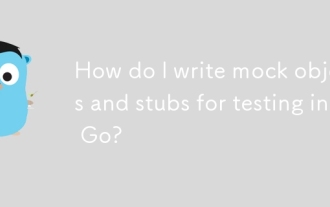
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
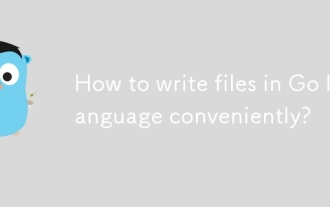
This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
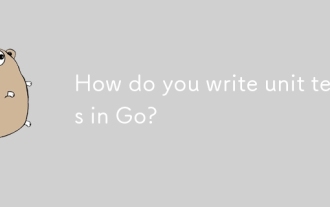
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
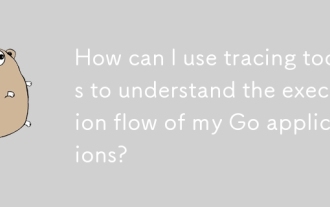
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
