How to prevent nodejs from closing
With the rapid development of web development, Node.js has become a very popular server-side development language. It uses an event-driven, non-blocking I/O model and can easily handle large numbers of client requests. However, due to its design features and usage, sometimes Node.js programs are shut down. This article will explain how to prevent Node.js from closing to ensure the stability and reliability of the program.
- Monitoring Process
The most common unexpected shutdown situation of Node.js program is process crash. Therefore, you need to monitor how the process is running. Node.js provides the process object to help developers monitor the status of the process. You can use the following code to start a periodic function that periodically checks whether the process is running.
setInterval(function() { console.log("Process is running"); }, 5000);
The above code will output the "Process is running" message every 5 seconds. If you don't see output for a while, your Node.js process may have crashed.
- Handling Uncaught Exceptions
Another situation that causes a Node.js program to shut down unexpectedly is an uncaught exception. This situation usually occurs when a program attempts to access a variable that does not exist or handles an exception incorrectly. Node.js provides the uncaughtException event. When an uncaught exception occurs, the program will automatically trigger this event. You can listen to this event to handle exception information and avoid unexpected program shutdown.
The following is an example:
process.on('uncaughtException', function (err) { console.log('Caught exception: ' + err); });
In the above code, we listen to the uncaughtException event and print the exception information in the event. In actual development, you can handle exception information reasonably according to different situations to avoid program crashes.
- Use process management tools
If your Node.js program closes unexpectedly, you need to restart it manually. This is inconvenient and may make your server system unstable. Therefore, we recommend using process management tools, such as PM2, Forever, etc. These tools can automatically manage the startup, restart, logs, etc. of the Node.js process for you. When a Node.js program closes unexpectedly, these tools automatically restart the program to ensure it can continue to work.
The following is an example of using PM2 to start a Node.js program:
pm2 start app.js
In the above code, we use PM2 to start a Node.js program named app.js. PM2 will automatically assign a process ID to the program, and then you can use pm2 stop, pm2 restart and other commands to manage the process.
- Optimize memory management
Another reason that causes the Node.js program to close is memory overflow. Since Node.js is single-threaded, memory needs to be managed with extra care. In Node.js, once the program runs out of available memory, the program crashes. Therefore, you need to optimize memory management to prevent this from happening.
Here are some quick memory management tips:
-Avoid frequent creation and deletion of objects. Reduce memory usage by reusing objects.
- Use streams and buffers instead of strings. Since strings are immutable in Node.js, frequent use of strings can lead to memory leaks.
-Use the garbage collector. Node.js provides a garbage collector that automatically detects and clears objects that are no longer needed, thereby reducing memory usage.
-Limit concurrent requests. By limiting the number of concurrent requests, you can prevent your program from running for long periods of time and consuming too much memory.
Summary
Node.js is a very powerful development tool, but it may also shut down unexpectedly, affecting development progress and server stability. This article introduces how to prevent Node.js from shutting down, including techniques such as monitoring processes, handling uncaught exceptions, using process management tools, and optimizing memory management. We encourage developers to pay attention to the stability and reliability of the program when developing Node.js programs to ensure that the program can run stably for a long time.
The above is the detailed content of How to prevent nodejs from closing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
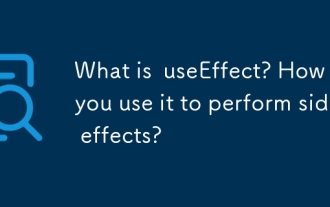
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
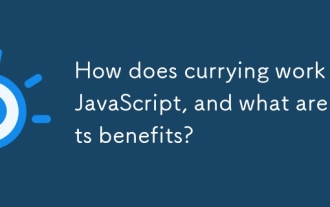
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
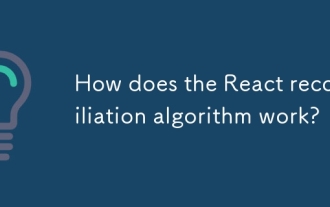
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
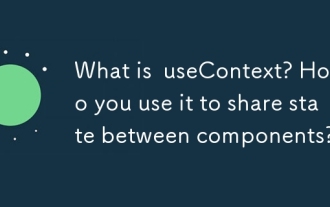
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
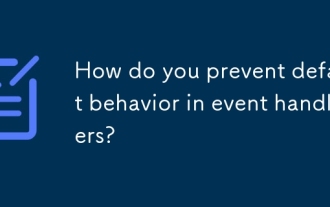
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
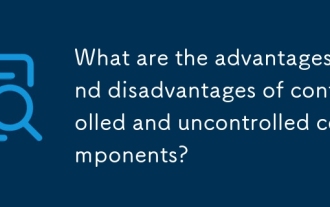
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
